mirror of
https://github.com/GNOME/libxml2.git
synced 2025-05-07 20:39:30 +08:00
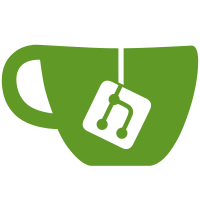
This adds Python code to look up the required feature macros for a symbol in tools/xmlmod.py.
1409 lines
55 KiB
C
1409 lines
55 KiB
C
/*
|
|
* testapi.c: libxml2 API tester program.
|
|
*
|
|
* Automatically generated by gentest.py
|
|
*
|
|
* See Copyright for the status of this software.
|
|
*/
|
|
|
|
/* Disable deprecation warnings */
|
|
#define XML_DEPRECATED
|
|
|
|
#include "libxml.h"
|
|
#include <libxml/HTMLparser.h>
|
|
#include <libxml/HTMLtree.h>
|
|
#include <libxml/c14n.h>
|
|
#include <libxml/catalog.h>
|
|
#include <libxml/debugXML.h>
|
|
#include <libxml/parserInternals.h>
|
|
#include <libxml/pattern.h>
|
|
#include <libxml/relaxng.h>
|
|
#include <libxml/schematron.h>
|
|
#include <libxml/uri.h>
|
|
#include <libxml/xinclude.h>
|
|
#include <libxml/xlink.h>
|
|
#include <libxml/xmlmodule.h>
|
|
#include <libxml/xmlreader.h>
|
|
#include <libxml/xmlsave.h>
|
|
#include <libxml/xmlschemas.h>
|
|
#include <libxml/xmlschemastypes.h>
|
|
#include <libxml/xmlwriter.h>
|
|
#include <libxml/xpathInternals.h>
|
|
#include <libxml/xpointer.h>
|
|
|
|
static void
|
|
ignoreError(void *userData ATTRIBUTE_UNUSED,
|
|
const xmlError *error ATTRIBUTE_UNUSED) {
|
|
}
|
|
|
|
int
|
|
main(int argc ATTRIBUTE_UNUSED, char **argv ATTRIBUTE_UNUSED) {
|
|
xmlInitParser();
|
|
xmlSetStructuredErrorFunc(NULL, ignoreError);
|
|
|
|
xmlFreeParserInputBuffer(__xmlParserInputBufferCreateFilename(NULL, 0));
|
|
xmlAddAttributeDecl(NULL, NULL, NULL, NULL, NULL, 0, 0, NULL, NULL);
|
|
xmlFreeNode(xmlAddChild(NULL, NULL));
|
|
xmlFreeNode(xmlAddChildList(NULL, NULL));
|
|
xmlAddDocEntity(NULL, NULL, 0, NULL, NULL, NULL);
|
|
xmlAddDtdEntity(NULL, NULL, 0, NULL, NULL, NULL);
|
|
xmlAddElementDecl(NULL, NULL, NULL, 0, NULL);
|
|
xmlAddEncodingAlias(NULL, NULL);
|
|
xmlAddEntity(NULL, 0, NULL, 0, NULL, NULL, NULL, NULL);
|
|
xmlAddID(NULL, NULL, NULL, NULL);
|
|
xmlAddIDSafe(NULL, NULL);
|
|
xmlFreeNode(xmlAddNextSibling(NULL, NULL));
|
|
xmlAddNotationDecl(NULL, NULL, NULL, NULL, NULL);
|
|
xmlFreeNode(xmlAddPrevSibling(NULL, NULL));
|
|
xmlAddRef(NULL, NULL, NULL, NULL);
|
|
xmlFreeNode(xmlAddSibling(NULL, NULL));
|
|
xmlFreeParserInputBuffer(xmlAllocParserInputBuffer(0));
|
|
xmlFree(xmlBufContent(NULL));
|
|
xmlFree(xmlBufEnd(NULL));
|
|
xmlBufGetNodeContent(NULL, NULL);
|
|
xmlBufShrink(NULL, 0);
|
|
xmlBufUse(NULL);
|
|
xmlBufferAdd(NULL, NULL, 0);
|
|
xmlBufferAddHead(NULL, NULL, 0);
|
|
xmlBufferCCat(NULL, NULL);
|
|
xmlBufferCat(NULL, NULL);
|
|
xmlBufferContent(NULL);
|
|
xmlBufferFree(xmlBufferCreate());
|
|
xmlBufferFree(xmlBufferCreateSize(0));
|
|
xmlBufferFree(xmlBufferCreateStatic(NULL, 0));
|
|
xmlFree(xmlBufferDetach(NULL));
|
|
xmlBufferDump(NULL, NULL);
|
|
xmlBufferEmpty(NULL);
|
|
xmlBufferFree(NULL);
|
|
xmlBufferGrow(NULL, 0);
|
|
xmlBufferLength(NULL);
|
|
xmlBufferResize(NULL, 0);
|
|
xmlBufferSetAllocationScheme(NULL, 0);
|
|
xmlBufferShrink(NULL, 0);
|
|
xmlBufferWriteCHAR(NULL, NULL);
|
|
xmlBufferWriteChar(NULL, NULL);
|
|
xmlBufferWriteQuotedString(NULL, NULL);
|
|
xmlFree(xmlBuildQName(NULL, NULL, NULL, 0));
|
|
xmlFree(xmlBuildRelativeURI(NULL, NULL));
|
|
xmlBuildRelativeURISafe(NULL, NULL, NULL);
|
|
xmlFree(xmlBuildURI(NULL, NULL));
|
|
xmlBuildURISafe(NULL, NULL, NULL);
|
|
xmlByteConsumed(NULL);
|
|
xmlFree(xmlCanonicPath(NULL));
|
|
xmlCharEncCloseFunc(NULL);
|
|
xmlCharEncFirstLine(NULL, NULL, NULL);
|
|
xmlCharEncInFunc(NULL, NULL, NULL);
|
|
xmlCharEncNewCustomHandler(NULL, 0, 0, 0, NULL, NULL, NULL);
|
|
xmlCharEncOutFunc(NULL, NULL, NULL);
|
|
xmlCharInRange(0, NULL);
|
|
xmlFree(xmlCharStrdup(NULL));
|
|
xmlFree(xmlCharStrndup(NULL, 0));
|
|
xmlCheckFilename(NULL);
|
|
xmlFreeInputStream(xmlCheckHTTPInput(NULL, NULL));
|
|
xmlCheckLanguageID(NULL);
|
|
xmlCheckThreadLocalStorage();
|
|
xmlCheckUTF8(NULL);
|
|
xmlChildElementCount(NULL);
|
|
xmlCleanupCharEncodingHandlers();
|
|
xmlCleanupEncodingAliases();
|
|
xmlCleanupGlobals();
|
|
xmlCleanupInputCallbacks();
|
|
xmlCleanupMemory();
|
|
xmlCleanupThreads();
|
|
xmlClearNodeInfoSeq(NULL);
|
|
xmlClearParserCtxt(NULL);
|
|
xmlCopyAttributeTable(NULL);
|
|
xmlCopyChar(0, NULL, 0);
|
|
xmlCopyCharMultiByte(NULL, 0);
|
|
xmlFreeDoc(xmlCopyDoc(NULL, 0));
|
|
xmlCopyDocElementContent(NULL, NULL);
|
|
xmlFreeDtd(xmlCopyDtd(NULL));
|
|
xmlCopyElementContent(NULL);
|
|
xmlCopyElementTable(NULL);
|
|
xmlFreeEntitiesTable(xmlCopyEntitiesTable(NULL));
|
|
xmlFreeEnumeration(xmlCopyEnumeration(NULL));
|
|
xmlCopyError(NULL, NULL);
|
|
xmlFreeNs(xmlCopyNamespace(NULL));
|
|
xmlFreeNs(xmlCopyNamespaceList(NULL));
|
|
xmlFreeNode(xmlCopyNode(NULL, 0));
|
|
xmlFreeNode(xmlCopyNodeList(NULL));
|
|
xmlCopyNotationTable(NULL);
|
|
xmlCopyProp(NULL, NULL);
|
|
xmlCopyPropList(NULL, NULL);
|
|
xmlCreateCharEncodingHandler(NULL, 0, 0, NULL, NULL);
|
|
xmlFreeParserCtxt(xmlCreateDocParserCtxt(NULL));
|
|
xmlFreeEntitiesTable(xmlCreateEntitiesTable());
|
|
xmlFreeParserCtxt(xmlCreateEntityParserCtxt(NULL, NULL, NULL));
|
|
xmlFreeEnumeration(xmlCreateEnumeration(NULL));
|
|
xmlFreeParserCtxt(xmlCreateFileParserCtxt(NULL));
|
|
xmlFreeParserCtxt(xmlCreateIOParserCtxt(NULL, NULL, 0, 0, NULL, 0));
|
|
xmlFreeDtd(xmlCreateIntSubset(NULL, NULL, NULL, NULL));
|
|
xmlFreeParserCtxt(xmlCreateMemoryParserCtxt(NULL, 0));
|
|
xmlFreeURI(xmlCreateURI());
|
|
xmlFreeParserCtxt(xmlCreateURLParserCtxt(NULL, 0));
|
|
xmlCtxtErrMemory(NULL);
|
|
xmlCtxtGetCatalogs(NULL);
|
|
xmlCtxtGetDeclaredEncoding(NULL);
|
|
xmlDictFree(xmlCtxtGetDict(NULL));
|
|
xmlFreeDoc(xmlCtxtGetDocument(NULL));
|
|
xmlCtxtGetLastError(NULL);
|
|
xmlCtxtGetOptions(NULL);
|
|
xmlCtxtGetPrivate(NULL);
|
|
xmlCtxtGetSaxHandler(NULL);
|
|
xmlCtxtGetStandalone(NULL);
|
|
xmlCtxtGetStatus(NULL);
|
|
xmlCtxtGetVersion(NULL);
|
|
xmlCtxtIsHtml(NULL);
|
|
xmlCtxtIsStopped(NULL);
|
|
xmlFreeNode(xmlCtxtParseContent(NULL, NULL, NULL, 0));
|
|
xmlFreeDoc(xmlCtxtParseDocument(NULL, NULL));
|
|
xmlFreeInputStream(xmlCtxtPopInput(NULL));
|
|
xmlCtxtPushInput(NULL, NULL);
|
|
xmlFreeDoc(xmlCtxtReadDoc(NULL, NULL, NULL, NULL, 0));
|
|
xmlFreeDoc(xmlCtxtReadFd(NULL, 0, NULL, NULL, 0));
|
|
xmlFreeDoc(xmlCtxtReadFile(NULL, NULL, NULL, 0));
|
|
xmlFreeDoc(xmlCtxtReadIO(NULL, 0, 0, NULL, NULL, NULL, 0));
|
|
xmlFreeDoc(xmlCtxtReadMemory(NULL, NULL, 0, NULL, NULL, 0));
|
|
xmlCtxtReset(NULL);
|
|
xmlCtxtResetLastError(NULL);
|
|
xmlCtxtResetPush(NULL, NULL, 0, NULL, NULL);
|
|
xmlCtxtSetCatalogs(NULL, NULL);
|
|
xmlCtxtSetCharEncConvImpl(NULL, 0, NULL);
|
|
xmlCtxtSetDict(NULL, NULL);
|
|
xmlCtxtSetErrorHandler(NULL, 0, NULL);
|
|
xmlCtxtSetMaxAmplification(NULL, 0);
|
|
xmlCtxtSetOptions(NULL, 0);
|
|
xmlCtxtSetPrivate(NULL, NULL);
|
|
xmlCtxtSetResourceLoader(NULL, 0, NULL);
|
|
xmlCtxtSetSaxHandler(NULL, NULL);
|
|
xmlCtxtUseOptions(NULL, 0);
|
|
xmlCurrentChar(NULL, NULL);
|
|
xmlDOMWrapAdoptNode(NULL, NULL, NULL, NULL, NULL, 0);
|
|
xmlDOMWrapCloneNode(NULL, NULL, NULL, NULL, NULL, NULL, 0, 0);
|
|
xmlDOMWrapFreeCtxt(NULL);
|
|
xmlDOMWrapFreeCtxt(xmlDOMWrapNewCtxt());
