mirror of
https://github.com/GNOME/libxml2.git
synced 2025-05-10 13:41:38 +08:00
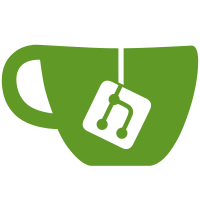
Commit 62150ed2 introduced a small regression in the error messages for mismatched tags. This typically only affected messages after the first mismatch, but with custom SAX handlers all line numbers would be off. This also fixes line numbers in the SAX push parser which were never handled correctly.
57 lines
1.3 KiB
Python
Executable File
57 lines
1.3 KiB
Python
Executable File
#!/usr/bin/python -u
|
|
#
|
|
# This test exercise the redirection of error messages with a
|
|
# functions defined in Python.
|
|
#
|
|
import sys
|
|
import libxml2
|
|
|
|
# Memory debug specific
|
|
libxml2.debugMemory(1)
|
|
|
|
expect="""--> (3) xmlns: URI foo is not absolute
|
|
--> (4) Opening and ending tag mismatch: x line 1 and y
|
|
"""
|
|
|
|
err=""
|
|
def callback(arg,msg,severity,reserved):
|
|
global err
|
|
err = err + "%s (%d) %s" % (arg,severity,msg)
|
|
|
|
s = """<x xmlns="foo"></y>"""
|
|
|
|
parserCtxt = libxml2.createPushParser(None,"",0,"test.xml")
|
|
parserCtxt.setErrorHandler(callback, "-->")
|
|
if parserCtxt.getErrorHandler() != (callback,"-->"):
|
|
print("getErrorHandler failed")
|
|
sys.exit(1)
|
|
parserCtxt.parseChunk(s,len(s),1)
|
|
doc = parserCtxt.doc()
|
|
doc.freeDoc()
|
|
parserCtxt = None
|
|
|
|
if err != expect:
|
|
print("error")
|
|
print("received %s" %(err))
|
|
print("expected %s" %(expect))
|
|
sys.exit(1)
|
|
|
|
i = 10000
|
|
while i > 0:
|
|
parserCtxt = libxml2.createPushParser(None,"",0,"test.xml")
|
|
parserCtxt.setErrorHandler(callback, "-->")
|
|
parserCtxt.parseChunk(s,len(s),1)
|
|
doc = parserCtxt.doc()
|
|
doc.freeDoc()
|
|
parserCtxt = None
|
|
err = ""
|
|
i = i - 1
|
|
|
|
# Memory debug specific
|
|
libxml2.cleanupParser()
|
|
if libxml2.debugMemory(1) == 0:
|
|
print("OK")
|
|
else:
|
|
print("Memory leak %d bytes" % (libxml2.debugMemory(1)))
|
|
libxml2.dumpMemory()
|