|
|
xmlDOMWrapReconcileNamespaces(NULL, NULL, 0);
|
|
xmlDOMWrapRemoveNode(NULL, NULL, NULL, 0);
|
|
xmlDefaultSAXHandlerInit();
|
|
xmlDelEncodingAlias(NULL);
|
|
xmlDeregisterNodeDefault(0);
|
|
xmlDetectCharEncoding(NULL, 0);
|
|
xmlDictCleanup();
|
|
xmlDictFree(xmlDictCreate());
|
|
xmlDictFree(xmlDictCreateSub(NULL));
|
|
xmlDictExists(NULL, NULL, 0);
|
|
xmlDictFree(NULL);
|
|
xmlDictGetUsage(NULL);
|
|
xmlDictLookup(NULL, NULL, 0);
|
|
xmlDictOwns(NULL, NULL);
|
|
xmlDictQLookup(NULL, NULL, NULL);
|
|
xmlDictReference(NULL);
|
|
xmlDictSetLimit(NULL, 0);
|
|
xmlDictSize(NULL);
|
|
xmlFreeNode(xmlDocCopyNode(NULL, NULL, 0));
|
|
xmlFreeNode(xmlDocCopyNodeList(NULL, NULL));
|
|
xmlFreeNode(xmlDocGetRootElement(NULL));
|
|
xmlFreeNode(xmlDocSetRootElement(NULL, NULL));
|
|
xmlFree(xmlEncodeEntitiesReentrant(NULL, NULL));
|
|
xmlFree(xmlEncodeSpecialChars(NULL, NULL));
|
|
xmlFileClose(NULL);
|
|
xmlFileMatch(NULL);
|
|
xmlFileOpen(NULL);
|
|
xmlFileRead(NULL, NULL, 0);
|
|
xmlFindCharEncodingHandler(NULL);
|
|
xmlFreeNode(xmlFirstElementChild(NULL));
|
|
xmlFormatError(NULL, 0, NULL);
|
|
xmlFreeAttributeTable(NULL);
|
|
xmlFreeDoc(NULL);
|
|
xmlFreeDocElementContent(NULL, NULL);
|
|
xmlFreeDtd(NULL);
|
|
xmlFreeElementContent(NULL);
|
|
xmlFreeElementTable(NULL);
|
|
xmlFreeEntitiesTable(NULL);
|
|
xmlFreeEntity(NULL);
|
|
xmlFreeEnumeration(NULL);
|
|
xmlFreeIDTable(NULL);
|
|
xmlFreeInputStream(NULL);
|
|
xmlFreeMutex(NULL);
|
|
xmlFreeNode(NULL);
|
|
xmlFreeNodeList(NULL);
|
|
xmlFreeNotationTable(NULL);
|
|
xmlFreeNs(NULL);
|
|
xmlFreeNsList(NULL);
|
|
xmlFreeParserCtxt(NULL);
|
|
xmlFreeParserInputBuffer(NULL);
|
|
xmlFreeProp(NULL);
|
|
xmlFreePropList(NULL);
|
|
xmlFreeRMutex(NULL);
|
|
xmlFreeRefTable(NULL);
|
|
xmlFreeURI(NULL);
|
|
xmlGcMemGet(NULL, NULL, NULL, NULL, NULL);
|
|
xmlGcMemSetup(0, 0, 0, 0, 0);
|
|
xmlGetBufferAllocationScheme();
|
|
xmlGetCharEncodingHandler(0);
|
|
xmlGetCharEncodingName(0);
|
|
xmlGetCompressMode();
|
|
xmlGetDocCompressMode(NULL);
|
|
xmlGetDocEntity(NULL, NULL);
|
|
xmlGetDtdAttrDesc(NULL, NULL, NULL);
|
|
xmlGetDtdElementDesc(NULL, NULL);
|
|
xmlGetDtdEntity(NULL, NULL);
|
|
xmlGetDtdNotationDesc(NULL, NULL);
|
|
xmlGetDtdQAttrDesc(NULL, NULL, NULL, NULL);
|
|
xmlGetDtdQElementDesc(NULL, NULL, NULL);
|
|
xmlGetEncodingAlias(NULL);
|
|
xmlGetExternalEntityLoader();
|
|
xmlGetID(NULL, NULL);
|
|
xmlFreeDtd(xmlGetIntSubset(NULL));
|
|
xmlFreeNode(xmlGetLastChild(NULL));
|
|
xmlGetLastError();
|
|
xmlGetLineNo(NULL);
|
|
xmlFree(xmlGetNoNsProp(NULL, NULL));
|
|
xmlFree(xmlGetNodePath(NULL));
|
|
xmlGetNsList(NULL, NULL);
|
|
xmlGetNsListSafe(NULL, NULL, NULL);
|
|
xmlFree(xmlGetNsProp(NULL, NULL, NULL));
|
|
xmlGetParameterEntity(NULL, NULL);
|
|
xmlGetPredefinedEntity(NULL);
|
|
xmlFree(xmlGetProp(NULL, NULL));
|
|
xmlListDelete(xmlGetRefs(NULL, NULL));
|
|
xmlGetUTF8Char(NULL, NULL);
|
|
xmlHasFeature(0);
|
|
xmlHasNsProp(NULL, NULL, NULL);
|
|
xmlHasProp(NULL, NULL);
|
|
xmlHashAdd(NULL, NULL, NULL);
|
|
xmlHashAdd2(NULL, NULL, NULL, NULL);
|
|
xmlHashAdd3(NULL, NULL, NULL, NULL, NULL);
|
|
xmlHashAddEntry(NULL, NULL, NULL);
|
|
xmlHashAddEntry2(NULL, NULL, NULL, NULL);
|
|
xmlHashAddEntry3(NULL, NULL, NULL, NULL, NULL);
|
|
xmlHashFree(xmlHashCopy(NULL, 0), NULL);
|
|
xmlHashFree(xmlHashCopySafe(NULL, 0, 0), NULL);
|
|
xmlHashFree(xmlHashCreate(0), NULL);
|
|
xmlHashFree(xmlHashCreateDict(0, NULL), NULL);
|
|
xmlHashDefaultDeallocator(NULL, NULL);
|
|
xmlHashFree(NULL, 0);
|
|
xmlHashLookup(NULL, NULL);
|
|
xmlHashLookup2(NULL, NULL, NULL);
|
|
xmlHashLookup3(NULL, NULL, NULL, NULL);
|
|
xmlHashQLookup(NULL, NULL, NULL);
|
|
xmlHashQLookup2(NULL, NULL, NULL, NULL, NULL);
|
|
xmlHashQLookup3(NULL, NULL, NULL, NULL, NULL, NULL, NULL);
|
|
xmlHashRemoveEntry(NULL, NULL, 0);
|
|
xmlHashRemoveEntry2(NULL, NULL, NULL, 0);
|
|
xmlHashRemoveEntry3(NULL, NULL, NULL, NULL, 0);
|
|
xmlHashScan(NULL, 0, NULL);
|
|
xmlHashScan3(NULL, NULL, NULL, NULL, 0, NULL);
|
|
xmlHashScanFull(NULL, 0, NULL);
|
|
xmlHashScanFull3(NULL, NULL, NULL, NULL, 0, NULL);
|
|
xmlHashSize(NULL);
|
|
xmlHashUpdateEntry(NULL, NULL, NULL, 0);
|
|
xmlHashUpdateEntry2(NULL, NULL, NULL, NULL, 0);
|
|
xmlHashUpdateEntry3(NULL, NULL, NULL, NULL, NULL, 0);
|
|
xmlInitCharEncodingHandlers();
|
|
xmlInitGlobals();
|
|
xmlInitMemory();
|
|
xmlInitNodeInfoSeq(NULL);
|
|
xmlInitParserCtxt(NULL);
|
|
xmlInitThreads();
|
|
xmlInitializeDict();
|
|
xmlInputSetEncodingHandler(NULL, NULL);
|
|
xmlIsBaseChar(0);
|
|
xmlIsBlank(0);
|
|
xmlIsBlankNode(NULL);
|
|
xmlIsChar(0);
|
|
xmlIsCombining(0);
|
|
xmlIsDigit(0);
|
|
xmlIsExtender(0);
|
|
xmlIsID(NULL, NULL, NULL);
|
|
xmlIsIdeographic(0);
|
|
xmlIsLetter(0);
|
|
xmlIsMixedElement(NULL, NULL);
|
|
xmlIsPubidChar(0);
|
|
xmlIsRef(NULL, NULL, NULL);
|
|
xmlIsXHTML(NULL, NULL);
|
|
xmlIsolat1ToUTF8(NULL, NULL, NULL, NULL);
|
|
xmlKeepBlanksDefault(0);
|
|
xmlFreeNode(xmlLastElementChild(NULL));
|
|
xmlLineNumbersDefault(0);
|
|
xmlLinkGetData(NULL);
|
|
xmlListAppend(NULL, NULL);
|
|
xmlListClear(NULL);
|
|
xmlListCopy(NULL, NULL);
|
|
xmlListDelete(xmlListCreate(0, 0));
|
|
xmlListDelete(NULL);
|
|
xmlListDelete(xmlListDup(NULL));
|
|
xmlListEmpty(NULL);
|
|
xmlListEnd(NULL);
|
|
xmlListFront(NULL);
|
|
xmlListInsert(NULL, NULL);
|
|
xmlListMerge(NULL, NULL);
|
|
xmlListPopBack(NULL);
|
|
xmlListPopFront(NULL);
|
|
xmlListPushBack(NULL, NULL);
|
|
xmlListPushFront(NULL, NULL);
|
|
xmlListRemoveAll(NULL, NULL);
|
|
xmlListRemoveFirst(NULL, NULL);
|
|
xmlListRemoveLast(NULL, NULL);
|
|
xmlListReverse(NULL);
|
|
xmlListReverseSearch(NULL, NULL);
|
|
xmlListReverseWalk(NULL, 0, NULL);
|
|
xmlListSearch(NULL, NULL);
|
|
xmlListSize(NULL);
|
|
xmlListSort(NULL);
|
|
xmlListWalk(NULL, 0, NULL);
|
|
xmlFreeInputStream(xmlLoadExternalEntity(NULL, NULL, NULL));
|
|
xmlLockLibrary();
|
|
xmlLookupCharEncodingHandler(0, NULL);
|
|
xmlMemBlocks();
|
|
xmlMemDisplay(NULL);
|
|
xmlMemDisplayLast(NULL, 0);
|
|
xmlMemFree(NULL);
|
|
xmlMemGet(NULL, NULL, NULL, NULL);
|
|
xmlMemSetup(0, 0, 0, 0);
|
|
xmlMemShow(NULL, 0);
|
|
xmlMemSize(NULL);
|
|
xmlMemUsed();
|
|
xmlMemoryDump();
|
|
xmlMutexLock(NULL);
|
|
xmlMutexUnlock(NULL);
|
|
xmlFreeNode(xmlNewCDataBlock(NULL, NULL, 0));
|
|
xmlNewCharEncodingHandler(NULL, 0, 0);
|
|
xmlFreeNode(xmlNewCharRef(NULL, NULL));
|
|
xmlFreeNode(xmlNewChild(NULL, NULL, NULL, NULL));
|
|
xmlFreeNode(xmlNewComment(NULL));
|
|
xmlFreeDoc(xmlNewDoc(NULL));
|
|
xmlFreeNode(xmlNewDocComment(NULL, NULL));
|
|
xmlNewDocElementContent(NULL, NULL, 0);
|
|
xmlFreeNode(xmlNewDocFragment(NULL));
|
|
xmlFreeNode(xmlNewDocNode(NULL, NULL, NULL, NULL));
|
|
xmlFreeNode(xmlNewDocNodeEatName(NULL, NULL, NULL, NULL));
|
|
xmlFreeNode(xmlNewDocPI(NULL, NULL, NULL));
|
|
xmlNewDocProp(NULL, NULL, NULL);
|
|
xmlFreeNode(xmlNewDocRawNode(NULL, NULL, NULL, NULL));
|
|
xmlFreeNode(xmlNewDocText(NULL, NULL));
|
|
xmlFreeNode(xmlNewDocTextLen(NULL, NULL, 0));
|
|
xmlFreeDtd(xmlNewDtd(NULL, NULL, NULL, NULL));
|
|
xmlNewElementContent(NULL, 0);
|
|
xmlNewEntity(NULL, NULL, 0, NULL, NULL, NULL);
|
|
xmlFreeInputStream(xmlNewEntityInputStream(NULL, NULL));
|
|
xmlFreeInputStream(xmlNewIOInputStream(NULL, NULL, 0));
|
|
xmlFreeInputStream(xmlNewInputFromFd(NULL, 0, 0));
|
|
xmlFreeInputStream(xmlNewInputFromFile(NULL, NULL));
|
|
xmlFreeInputStream(xmlNewInputFromIO(NULL, 0, 0, NULL, 0));
|
|
xmlFreeInputStream(xmlNewInputFromMemory(NULL, NULL, 0, 0));
|
|
xmlFreeInputStream(xmlNewInputFromString(NULL, NULL, 0));
|
|
xmlNewInputFromUrl(NULL, 0, NULL);
|
|
xmlFreeInputStream(xmlNewInputStream(NULL));
|
|
xmlFreeMutex(xmlNewMutex());
|
|
xmlFreeNode(xmlNewNode(NULL, NULL));
|
|
xmlFreeNode(xmlNewNodeEatName(NULL, NULL));
|
|
xmlFreeNs(xmlNewNs(NULL, NULL, NULL));
|
|
xmlNewNsProp(NULL, NULL, NULL, NULL);
|
|
xmlNewNsPropEatName(NULL, NULL, NULL, NULL);
|
|
xmlFreeNode(xmlNewPI(NULL, NULL));
|
|
xmlFreeParserCtxt(xmlNewParserCtxt());
|
|
xmlNewProp(NULL, NULL, NULL);
|
|
xmlFreeRMutex(xmlNewRMutex());
|
|
xmlFreeNode(xmlNewReference(NULL, NULL));
|
|
xmlFreeParserCtxt(xmlNewSAXParserCtxt(NULL, NULL));
|
|
xmlFreeInputStream(xmlNewStringInputStream(NULL, NULL));
|
|
xmlFreeNode(xmlNewText(NULL));
|
|
xmlFreeNode(xmlNewTextChild(NULL, NULL, NULL, NULL));
|
|
xmlFreeNode(xmlNewTextLen(NULL, 0));
|
|
xmlNextChar(NULL);
|
|
xmlFreeNode(xmlNextElementSibling(NULL));
|
|
xmlFreeInputStream(xmlNoNetExternalEntityLoader(NULL, NULL, NULL));
|
|
xmlNodeAddContent(NULL, NULL);
|
|
xmlNodeAddContentLen(NULL, NULL, 0);
|
|
xmlNodeBufGetContent(NULL, NULL);
|
|
xmlNodeGetAttrValue(NULL, NULL, NULL, NULL);
|
|
xmlFree(xmlNodeGetBase(NULL, NULL));
|
|
xmlNodeGetBaseSafe(NULL, NULL, NULL);
|
|
xmlFree(xmlNodeGetContent(NULL));
|
|
xmlFree(xmlNodeGetLang(NULL));
|
|
xmlNodeGetSpacePreserve(NULL);
|
|
xmlNodeIsText(NULL);
|
|
xmlFree(xmlNodeListGetRawString(NULL, NULL, 0));
|
|
xmlFree(xmlNodeListGetString(NULL, NULL, 0));
|
|
xmlNodeSetBase(NULL, NULL);
|
|
xmlNodeSetContent(NULL, NULL);
|
|
xmlNodeSetContentLen(NULL, NULL, 0);
|
|
xmlNodeSetLang(NULL, NULL);
|
|
xmlNodeSetName(NULL, NULL);
|
|
xmlNodeSetSpacePreserve(NULL, 0);
|
|
xmlNormalizeURIPath(NULL);
|
|
xmlFree(xmlNormalizeWindowsPath(NULL));
|
|
xmlOpenCharEncodingHandler(NULL, 0, NULL);
|
|
xmlOutputBufferCreateFilenameDefault(0);
|
|
xmlFree(xmlParseAttValue(NULL));
|
|
xmlParseCharEncoding(NULL);
|
|
xmlParseContent(NULL);
|
|
xmlParseCtxtExternalEntity(NULL, NULL, NULL, NULL);
|
|
xmlParseDocument(NULL);
|
|
xmlParseEntityRef(NULL);
|
|
xmlParseExtParsedEnt(NULL);
|
|
xmlParseInNodeContext(NULL, NULL, 0, 0, NULL);
|
|
xmlFreeURI(xmlParseURI(NULL));
|
|
xmlFreeURI(xmlParseURIRaw(NULL, 0));
|
|
xmlParseURIReference(NULL, NULL);
|
|
xmlParseURISafe(NULL, NULL);
|
|
xmlParserAddNodeInfo(NULL, NULL);
|
|
xmlParserFindNodeInfo(NULL, NULL);
|
|
xmlParserFindNodeInfoIndex(NULL, NULL);
|
|
xmlParserGetDirectory(NULL);
|
|
xmlFreeParserInputBuffer(xmlParserInputBufferCreateFd(0, 0));
|
|
xmlFreeParserInputBuffer(xmlParserInputBufferCreateFile(NULL, 0));
|
|
xmlFreeParserInputBuffer(xmlParserInputBufferCreateFilename(NULL, 0));
|
|
xmlParserInputBufferCreateFilenameDefault(0);
|
|
xmlFreeParserInputBuffer(xmlParserInputBufferCreateIO(0, 0, NULL, 0));
|
|
xmlFreeParserInputBuffer(xmlParserInputBufferCreateMem(NULL, 0, 0));
|
|
xmlFreeParserInputBuffer(xmlParserInputBufferCreateStatic(NULL, 0, 0));
|
|
xmlParserInputBufferGrow(NULL, 0);
|
|
xmlParserInputBufferPush(NULL, 0, NULL);
|
|
xmlParserInputBufferRead(NULL, 0);
|
|
xmlParserInputGrow(NULL, 0);
|
|
xmlParserInputRead(NULL, 0);
|
|
xmlParserInputShrink(NULL);
|
|
xmlParserPrintFileContext(NULL);
|
|
xmlParserPrintFileInfo(NULL);
|
|
xmlFree(xmlPathToURI(NULL));
|
|
xmlPedanticParserDefault(0);
|
|
xmlPopInput(NULL);
|
|
xmlPopInputCallbacks();
|
|
xmlFreeNode(xmlPreviousElementSibling(NULL));
|
|
xmlPrintURI(NULL, NULL);
|
|
xmlPushInput(NULL, NULL);
|
|
xmlRMutexLock(NULL);
|
|
xmlRMutexUnlock(NULL);
|
|
xmlFreeDoc(xmlReadDoc(NULL, NULL, NULL, 0));
|
|
xmlFreeDoc(xmlReadFile(NULL, NULL, 0));
|
|
xmlFreeDoc(xmlReadIO(0, 0, NULL, NULL, NULL, 0));
|
|
xmlFreeDoc(xmlReadMemory(NULL, 0, NULL, NULL, 0));
|
|
xmlReconciliateNs(NULL, NULL);
|
|
xmlRegisterCharEncodingHandler(NULL);
|
|
xmlRegisterDefaultInputCallbacks();
|
|
xmlRegisterInputCallbacks(0, 0, 0, 0);
|
|
xmlRegisterNodeDefault(0);
|
|
xmlRemoveID(NULL, NULL);
|
|
xmlRemoveProp(NULL);
|
|
xmlRemoveRef(NULL, NULL);
|
|
xmlFreeNode(xmlReplaceNode(NULL, NULL));
|
|
xmlResetError(NULL);
|
|
xmlResetLastError();
|
|
xmlSAX2AttributeDecl(NULL, NULL, NULL, 0, 0, NULL, NULL);
|
|
xmlSAX2CDataBlock(NULL, NULL, 0);
|
|
xmlSAX2Characters(NULL, NULL, 0);
|
|
xmlSAX2Comment(NULL, NULL);
|
|
xmlSAX2ElementDecl(NULL, NULL, 0, NULL);
|
|
xmlSAX2EndDocument(NULL);
|
|
xmlSAX2EndElement(NULL, NULL);
|
|
xmlSAX2EndElementNs(NULL, NULL, NULL, NULL);
|
|
xmlSAX2EntityDecl(NULL, NULL, 0, NULL, NULL, NULL);
|
|
xmlSAX2ExternalSubset(NULL, NULL, NULL, NULL);
|
|
xmlSAX2GetColumnNumber(NULL);
|
|
xmlSAX2GetEntity(NULL, NULL);
|
|
xmlSAX2GetLineNumber(NULL);
|
|
xmlSAX2GetParameterEntity(NULL, NULL);
|
|
xmlSAX2GetPublicId(NULL);
|
|
xmlSAX2GetSystemId(NULL);
|
|
xmlSAX2HasExternalSubset(NULL);
|
|
xmlSAX2HasInternalSubset(NULL);
|
|
xmlSAX2IgnorableWhitespace(NULL, NULL, 0);
|
|
xmlSAX2InitDefaultSAXHandler(NULL, 0);
|
|
xmlSAX2InternalSubset(NULL, NULL, NULL, NULL);
|
|
xmlSAX2IsStandalone(NULL);
|
|
xmlSAX2NotationDecl(NULL, NULL, NULL, NULL);
|
|
xmlSAX2ProcessingInstruction(NULL, NULL, NULL);
|
|
xmlSAX2Reference(NULL, NULL);
|
|
xmlFreeInputStream(xmlSAX2ResolveEntity(NULL, NULL, NULL));
|
|
xmlSAX2SetDocumentLocator(NULL, NULL);
|
|
xmlSAX2StartDocument(NULL);
|
|
xmlSAX2StartElement(NULL, NULL, NULL);
|
|
xmlSAX2StartElementNs(NULL, NULL, NULL, NULL, 0, NULL, 0, 0, NULL);
|
|
xmlSAX2UnparsedEntityDecl(NULL, NULL, NULL, NULL, NULL);
|
|
xmlSAXVersion(NULL, 0);
|
|
xmlFree(xmlSaveUri(NULL));
|
|
xmlFreeNs(xmlSearchNs(NULL, NULL, NULL));
|
|
xmlFreeNs(xmlSearchNsByHref(NULL, NULL, NULL));
|
|
xmlSetBufferAllocationScheme(0);
|
|
xmlSetCompressMode(0);
|
|
xmlSetDocCompressMode(NULL, 0);
|
|
xmlSetExternalEntityLoader(0);
|
|
xmlSetGenericErrorFunc(NULL, 0);
|
|
xmlSetListDoc(NULL, NULL);
|
|
xmlSetNs(NULL, NULL);
|
|
xmlSetNsProp(NULL, NULL, NULL, NULL);
|
|
xmlSetProp(NULL, NULL, NULL);
|
|
xmlSetTreeDoc(NULL, NULL);
|
|
xmlSnprintfElementContent(NULL, 0, NULL, 0);
|
|
xmlFree(xmlSplitQName(NULL, NULL, NULL));
|
|
xmlFree(xmlSplitQName2(NULL, NULL));
|
|
xmlSplitQName3(NULL, NULL);
|
|
xmlStopParser(NULL);
|
|
xmlStrEqual(NULL, NULL);
|
|
xmlStrPrintf(NULL, 0, NULL, 0);
|
|
xmlStrQEqual(NULL, NULL, NULL);
|
|
xmlStrVPrintf(NULL, 0, NULL, 0);
|
|
xmlStrcasecmp(NULL, NULL);
|
|
xmlStrcasestr(NULL, NULL);
|
|
xmlFree(xmlStrcat(NULL, NULL));
|
|
xmlStrchr(NULL, 0);
|
|
xmlStrcmp(NULL, NULL);
|
|
xmlFree(xmlStrdup(NULL));
|
|
xmlStringCurrentChar(NULL, NULL, NULL);
|
|
xmlFree(xmlStringDecodeEntities(NULL, NULL, 0, 0, 0, 0));
|
|
xmlFreeNode(xmlStringGetNodeList(NULL, NULL));
|
|
xmlFree(xmlStringLenDecodeEntities(NULL, NULL, 0, 0, 0, 0, 0));
|
|
xmlFreeNode(xmlStringLenGetNodeList(NULL, NULL, 0));
|
|
xmlStrlen(NULL);
|
|
xmlStrncasecmp(NULL, NULL, 0);
|
|
xmlFree(xmlStrncat(NULL, NULL, 0));
|
|
xmlFree(xmlStrncatNew(NULL, NULL, 0));
|
|
xmlStrncmp(NULL, NULL, 0);
|
|
xmlFree(xmlStrndup(NULL, 0));
|
|
xmlStrstr(NULL, NULL);
|
|
xmlFree(xmlStrsub(NULL, 0, 0));
|
|
xmlSubstituteEntitiesDefault(0);
|
|
xmlSwitchEncoding(NULL, 0);
|
|
xmlSwitchEncodingName(NULL, NULL);
|
|
xmlSwitchInputEncoding(NULL, NULL, NULL);
|
|
xmlSwitchToEncoding(NULL, NULL);
|
|
xmlTextConcat(NULL, NULL, 0);
|
|
xmlFreeNode(xmlTextMerge(NULL, NULL));
|
|
xmlThrDefDeregisterNodeDefault(0);
|
|
xmlThrDefDoValidityCheckingDefaultValue(0);
|
|
xmlThrDefGetWarningsDefaultValue(0);
|
|
xmlThrDefKeepBlanksDefaultValue(0);
|
|
xmlThrDefLineNumbersDefaultValue(0);
|
|
xmlThrDefLoadExtDtdDefaultValue(0);
|
|
xmlThrDefOutputBufferCreateFilenameDefault(0);
|
|
xmlThrDefParserInputBufferCreateFilenameDefault(0);
|
|
xmlThrDefPedanticParserDefaultValue(0);
|
|
xmlThrDefRegisterNodeDefault(0);
|
|
xmlThrDefSetGenericErrorFunc(NULL, 0);
|
|
xmlThrDefSetStructuredErrorFunc(NULL, 0);
|
|
xmlThrDefSubstituteEntitiesDefaultValue(0);
|
|
xmlFree(xmlURIEscape(NULL));
|
|
xmlFree(xmlURIEscapeStr(NULL, NULL));
|
|
xmlURIUnescapeString(NULL, 0, NULL);
|
|
xmlUTF8Charcmp(NULL, NULL);
|
|
xmlUTF8Size(NULL);
|
|
xmlUTF8Strlen(NULL);
|
|
xmlUTF8Strloc(NULL, NULL);
|
|
xmlFree(xmlUTF8Strndup(NULL, 0));
|
|
xmlUTF8Strpos(NULL, 0);
|
|
xmlUTF8Strsize(NULL, 0);
|
|
xmlFree(xmlUTF8Strsub(NULL, 0, 0));
|
|
xmlUnlinkNode(NULL);
|
|
xmlUnlockLibrary();
|
|
xmlUnsetNsProp(NULL, NULL, NULL);
|
|
xmlUnsetProp(NULL, NULL);
|
|
xmlValidateNCName(NULL, 0);
|
|
xmlValidateNMToken(NULL, 0);
|
|
xmlValidateName(NULL, 0);
|
|
xmlValidateQName(NULL, 0);
|
|
|
|
#ifdef LIBXML_C14N_ENABLED
|
|
xmlC14NDocDumpMemory(NULL, NULL, 0, NULL, 0, NULL);
|
|
xmlC14NDocSave(NULL, NULL, 0, NULL, 0, NULL, 0);
|
|
xmlC14NDocSaveTo(NULL, NULL, 0, NULL, 0, NULL);
|
|
xmlC14NExecute(NULL, 0, NULL, 0, NULL, 0, NULL);
|
|
#endif /* LIBXML_C14N_ENABLED */
|
|
|
|
#ifdef LIBXML_CATALOG_ENABLED
|
|
xmlACatalogAdd(NULL, NULL, NULL, NULL);
|
|
xmlACatalogRemove(NULL, NULL);
|
|
xmlFree(xmlACatalogResolve(NULL, NULL, NULL));
|
|
xmlFree(xmlACatalogResolvePublic(NULL, NULL));
|
|
xmlFree(xmlACatalogResolveSystem(NULL, NULL));
|
|
xmlFree(xmlACatalogResolveURI(NULL, NULL));
|
|
xmlCatalogAdd(NULL, NULL, NULL);
|
|
xmlCatalogAddLocal(NULL, NULL);
|
|
xmlCatalogCleanup();
|
|
xmlCatalogConvert();
|
|
xmlCatalogFreeLocal(NULL);
|
|
xmlCatalogGetDefaults();
|
|
xmlCatalogIsEmpty(NULL);
|
|
xmlFree(xmlCatalogLocalResolve(NULL, NULL, NULL));
|
|
xmlFree(xmlCatalogLocalResolveURI(NULL, NULL));
|
|
xmlCatalogRemove(NULL);
|
|
xmlFree(xmlCatalogResolve(NULL, NULL));
|
|
xmlFree(xmlCatalogResolvePublic(NULL));
|
|
xmlFree(xmlCatalogResolveSystem(NULL));
|
|
xmlFree(xmlCatalogResolveURI(NULL));
|
|
xmlCatalogSetDebug(0);
|
|
xmlCatalogSetDefaultPrefer(0);
|
|
xmlCatalogSetDefaults(0);
|
|
xmlConvertSGMLCatalog(NULL);
|
|
xmlFreeCatalog(NULL);
|
|
xmlInitializeCatalog();
|
|
xmlFreeCatalog(xmlLoadACatalog(NULL));
|
|
xmlLoadCatalog(NULL);
|
|
xmlLoadCatalogs(NULL);
|
|
xmlFreeCatalog(xmlLoadSGMLSuperCatalog(NULL));
|
|
xmlFreeCatalog(xmlNewCatalog(0));
|
|
xmlFreeDoc(xmlParseCatalogFile(NULL));
|
|
#ifdef LIBXML_OUTPUT_ENABLED
|
|
xmlACatalogDump(NULL, NULL);
|
|
xmlCatalogDump(NULL);
|
|
#endif /* LIBXML_OUTPUT_ENABLED */
|
|
#endif /* LIBXML_CATALOG_ENABLED */
|
|
|
|
#ifdef LIBXML_DEBUG_ENABLED
|
|
xmlDebugCheckDocument(NULL, NULL);
|
|
xmlDebugDumpAttr(NULL, NULL, 0);
|
|
xmlDebugDumpAttrList(NULL, NULL, 0);
|
|
xmlDebugDumpDocumentHead(NULL, NULL);
|
|
xmlDebugDumpEntities(NULL, NULL);
|
|
xmlDebugDumpNodeList(NULL, NULL, 0);
|
|
xmlDebugDumpOneNode(NULL, NULL, 0);
|
|
#endif /* LIBXML_DEBUG_ENABLED */
|
|
|
|
#ifdef LIBXML_HTML_ENABLED
|
|
htmlAttrAllowed(NULL, NULL, 0);
|
|
htmlAutoCloseTag(NULL, NULL, NULL);
|
|
htmlFreeParserCtxt(htmlCreateFileParserCtxt(NULL, NULL));
|
|
htmlFreeParserCtxt(htmlCreateMemoryParserCtxt(NULL, 0));
|
|
xmlFreeDoc(htmlCtxtParseDocument(NULL, NULL));
|
|
xmlFreeDoc(htmlCtxtReadDoc(NULL, NULL, NULL, NULL, 0));
|
|
xmlFreeDoc(htmlCtxtReadFd(NULL, 0, NULL, NULL, 0));
|
|
xmlFreeDoc(htmlCtxtReadFile(NULL, NULL, NULL, 0));
|
|
xmlFreeDoc(htmlCtxtReadIO(NULL, 0, 0, NULL, NULL, NULL, 0));
|
|
xmlFreeDoc(htmlCtxtReadMemory(NULL, NULL, 0, NULL, NULL, 0));
|
|
htmlCtxtReset(NULL);
|
|
htmlCtxtSetOptions(NULL, 0);
|
|
htmlCtxtUseOptions(NULL, 0);
|
|
htmlDefaultSAXHandlerInit();
|
|
htmlElementAllowedHere(NULL, NULL);
|
|
htmlElementStatusHere(NULL, NULL);
|
|
htmlEncodeEntities(NULL, NULL, NULL, NULL, 0);
|
|
htmlEntityLookup(NULL);
|
|
htmlEntityValueLookup(0);
|
|
htmlFreeParserCtxt(NULL);
|
|
htmlGetMetaEncoding(NULL);
|
|
htmlHandleOmittedElem(0);
|
|
htmlInitAutoClose();
|
|
htmlIsAutoClosed(NULL, NULL);
|
|
htmlIsBooleanAttr(NULL);
|
|
htmlIsScriptAttribute(NULL);
|
|
xmlFreeDoc(htmlNewDoc(NULL, NULL));
|
|
xmlFreeDoc(htmlNewDocNoDtD(NULL, NULL));
|
|
htmlFreeParserCtxt(htmlNewParserCtxt());
|
|
htmlFreeParserCtxt(htmlNewSAXParserCtxt(NULL, NULL));
|
|
htmlNodeStatus(NULL, 0);
|
|
htmlParseCharRef(NULL);
|
|
xmlFreeDoc(htmlParseDoc(NULL, NULL));
|
|
htmlParseDocument(NULL);
|
|
htmlParseElement(NULL);
|
|
htmlParseEntityRef(NULL, NULL);
|
|
xmlFreeDoc(htmlParseFile(NULL, NULL));
|
|
xmlFreeDoc(htmlReadDoc(NULL, NULL, NULL, 0));
|
|
xmlFreeDoc(htmlReadFile(NULL, NULL, 0));
|
|
xmlFreeDoc(htmlReadIO(0, 0, NULL, NULL, NULL, 0));
|
|
xmlFreeDoc(htmlReadMemory(NULL, 0, NULL, NULL, 0));
|
|
xmlFreeDoc(htmlSAXParseDoc(NULL, NULL, NULL, NULL));
|
|
xmlFreeDoc(htmlSAXParseFile(NULL, NULL, NULL, NULL));
|
|
htmlSetMetaEncoding(NULL, NULL);
|
|
htmlTagLookup(NULL);
|
|
htmlUTF8ToHtml(NULL, NULL, NULL, NULL);
|
|
xmlSAX2InitHtmlDefaultSAXHandler(NULL);
|
|
#ifdef LIBXML_OUTPUT_ENABLED
|
|
htmlDocContentDumpFormatOutput(NULL, NULL, NULL, 0);
|
|
htmlDocContentDumpOutput(NULL, NULL, NULL);
|
|
htmlDocDump(NULL, NULL);
|
|
htmlDocDumpMemory(NULL, NULL, NULL);
|
|
htmlDocDumpMemoryFormat(NULL, NULL, NULL, 0);
|
|
htmlNodeDump(NULL, NULL, NULL);
|
|
htmlNodeDumpFile(NULL, NULL, NULL);
|
|
htmlNodeDumpFileFormat(NULL, NULL, NULL, NULL, 0);
|
|
htmlNodeDumpFormatOutput(NULL, NULL, NULL, NULL, 0);
|
|
htmlNodeDumpOutput(NULL, NULL, NULL, NULL);
|
|
htmlSaveFile(NULL, NULL);
|
|
htmlSaveFileEnc(NULL, NULL, NULL);
|
|
htmlSaveFileFormat(NULL, NULL, NULL, 0);
|
|
#endif /* LIBXML_OUTPUT_ENABLED */
|
|
#ifdef LIBXML_PUSH_ENABLED
|
|
htmlFreeParserCtxt(htmlCreatePushParserCtxt(NULL, NULL, NULL, 0, NULL, 0));
|
|
htmlParseChunk(NULL, NULL, 0, 0);
|
|
#endif /* LIBXML_PUSH_ENABLED */
|
|
#endif /* LIBXML_HTML_ENABLED */
|
|
|
|
#ifdef LIBXML_MODULES_ENABLED
|
|
xmlModuleClose(NULL);
|
|
xmlModuleFree(NULL);
|
|
xmlModuleFree(xmlModuleOpen(NULL, 0));
|
|
xmlModuleSymbol(NULL, NULL, NULL);
|
|
#endif /* LIBXML_MODULES_ENABLED */
|
|
|
|
#ifdef LIBXML_OUTPUT_ENABLED
|
|
xmlOutputBufferClose(__xmlOutputBufferCreateFilename(NULL, NULL, 0));
|
|
xmlOutputBufferClose(xmlAllocOutputBuffer(NULL));
|
|
xmlAttrSerializeTxtContent(NULL, NULL, NULL, NULL);
|
|
xmlBufNodeDump(NULL, NULL, NULL, 0, 0);
|
|
xmlCleanupOutputCallbacks();
|
|
xmlDocDump(NULL, NULL);
|
|
xmlDocDumpFormatMemory(NULL, NULL, NULL, 0);
|
|
xmlDocDumpFormatMemoryEnc(NULL, NULL, NULL, NULL, 0);
|
|
xmlDocDumpMemory(NULL, NULL, NULL);
|
|
xmlDocDumpMemoryEnc(NULL, NULL, NULL, NULL);
|
|
xmlDocFormatDump(NULL, NULL, 0);
|
|
xmlDumpAttributeDecl(NULL, NULL);
|
|
xmlDumpAttributeTable(NULL, NULL);
|
|
xmlDumpElementDecl(NULL, NULL);
|
|
xmlDumpElementTable(NULL, NULL);
|
|
xmlDumpEntitiesTable(NULL, NULL);
|
|
xmlDumpEntityDecl(NULL, NULL);
|
|
xmlDumpNotationDecl(NULL, NULL);
|
|
xmlDumpNotationTable(NULL, NULL);
|
|
xmlElemDump(NULL, NULL, NULL);
|
|
xmlNodeDump(NULL, NULL, NULL, 0, 0);
|
|
xmlNodeDumpOutput(NULL, NULL, NULL, 0, 0, NULL);
|
|
xmlOutputBufferClose(NULL);
|
|
xmlOutputBufferClose(xmlOutputBufferCreateBuffer(NULL, NULL));
|
|
xmlOutputBufferClose(xmlOutputBufferCreateFd(0, NULL));
|
|
xmlOutputBufferClose(xmlOutputBufferCreateFile(NULL, NULL));
|
|
xmlOutputBufferClose(xmlOutputBufferCreateFilename(NULL, NULL, 0));
|
|
xmlOutputBufferClose(xmlOutputBufferCreateIO(0, 0, NULL, NULL));
|
|
xmlOutputBufferFlush(NULL);
|
|
xmlOutputBufferGetContent(NULL);
|
|
xmlOutputBufferGetSize(NULL);
|
|
xmlOutputBufferWrite(NULL, 0, NULL);
|
|
xmlOutputBufferWriteEscape(NULL, NULL, 0);
|
|
xmlOutputBufferWriteString(NULL, NULL);
|
|
xmlPopOutputCallbacks();
|
|
xmlRegisterDefaultOutputCallbacks();
|
|
xmlRegisterOutputCallbacks(0, 0, 0, 0);
|
|
xmlSaveClose(NULL);
|
|
xmlSaveDoc(NULL, NULL);
|
|
xmlSaveFile(NULL, NULL);
|
|
xmlSaveFileEnc(NULL, NULL, NULL);
|
|
xmlSaveFileTo(NULL, NULL, NULL);
|
|
xmlSaveFinish(NULL);
|
|
xmlSaveFlush(NULL);
|
|
xmlSaveFormatFile(NULL, NULL, 0);
|
|
xmlSaveFormatFileEnc(NULL, NULL, NULL, 0);
|
|
xmlSaveFormatFileTo(NULL, NULL, NULL, 0);
|
|
xmlSaveSetAttrEscape(NULL, 0);
|
|
xmlSaveSetEscape(NULL, 0);
|
|
xmlSaveSetIndentString(NULL, NULL);
|
|
xmlSaveClose(xmlSaveToBuffer(NULL, NULL, 0));
|
|
xmlSaveClose(xmlSaveToFd(0, NULL, 0));
|
|
xmlSaveClose(xmlSaveToFilename(NULL, NULL, 0));
|
|
xmlSaveClose(xmlSaveToIO(0, 0, NULL, NULL, 0));
|
|
xmlSaveTree(NULL, NULL);
|
|
xmlSprintfElementContent(NULL, NULL, 0);
|
|
xmlThrDefIndentTreeOutput(0);
|
|
xmlThrDefSaveNoEmptyTags(0);
|
|
xmlThrDefTreeIndentString(NULL);
|
|
xmlUTF8ToIsolat1(NULL, NULL, NULL, NULL);
|
|
#endif /* LIBXML_OUTPUT_ENABLED */
|
|
|
|
#ifdef LIBXML_PATTERN_ENABLED
|
|
xmlFreePattern(NULL);
|
|
xmlFreePatternList(NULL);
|
|
xmlFreeStreamCtxt(NULL);
|
|
xmlPatternCompileSafe(NULL, NULL, 0, NULL, NULL);
|
|
xmlPatternFromRoot(NULL);
|
|
xmlPatternGetStreamCtxt(NULL);
|
|
xmlPatternMatch(NULL, NULL);
|
|
xmlPatternMaxDepth(NULL);
|
|
xmlPatternMinDepth(NULL);
|
|
xmlPatternStreamable(NULL);
|
|
xmlPatterncompile(NULL, NULL, 0, NULL);
|
|
xmlStreamPop(NULL);
|
|
xmlStreamPush(NULL, NULL, NULL);
|
|
xmlStreamPushAttr(NULL, NULL, NULL);
|
|
xmlStreamPushNode(NULL, NULL, NULL, 0);
|
|
xmlStreamWantsAnyNode(NULL);
|
|
#endif /* LIBXML_PATTERN_ENABLED */
|
|
|
|
#ifdef LIBXML_PUSH_ENABLED
|
|
xmlFreeParserCtxt(xmlCreatePushParserCtxt(NULL, NULL, NULL, 0, NULL));
|
|
xmlParseChunk(NULL, NULL, 0, 0);
|
|
#endif /* LIBXML_PUSH_ENABLED */
|
|
|
|
#ifdef LIBXML_READER_ENABLED
|
|
xmlFreeTextReader(NULL);
|
|
xmlNewTextReader(NULL, NULL);
|
|
xmlNewTextReaderFilename(NULL);
|
|
xmlReaderForDoc(NULL, NULL, NULL, 0);
|
|
xmlReaderForFile(NULL, NULL, 0);
|
|
xmlReaderForIO(0, 0, NULL, NULL, NULL, 0);
|
|
xmlReaderForMemory(NULL, 0, NULL, NULL, 0);
|
|
xmlReaderNewDoc(NULL, NULL, NULL, NULL, 0);
|
|
xmlReaderNewFd(NULL, 0, NULL, NULL, 0);
|
|
xmlReaderNewFile(NULL, NULL, NULL, 0);
|
|
xmlReaderNewIO(NULL, 0, 0, NULL, NULL, NULL, 0);
|
|
xmlReaderNewMemory(NULL, NULL, 0, NULL, NULL, 0);
|
|
xmlReaderNewWalker(NULL, NULL);
|
|
xmlReaderWalker(NULL);
|
|
xmlTextReaderAttributeCount(NULL);
|
|
xmlFree(xmlTextReaderBaseUri(NULL));
|
|
xmlTextReaderByteConsumed(NULL);
|
|
xmlTextReaderClose(NULL);
|
|
xmlTextReaderConstBaseUri(NULL);
|
|
xmlTextReaderConstEncoding(NULL);
|
|
xmlTextReaderConstLocalName(NULL);
|
|
xmlTextReaderConstName(NULL);
|
|
xmlTextReaderConstNamespaceUri(NULL);
|
|
xmlTextReaderConstPrefix(NULL);
|
|
xmlTextReaderConstString(NULL, NULL);
|
|
xmlTextReaderConstValue(NULL);
|
|
xmlTextReaderConstXmlLang(NULL);
|
|
xmlTextReaderConstXmlVersion(NULL);
|
|
xmlFreeDoc(xmlTextReaderCurrentDoc(NULL));
|
|
xmlFreeNode(xmlTextReaderCurrentNode(NULL));
|
|
xmlTextReaderDepth(NULL);
|
|
xmlFreeNode(xmlTextReaderExpand(NULL));
|
|
xmlFree(xmlTextReaderGetAttribute(NULL, NULL));
|
|
xmlFree(xmlTextReaderGetAttributeNo(NULL, 0));
|
|
xmlFree(xmlTextReaderGetAttributeNs(NULL, NULL, NULL));
|
|
xmlTextReaderGetErrorHandler(NULL, NULL, NULL);
|
|
xmlTextReaderGetLastError(NULL);
|
|
xmlTextReaderGetParserColumnNumber(NULL);
|
|
xmlTextReaderGetParserLineNumber(NULL);
|
|
xmlTextReaderGetParserProp(NULL, 0);
|
|
xmlFreeParserInputBuffer(xmlTextReaderGetRemainder(NULL));
|
|
xmlTextReaderHasAttributes(NULL);
|
|
xmlTextReaderHasValue(NULL);
|
|
xmlTextReaderIsDefault(NULL);
|
|
xmlTextReaderIsEmptyElement(NULL);
|
|
xmlTextReaderIsNamespaceDecl(NULL);
|
|
xmlTextReaderIsValid(NULL);
|
|
xmlFree(xmlTextReaderLocalName(NULL));
|
|
xmlFree(xmlTextReaderLocatorBaseURI(NULL));
|
|
xmlTextReaderLocatorLineNumber(NULL);
|
|
xmlFree(xmlTextReaderLookupNamespace(NULL, NULL));
|
|
xmlTextReaderMoveToAttribute(NULL, NULL);
|
|
xmlTextReaderMoveToAttributeNo(NULL, 0);
|
|
xmlTextReaderMoveToAttributeNs(NULL, NULL, NULL);
|
|
xmlTextReaderMoveToElement(NULL);
|
|
xmlTextReaderMoveToFirstAttribute(NULL);
|
|
xmlTextReaderMoveToNextAttribute(NULL);
|
|
xmlFree(xmlTextReaderName(NULL));
|
|
xmlFree(xmlTextReaderNamespaceUri(NULL));
|
|
xmlTextReaderNext(NULL);
|
|
xmlTextReaderNextSibling(NULL);
|
|
xmlTextReaderNodeType(NULL);
|
|
xmlTextReaderNormalization(NULL);
|
|
xmlFree(xmlTextReaderPrefix(NULL));
|
|
xmlFreeNode(xmlTextReaderPreserve(NULL));
|
|
xmlTextReaderQuoteChar(NULL);
|
|
xmlTextReaderRead(NULL);
|
|
xmlTextReaderReadAttributeValue(NULL);
|
|
xmlTextReaderReadState(NULL);
|
|
xmlFree(xmlTextReaderReadString(NULL));
|
|
xmlTextReaderSetErrorHandler(NULL, 0, NULL);
|
|
xmlTextReaderSetMaxAmplification(NULL, 0);
|
|
xmlTextReaderSetParserProp(NULL, 0, 0);
|
|
xmlTextReaderSetResourceLoader(NULL, 0, NULL);
|
|
xmlTextReaderSetStructuredErrorHandler(NULL, 0, NULL);
|
|
xmlTextReaderSetup(NULL, NULL, NULL, NULL, 0);
|
|
xmlTextReaderStandalone(NULL);
|
|
xmlFree(xmlTextReaderValue(NULL));
|
|
xmlFree(xmlTextReaderXmlLang(NULL));
|
|
#ifdef LIBXML_PATTERN_ENABLED
|
|
xmlTextReaderPreservePattern(NULL, NULL, NULL);
|
|
#endif /* LIBXML_PATTERN_ENABLED */
|
|
#ifdef LIBXML_RELAXNG_ENABLED
|
|
xmlTextReaderRelaxNGSetSchema(NULL, NULL);
|
|
xmlTextReaderRelaxNGValidate(NULL, NULL);
|
|
xmlTextReaderRelaxNGValidateCtxt(NULL, NULL, 0);
|
|
#endif /* LIBXML_RELAXNG_ENABLED */
|
|
#ifdef LIBXML_SCHEMAS_ENABLED
|
|
xmlTextReaderSchemaValidate(NULL, NULL);
|
|
xmlTextReaderSchemaValidateCtxt(NULL, NULL, 0);
|
|
xmlTextReaderSetSchema(NULL, NULL);
|
|
#endif /* LIBXML_SCHEMAS_ENABLED */
|
|
#ifdef LIBXML_WRITER_ENABLED
|
|
xmlFree(xmlTextReaderReadInnerXml(NULL));
|
|
xmlFree(xmlTextReaderReadOuterXml(NULL));
|
|
#endif /* LIBXML_WRITER_ENABLED */
|
|
#endif /* LIBXML_READER_ENABLED */
|
|
|
|
#ifdef LIBXML_REGEXP_ENABLED
|
|
xmlAutomataCompile(NULL);
|
|
xmlAutomataGetInitState(NULL);
|
|
xmlAutomataIsDeterminist(NULL);
|
|
xmlAutomataNewAllTrans(NULL, NULL, NULL, 0);
|
|
xmlAutomataNewCountTrans(NULL, NULL, NULL, NULL, 0, 0, NULL);
|
|
xmlAutomataNewCountTrans2(NULL, NULL, NULL, NULL, NULL, 0, 0, NULL);
|
|
xmlAutomataNewCountedTrans(NULL, NULL, NULL, 0);
|
|
xmlAutomataNewCounter(NULL, 0, 0);
|
|
xmlAutomataNewCounterTrans(NULL, NULL, NULL, 0);
|
|
xmlAutomataNewEpsilon(NULL, NULL, NULL);
|
|
xmlAutomataNewNegTrans(NULL, NULL, NULL, NULL, NULL, NULL);
|
|
xmlAutomataNewOnceTrans(NULL, NULL, NULL, NULL, 0, 0, NULL);
|
|
xmlAutomataNewOnceTrans2(NULL, NULL, NULL, NULL, NULL, 0, 0, NULL);
|
|
xmlAutomataNewState(NULL);
|
|
xmlAutomataNewTransition(NULL, NULL, NULL, NULL, NULL);
|
|
xmlAutomataNewTransition2(NULL, NULL, NULL, NULL, NULL, NULL);
|
|
xmlAutomataSetFinalState(NULL, NULL);
|
|
xmlFreeAutomata(NULL);
|
|
xmlFreeAutomata(xmlNewAutomata());
|
|
xmlRegExecErrInfo(NULL, NULL, NULL, NULL, NULL, NULL);
|
|
xmlRegExecNextValues(NULL, NULL, NULL, NULL, NULL);
|
|
xmlRegExecPushString(NULL, NULL, NULL);
|
|
xmlRegExecPushString2(NULL, NULL, NULL, NULL);
|
|
xmlRegFreeExecCtxt(NULL);
|
|
xmlRegFreeRegexp(NULL);
|
|
xmlRegNewExecCtxt(NULL, 0, NULL);
|
|
xmlRegexpCompile(NULL);
|
|
xmlRegexpExec(NULL, NULL);
|
|
xmlRegexpIsDeterminist(NULL);
|
|
xmlRegexpPrint(NULL, NULL);
|
|
#endif /* LIBXML_REGEXP_ENABLED */
|
|
|
|
#ifdef LIBXML_RELAXNG_ENABLED
|
|
xmlRelaxNGCleanupTypes();
|
|
xmlRelaxNGFree(NULL);
|
|
xmlRelaxNGFreeParserCtxt(NULL);
|
|
xmlRelaxNGFreeValidCtxt(NULL);
|
|
xmlRelaxNGGetParserErrors(NULL, NULL, NULL, NULL);
|
|
xmlRelaxNGGetValidErrors(NULL, NULL, NULL, NULL);
|
|
xmlRelaxNGInitTypes();
|
|
xmlRelaxNGNewDocParserCtxt(NULL);
|
|
xmlRelaxNGNewMemParserCtxt(NULL, 0);
|
|
xmlRelaxNGNewParserCtxt(NULL);
|
|
xmlRelaxNGFreeValidCtxt(xmlRelaxNGNewValidCtxt(NULL));
|
|
xmlRelaxNGParse(NULL);
|
|
xmlRelaxNGSetParserErrors(NULL, 0, 0, NULL);
|
|
xmlRelaxNGSetParserStructuredErrors(NULL, 0, NULL);
|
|
xmlRelaxNGSetResourceLoader(NULL, 0, NULL);
|
|
xmlRelaxNGSetValidErrors(NULL, 0, 0, NULL);
|
|
xmlRelaxNGSetValidStructuredErrors(NULL, 0, NULL);
|
|
xmlRelaxNGValidateDoc(NULL, NULL);
|
|
xmlRelaxNGValidateFullElement(NULL, NULL, NULL);
|
|
xmlRelaxNGValidatePopElement(NULL, NULL, NULL);
|
|
xmlRelaxNGValidatePushCData(NULL, NULL, 0);
|
|
xmlRelaxNGValidatePushElement(NULL, NULL, NULL);
|
|
xmlRelaxParserSetFlag(NULL, 0);
|
|
#ifdef LIBXML_OUTPUT_ENABLED
|
|
xmlRelaxNGDump(NULL, NULL);
|
|
xmlRelaxNGDumpTree(NULL, NULL);
|
|
#endif /* LIBXML_OUTPUT_ENABLED */
|
|
#endif /* LIBXML_RELAXNG_ENABLED */
|
|
|
|
#ifdef LIBXML_SAX1_ENABLED
|
|
xmlParseBalancedChunkMemory(NULL, NULL, NULL, 0, NULL, NULL);
|
|
xmlParseBalancedChunkMemoryRecover(NULL, NULL, NULL, 0, NULL, NULL, 0);
|
|
xmlFreeDoc(xmlParseDoc(NULL));
|
|
xmlFreeDoc(xmlParseEntity(NULL));
|
|
xmlParseExternalEntity(NULL, NULL, NULL, 0, NULL, NULL, NULL);
|
|
xmlFreeDoc(xmlParseFile(NULL));
|
|
xmlFreeDoc(xmlParseMemory(NULL, 0));
|
|
xmlFreeDoc(xmlRecoverDoc(NULL));
|
|
xmlFreeDoc(xmlRecoverFile(NULL));
|
|
xmlFreeDoc(xmlRecoverMemory(NULL, 0));
|
|
xmlSAXDefaultVersion(0);
|
|
xmlFreeDoc(xmlSAXParseDoc(NULL, NULL, 0));
|
|
xmlFreeDoc(xmlSAXParseEntity(NULL, NULL));
|
|
xmlFreeDoc(xmlSAXParseFile(NULL, NULL, 0));
|
|
xmlFreeDoc(xmlSAXParseFileWithData(NULL, NULL, 0, NULL));
|
|
xmlFreeDoc(xmlSAXParseMemory(NULL, NULL, 0, 0));
|
|
xmlFreeDoc(xmlSAXParseMemoryWithData(NULL, NULL, 0, 0, NULL));
|
|
xmlSAXUserParseFile(NULL, NULL, NULL);
|
|
xmlSAXUserParseMemory(NULL, NULL, NULL, 0);
|
|
xmlSetupParserForBuffer(NULL, NULL, NULL);
|
|
#endif /* LIBXML_SAX1_ENABLED */
|
|
|
|
#ifdef LIBXML_SCHEMAS_ENABLED
|
|
xmlSchemaCheckFacet(NULL, NULL, NULL, NULL);
|
|
xmlSchemaCleanupTypes();
|
|
xmlFree(xmlSchemaCollapseString(NULL));
|
|
xmlSchemaCompareValues(NULL, NULL);
|
|
xmlSchemaCompareValuesWhtsp(NULL, 0, NULL, 0);
|
|
xmlSchemaFreeValue(xmlSchemaCopyValue(NULL));
|
|
xmlSchemaFree(NULL);
|
|
xmlSchemaFreeFacet(NULL);
|
|
xmlSchemaFreeParserCtxt(NULL);
|
|
xmlSchemaFreeType(NULL);
|
|
xmlSchemaFreeValidCtxt(NULL);
|
|
xmlSchemaFreeValue(NULL);
|
|
xmlSchemaFreeWildcard(NULL);
|
|
xmlSchemaGetBuiltInListSimpleTypeItemType(NULL);
|
|
xmlSchemaGetBuiltInType(0);
|
|
xmlSchemaGetCanonValue(NULL, NULL);
|
|
xmlSchemaGetCanonValueWhtsp(NULL, NULL, 0);
|
|
xmlSchemaGetFacetValueAsULong(NULL);
|
|
xmlSchemaGetParserErrors(NULL, NULL, NULL, NULL);
|
|
xmlSchemaGetPredefinedType(NULL, NULL);
|
|
xmlSchemaGetValType(NULL);
|
|
xmlSchemaGetValidErrors(NULL, NULL, NULL, NULL);
|
|
xmlSchemaInitTypes();
|
|
xmlSchemaIsBuiltInTypeFacet(NULL, 0);
|
|
xmlSchemaIsValid(NULL);
|
|
xmlSchemaNewDocParserCtxt(NULL);
|
|
xmlSchemaFreeFacet(xmlSchemaNewFacet());
|
|
xmlSchemaNewMemParserCtxt(NULL, 0);
|
|
xmlSchemaFreeValue(xmlSchemaNewNOTATIONValue(NULL, NULL));
|
|
xmlSchemaNewParserCtxt(NULL);
|
|
xmlSchemaFreeValue(xmlSchemaNewQNameValue(NULL, NULL));
|
|
xmlSchemaFreeValue(xmlSchemaNewStringValue(0, NULL));
|
|
xmlSchemaFreeValidCtxt(xmlSchemaNewValidCtxt(NULL));
|
|
xmlSchemaParse(NULL);
|
|
xmlSchemaSAXPlug(NULL, NULL, NULL);
|
|
xmlSchemaSAXUnplug(NULL);
|
|
xmlSchemaSetParserErrors(NULL, 0, 0, NULL);
|
|
xmlSchemaSetParserStructuredErrors(NULL, 0, NULL);
|
|
xmlSchemaSetResourceLoader(NULL, 0, NULL);
|
|
xmlSchemaSetValidErrors(NULL, 0, 0, NULL);
|
|
xmlSchemaSetValidOptions(NULL, 0);
|
|
xmlSchemaSetValidStructuredErrors(NULL, 0, NULL);
|
|
xmlSchemaValPredefTypeNode(NULL, NULL, NULL, NULL);
|
|
xmlSchemaValPredefTypeNodeNoNorm(NULL, NULL, NULL, NULL);
|
|
xmlSchemaValidCtxtGetOptions(NULL);
|
|
xmlFreeParserCtxt(xmlSchemaValidCtxtGetParserCtxt(NULL));
|
|
xmlSchemaValidateDoc(NULL, NULL);
|
|
xmlSchemaValidateFacet(NULL, NULL, NULL, NULL);
|
|
xmlSchemaValidateFacetWhtsp(NULL, 0, 0, NULL, NULL, 0);
|
|
xmlSchemaValidateFile(NULL, NULL, 0);
|
|
xmlSchemaValidateLengthFacet(NULL, NULL, NULL, NULL, NULL);
|
|
xmlSchemaValidateLengthFacetWhtsp(NULL, 0, NULL, NULL, NULL, 0);
|
|
xmlSchemaValidateListSimpleTypeFacet(NULL, NULL, 0, NULL);
|
|
xmlSchemaValidateOneElement(NULL, NULL);
|
|
xmlSchemaValidatePredefinedType(NULL, NULL, NULL);
|
|
xmlSchemaValidateSetFilename(NULL, NULL);
|
|
xmlSchemaValidateSetLocator(NULL, 0, NULL);
|
|
xmlSchemaValidateStream(NULL, NULL, 0, NULL, NULL);
|
|
xmlSchemaValueAppend(NULL, NULL);
|
|
xmlSchemaValueGetAsBoolean(NULL);
|
|
xmlSchemaValueGetAsString(NULL);
|
|
xmlSchemaFreeValue(xmlSchemaValueGetNext(NULL));
|
|
xmlFree(xmlSchemaWhiteSpaceReplace(NULL));
|
|
#ifdef LIBXML_DEBUG_ENABLED
|
|
xmlSchemaDump(NULL, NULL);
|
|
#endif /* LIBXML_DEBUG_ENABLED */
|
|
#endif /* LIBXML_SCHEMAS_ENABLED */
|
|
|
|
#ifdef LIBXML_SCHEMATRON_ENABLED
|
|
xmlSchematronFree(NULL);
|
|
xmlSchematronFreeParserCtxt(NULL);
|
|
xmlSchematronFreeValidCtxt(NULL);
|
|
xmlSchematronNewDocParserCtxt(NULL);
|
|
xmlSchematronNewMemParserCtxt(NULL, 0);
|
|
xmlSchematronNewParserCtxt(NULL);
|
|
xmlSchematronNewValidCtxt(NULL, 0);
|
|
xmlSchematronParse(NULL);
|
|
xmlSchematronSetValidStructuredErrors(NULL, 0, NULL);
|
|
xmlSchematronValidateDoc(NULL, NULL);
|
|
#endif /* LIBXML_SCHEMATRON_ENABLED */
|
|
|
|
#ifdef LIBXML_VALID_ENABLED
|
|
xmlFreeValidCtxt(xmlCtxtGetValidCtxt(NULL));
|
|
xmlFreeDtd(xmlCtxtParseDtd(NULL, NULL, NULL, NULL));
|
|
xmlCtxtValidateDocument(NULL, NULL);
|
|
xmlCtxtValidateDtd(NULL, NULL, NULL);
|
|
xmlFreeValidCtxt(NULL);
|
|
xmlFreeDtd(xmlIOParseDTD(NULL, NULL, 0));
|
|
xmlFreeValidCtxt(xmlNewValidCtxt());
|
|
xmlFreeDtd(xmlParseDTD(NULL, NULL));
|
|
xmlFreeDtd(xmlSAXParseDTD(NULL, NULL, NULL));
|
|
xmlFree(xmlValidCtxtNormalizeAttributeValue(NULL, NULL, NULL, NULL, NULL));
|
|
xmlValidGetPotentialChildren(NULL, NULL, NULL, 0);
|
|
xmlValidGetValidElements(NULL, NULL, NULL, 0);
|
|
xmlFree(xmlValidNormalizeAttributeValue(NULL, NULL, NULL, NULL));
|
|
xmlValidateAttributeDecl(NULL, NULL, NULL);
|
|
xmlValidateAttributeValue(0, NULL);
|
|
xmlValidateDocument(NULL, NULL);
|
|
xmlValidateDocumentFinal(NULL, NULL);
|
|
xmlValidateDtd(NULL, NULL, NULL);
|
|
xmlValidateDtdFinal(NULL, NULL);
|
|
xmlValidateElement(NULL, NULL, NULL);
|
|
xmlValidateElementDecl(NULL, NULL, NULL);
|
|
xmlValidateNameValue(NULL);
|
|
xmlValidateNamesValue(NULL);
|
|
xmlValidateNmtokenValue(NULL);
|
|
xmlValidateNmtokensValue(NULL);
|
|
xmlValidateNotationDecl(NULL, NULL, NULL);
|
|
xmlValidateNotationUse(NULL, NULL, NULL);
|
|
xmlValidateOneAttribute(NULL, NULL, NULL, NULL, NULL);
|
|
xmlValidateOneElement(NULL, NULL, NULL);
|
|
xmlValidateOneNamespace(NULL, NULL, NULL, NULL, NULL, NULL);
|
|
xmlValidateRoot(NULL, NULL);
|
|
#ifdef LIBXML_REGEXP_ENABLED
|
|
xmlValidBuildContentModel(NULL, NULL);
|
|
xmlValidatePopElement(NULL, NULL, NULL, NULL);
|
|
xmlValidatePushCData(NULL, NULL, 0);
|
|
xmlValidatePushElement(NULL, NULL, NULL, NULL);
|
|
#endif /* LIBXML_REGEXP_ENABLED */
|
|
#endif /* LIBXML_VALID_ENABLED */
|
|
|
|
#ifdef LIBXML_WRITER_ENABLED
|
|
xmlFreeTextWriter(NULL);
|
|
xmlFreeTextWriter(xmlNewTextWriter(NULL));
|
|
xmlFreeTextWriter(xmlNewTextWriterDoc(NULL, 0));
|
|
xmlFreeTextWriter(xmlNewTextWriterFilename(NULL, 0));
|
|
xmlFreeTextWriter(xmlNewTextWriterMemory(NULL, 0));
|
|
xmlFreeTextWriter(xmlNewTextWriterPushParser(NULL, 0));
|
|
xmlFreeTextWriter(xmlNewTextWriterTree(NULL, NULL, 0));
|
|
xmlTextWriterClose(NULL);
|
|
xmlTextWriterEndAttribute(NULL);
|
|
xmlTextWriterEndCDATA(NULL);
|
|
xmlTextWriterEndComment(NULL);
|
|
xmlTextWriterEndDTD(NULL);
|
|
xmlTextWriterEndDTDAttlist(NULL);
|
|
xmlTextWriterEndDTDElement(NULL);
|
|
xmlTextWriterEndDTDEntity(NULL);
|
|
xmlTextWriterEndDocument(NULL);
|
|
xmlTextWriterEndElement(NULL);
|
|
xmlTextWriterEndPI(NULL);
|
|
xmlTextWriterFlush(NULL);
|
|
xmlTextWriterFullEndElement(NULL);
|
|
xmlTextWriterSetIndent(NULL, 0);
|
|
xmlTextWriterSetIndentString(NULL, NULL);
|
|
xmlTextWriterSetQuoteChar(NULL, 0);
|
|
xmlTextWriterStartAttribute(NULL, NULL);
|
|
xmlTextWriterStartAttributeNS(NULL, NULL, NULL, NULL);
|
|
xmlTextWriterStartCDATA(NULL);
|
|
xmlTextWriterStartComment(NULL);
|
|
xmlTextWriterStartDTD(NULL, NULL, NULL, NULL);
|
|
xmlTextWriterStartDTDAttlist(NULL, NULL);
|
|
xmlTextWriterStartDTDElement(NULL, NULL);
|
|
xmlTextWriterStartDTDEntity(NULL, 0, NULL);
|
|
xmlTextWriterStartDocument(NULL, NULL, NULL, NULL);
|
|
xmlTextWriterStartElement(NULL, NULL);
|
|
xmlTextWriterStartElementNS(NULL, NULL, NULL, NULL);
|
|
xmlTextWriterStartPI(NULL, NULL);
|
|
xmlTextWriterWriteAttribute(NULL, NULL, NULL);
|
|
xmlTextWriterWriteAttributeNS(NULL, NULL, NULL, NULL, NULL);
|
|
xmlTextWriterWriteBase64(NULL, NULL, 0, 0);
|
|
xmlTextWriterWriteBinHex(NULL, NULL, 0, 0);
|
|
xmlTextWriterWriteCDATA(NULL, NULL);
|
|
xmlTextWriterWriteComment(NULL, NULL);
|
|
xmlTextWriterWriteDTD(NULL, NULL, NULL, NULL, NULL);
|
|
xmlTextWriterWriteDTDAttlist(NULL, NULL, NULL);
|
|
xmlTextWriterWriteDTDElement(NULL, NULL, NULL);
|
|
xmlTextWriterWriteDTDEntity(NULL, 0, NULL, NULL, NULL, NULL, NULL);
|
|
xmlTextWriterWriteDTDExternalEntity(NULL, 0, NULL, NULL, NULL, NULL);
|
|
xmlTextWriterWriteDTDExternalEntityContents(NULL, NULL, NULL, NULL);
|
|
xmlTextWriterWriteDTDInternalEntity(NULL, 0, NULL, NULL);
|
|
xmlTextWriterWriteDTDNotation(NULL, NULL, NULL, NULL);
|
|
xmlTextWriterWriteElement(NULL, NULL, NULL);
|
|
xmlTextWriterWriteElementNS(NULL, NULL, NULL, NULL, NULL);
|
|
xmlTextWriterWriteFormatAttribute(NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteFormatAttributeNS(NULL, NULL, NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteFormatCDATA(NULL, NULL, 0);
|
|
xmlTextWriterWriteFormatComment(NULL, NULL, 0);
|
|
xmlTextWriterWriteFormatDTD(NULL, NULL, NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteFormatDTDAttlist(NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteFormatDTDElement(NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteFormatDTDInternalEntity(NULL, 0, NULL, NULL, 0);
|
|
xmlTextWriterWriteFormatElement(NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteFormatElementNS(NULL, NULL, NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteFormatPI(NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteFormatRaw(NULL, NULL, 0);
|
|
xmlTextWriterWriteFormatString(NULL, NULL, 0);
|
|
xmlTextWriterWritePI(NULL, NULL, NULL);
|
|
xmlTextWriterWriteRaw(NULL, NULL);
|
|
xmlTextWriterWriteRawLen(NULL, NULL, 0);
|
|
xmlTextWriterWriteString(NULL, NULL);
|
|
xmlTextWriterWriteVFormatAttribute(NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteVFormatAttributeNS(NULL, NULL, NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteVFormatCDATA(NULL, NULL, 0);
|
|
xmlTextWriterWriteVFormatComment(NULL, NULL, 0);
|
|
xmlTextWriterWriteVFormatDTD(NULL, NULL, NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteVFormatDTDAttlist(NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteVFormatDTDElement(NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteVFormatDTDInternalEntity(NULL, 0, NULL, NULL, 0);
|
|
xmlTextWriterWriteVFormatElement(NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteVFormatElementNS(NULL, NULL, NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteVFormatPI(NULL, NULL, NULL, 0);
|
|
xmlTextWriterWriteVFormatRaw(NULL, NULL, 0);
|
|
xmlTextWriterWriteVFormatString(NULL, NULL, 0);
|
|
#endif /* LIBXML_WRITER_ENABLED */
|
|
|
|
#ifdef LIBXML_XINCLUDE_ENABLED
|
|
xmlXIncludeFreeContext(NULL);
|
|
xmlXIncludeGetLastError(NULL);
|
|
xmlXIncludeNewContext(NULL);
|
|
xmlXIncludeProcess(NULL);
|
|
xmlXIncludeProcessFlags(NULL, 0);
|
|
xmlXIncludeProcessFlagsData(NULL, 0, NULL);
|
|
xmlXIncludeProcessNode(NULL, NULL);
|
|
xmlXIncludeProcessTree(NULL);
|
|
xmlXIncludeProcessTreeFlags(NULL, 0);
|
|
xmlXIncludeProcessTreeFlagsData(NULL, 0, NULL);
|
|
xmlXIncludeSetErrorHandler(NULL, 0, NULL);
|
|
xmlXIncludeSetFlags(NULL, 0);
|
|
xmlXIncludeSetResourceLoader(NULL, 0, NULL);
|
|
#endif /* LIBXML_XINCLUDE_ENABLED */
|
|
|
|
#ifdef LIBXML_XPATH_ENABLED
|
|
xmlXPathAddValues(NULL);
|
|
xmlXPathBooleanFunction(NULL, 0);
|
|
xmlXPathCastBooleanToNumber(0);
|
|
xmlFree(xmlXPathCastBooleanToString(0));
|
|
xmlXPathCastNodeSetToBoolean(NULL);
|
|
xmlXPathCastNodeSetToNumber(NULL);
|
|
xmlFree(xmlXPathCastNodeSetToString(NULL));
|
|
xmlXPathCastNodeToNumber(NULL);
|
|
xmlFree(xmlXPathCastNodeToString(NULL));
|
|
xmlXPathCastNumberToBoolean(0);
|
|
xmlFree(xmlXPathCastNumberToString(0));
|
|
xmlXPathCastStringToBoolean(NULL);
|
|
xmlXPathCastStringToNumber(NULL);
|
|
xmlXPathCastToBoolean(NULL);
|
|
xmlXPathCastToNumber(NULL);
|
|
xmlFree(xmlXPathCastToString(NULL));
|
|
xmlXPathCeilingFunction(NULL, 0);
|
|
xmlXPathCmpNodes(NULL, NULL);
|
|
xmlXPathCompareValues(NULL, 0, 0);
|
|
xmlXPathCompile(NULL);
|
|
xmlXPathFreeObject(xmlXPathCompiledEval(NULL, NULL));
|
|
xmlXPathCompiledEvalToBoolean(NULL, NULL);
|
|
xmlXPathConcatFunction(NULL, 0);
|
|
xmlXPathContainsFunction(NULL, 0);
|
|
xmlXPathContextSetCache(NULL, 0, 0, 0);
|
|
xmlXPathFreeObject(xmlXPathConvertBoolean(NULL));
|
|
xmlXPathFreeObject(xmlXPathConvertNumber(NULL));
|
|
xmlXPathFreeObject(xmlXPathConvertString(NULL));
|
|
xmlXPathCountFunction(NULL, 0);
|
|
xmlXPathCtxtCompile(NULL, NULL);
|
|
xmlXPathFreeNodeSet(xmlXPathDifference(NULL, NULL));
|
|
xmlXPathFreeNodeSet(xmlXPathDistinct(NULL));
|
|
xmlXPathFreeNodeSet(xmlXPathDistinctSorted(NULL));
|
|
xmlXPathDivValues(NULL);
|
|
xmlXPathEqualValues(NULL);
|
|
xmlXPathErr(NULL, 0);
|
|
xmlXPathFreeObject(xmlXPathEval(NULL, NULL));
|
|
xmlXPathEvalExpr(NULL);
|
|
xmlXPathFreeObject(xmlXPathEvalExpression(NULL, NULL));
|
|
xmlXPathEvalPredicate(NULL, NULL);
|
|
xmlXPathEvaluatePredicateResult(NULL, NULL);
|
|
xmlXPathFalseFunction(NULL, 0);
|
|
xmlXPathFloorFunction(NULL, 0);
|
|
xmlXPathFreeCompExpr(NULL);
|
|
xmlXPathFreeContext(NULL);
|
|
xmlXPathFreeNodeSet(NULL);
|
|
xmlXPathFreeNodeSetList(NULL);
|
|
xmlXPathFreeObject(NULL);
|
|
xmlXPathFreeParserContext(NULL);
|
|
xmlXPathFunctionLookup(NULL, NULL);
|
|
xmlXPathFunctionLookupNS(NULL, NULL, NULL);
|
|
xmlXPathHasSameNodes(NULL, NULL);
|
|
xmlXPathIdFunction(NULL, 0);
|
|
xmlXPathInit();
|
|
xmlXPathFreeNodeSet(xmlXPathIntersection(NULL, NULL));
|
|
xmlXPathIsInf(0);
|
|
xmlXPathIsNaN(0);
|
|
xmlXPathIsNodeType(NULL);
|
|
xmlXPathLangFunction(NULL, 0);
|
|
xmlXPathLastFunction(NULL, 0);
|
|
xmlXPathFreeNodeSet(xmlXPathLeading(NULL, NULL));
|
|
xmlXPathFreeNodeSet(xmlXPathLeadingSorted(NULL, NULL));
|
|
xmlXPathLocalNameFunction(NULL, 0);
|
|
xmlXPathModValues(NULL);
|
|
xmlXPathMultValues(NULL);
|
|
xmlXPathNamespaceURIFunction(NULL, 0);
|
|
xmlXPathFreeObject(xmlXPathNewBoolean(0));
|
|
xmlXPathFreeObject(xmlXPathNewCString(NULL));
|
|
xmlXPathFreeContext(xmlXPathNewContext(NULL));
|
|
xmlXPathFreeObject(xmlXPathNewFloat(0));
|
|
xmlXPathFreeObject(xmlXPathNewNodeSet(NULL));
|
|
xmlXPathFreeObject(xmlXPathNewNodeSetList(NULL));
|
|
xmlXPathFreeParserContext(xmlXPathNewParserContext(NULL, NULL));
|
|
xmlXPathFreeObject(xmlXPathNewString(NULL));
|
|
xmlXPathFreeObject(xmlXPathNewValueTree(NULL));
|
|
xmlFreeNode(xmlXPathNextAncestor(NULL, NULL));
|
|
xmlFreeNode(xmlXPathNextAncestorOrSelf(NULL, NULL));
|
|
xmlFreeNode(xmlXPathNextAttribute(NULL, NULL));
|
|
xmlFreeNode(xmlXPathNextChild(NULL, NULL));
|
|
xmlFreeNode(xmlXPathNextDescendant(NULL, NULL));
|
|
xmlFreeNode(xmlXPathNextDescendantOrSelf(NULL, NULL));
|
|
xmlFreeNode(xmlXPathNextFollowing(NULL, NULL));
|
|
xmlFreeNode(xmlXPathNextFollowingSibling(NULL, NULL));
|
|
xmlFreeNode(xmlXPathNextNamespace(NULL, NULL));
|
|
xmlFreeNode(xmlXPathNextParent(NULL, NULL));
|
|
xmlFreeNode(xmlXPathNextPreceding(NULL, NULL));
|
|
xmlFreeNode(xmlXPathNextPrecedingSibling(NULL, NULL));
|
|
xmlFreeNode(xmlXPathNextSelf(NULL, NULL));
|
|
xmlXPathFreeObject(xmlXPathNodeEval(NULL, NULL, NULL));
|
|
xmlXPathFreeNodeSet(xmlXPathNodeLeading(NULL, NULL));
|
|
xmlXPathFreeNodeSet(xmlXPathNodeLeadingSorted(NULL, NULL));
|
|
xmlXPathNodeSetAdd(NULL, NULL);
|
|
xmlXPathNodeSetAddNs(NULL, NULL, NULL);
|
|
xmlXPathNodeSetAddUnique(NULL, NULL);
|
|
xmlXPathNodeSetContains(NULL, NULL);
|
|
xmlXPathFreeNodeSet(xmlXPathNodeSetCreate(NULL));
|
|
xmlXPathNodeSetDel(NULL, NULL);
|
|
xmlXPathNodeSetFreeNs(NULL);
|
|
xmlXPathFreeNodeSet(xmlXPathNodeSetMerge(NULL, NULL));
|
|
xmlXPathNodeSetRemove(NULL, 0);
|
|
xmlXPathNodeSetSort(NULL);
|
|
xmlXPathFreeNodeSet(xmlXPathNodeTrailing(NULL, NULL));
|
|
xmlXPathFreeNodeSet(xmlXPathNodeTrailingSorted(NULL, NULL));
|
|
xmlXPathNormalizeFunction(NULL, 0);
|
|
xmlXPathNotEqualValues(NULL);
|
|
xmlXPathNotFunction(NULL, 0);
|
|
xmlXPathNsLookup(NULL, NULL);
|
|
xmlXPathNumberFunction(NULL, 0);
|
|
xmlXPathFreeObject(xmlXPathObjectCopy(NULL));
|
|
xmlXPathOrderDocElems(NULL);
|
|
xmlFree(xmlXPathParseNCName(NULL));
|
|
xmlFree(xmlXPathParseName(NULL));
|
|
xmlXPathPopBoolean(NULL);
|
|
xmlXPathPopExternal(NULL);
|
|
xmlXPathFreeNodeSet(xmlXPathPopNodeSet(NULL));
|
|
xmlXPathPopNumber(NULL);
|
|
xmlFree(xmlXPathPopString(NULL));
|
|
xmlXPathPositionFunction(NULL, 0);
|
|
xmlXPathRegisterAllFunctions(NULL);
|
|
xmlXPathRegisterFunc(NULL, NULL, 0);
|
|
xmlXPathRegisterFuncLookup(NULL, 0, NULL);
|
|
xmlXPathRegisterFuncNS(NULL, NULL, NULL, 0);
|
|
xmlXPathRegisterNs(NULL, NULL, NULL);
|
|
xmlXPathRegisterVariable(NULL, NULL, NULL);
|
|
xmlXPathRegisterVariableLookup(NULL, 0, NULL);
|
|
xmlXPathRegisterVariableNS(NULL, NULL, NULL, NULL);
|
|
xmlXPathRegisteredFuncsCleanup(NULL);
|
|
xmlXPathRegisteredNsCleanup(NULL);
|
|
xmlXPathRegisteredVariablesCleanup(NULL);
|
|
xmlXPathRoot(NULL);
|
|
xmlXPathRoundFunction(NULL, 0);
|
|
xmlXPathSetContextNode(NULL, NULL);
|
|
xmlXPathSetErrorHandler(NULL, 0, NULL);
|
|
xmlXPathStartsWithFunction(NULL, 0);
|
|
xmlXPathStringEvalNumber(NULL);
|
|
xmlXPathStringFunction(NULL, 0);
|
|
xmlXPathStringLengthFunction(NULL, 0);
|
|
xmlXPathSubValues(NULL);
|
|
xmlXPathSubstringAfterFunction(NULL, 0);
|
|
xmlXPathSubstringBeforeFunction(NULL, 0);
|
|
xmlXPathSubstringFunction(NULL, 0);
|
|
xmlXPathSumFunction(NULL, 0);
|
|
xmlXPathFreeNodeSet(xmlXPathTrailing(NULL, NULL));
|
|
xmlXPathFreeNodeSet(xmlXPathTrailingSorted(NULL, NULL));
|
|
xmlXPathTranslateFunction(NULL, 0);
|
|
xmlXPathTrueFunction(NULL, 0);
|
|
xmlXPathValueFlipSign(NULL);
|
|
xmlXPathFreeObject(xmlXPathValuePop(NULL));
|
|
xmlXPathValuePush(NULL, NULL);
|
|
xmlXPathFreeObject(xmlXPathVariableLookup(NULL, NULL));
|
|
xmlXPathFreeObject(xmlXPathVariableLookupNS(NULL, NULL, NULL));
|
|
xmlXPathFreeObject(xmlXPathWrapCString(NULL));
|
|
xmlXPathFreeObject(xmlXPathWrapExternal(NULL));
|
|
xmlXPathFreeObject(xmlXPathWrapNodeSet(NULL));
|
|
xmlXPathFreeObject(xmlXPathWrapString(NULL));
|
|
xmlXPatherror(NULL, NULL, 0, 0);
|
|
#ifdef LIBXML_DEBUG_ENABLED
|
|
xmlXPathDebugDumpCompExpr(NULL, NULL, 0);
|
|
xmlXPathDebugDumpObject(NULL, NULL, 0);
|
|
#endif /* LIBXML_DEBUG_ENABLED */
|
|
#endif /* LIBXML_XPATH_ENABLED */
|
|
|
|
#ifdef LIBXML_XPTR_ENABLED
|
|
xlinkGetDefaultDetect();
|
|
xlinkGetDefaultHandler();
|
|
xlinkIsLink(NULL, NULL);
|
|
xlinkSetDefaultDetect(0);
|
|
xlinkSetDefaultHandler(NULL);
|
|
xmlXPathFreeObject(xmlXPtrEval(NULL, NULL));
|
|
xmlXPathFreeContext(xmlXPtrNewContext(NULL, NULL, NULL));
|
|
#endif /* LIBXML_XPTR_ENABLED */
|
|
|
|
xmlCleanupParser();
|
|
return 0;
|
|
}
|