mirror of
https://github.com/Kitware/CMake.git
synced 2025-05-09 23:08:18 +08:00
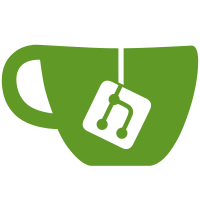
Add support for the "format" property of the Debug Adapter Protocol StackTrace request to fulfill the host's request to format the resulting StackFrame name differently.
41 lines
1.1 KiB
C++
41 lines
1.1 KiB
C++
/* Distributed under the OSI-approved BSD 3-Clause License. See accompanying
|
|
file Copyright.txt or https://cmake.org/licensing for details. */
|
|
#pragma once
|
|
|
|
#include "cmConfigure.h" // IWYU pragma: keep
|
|
|
|
#include <atomic>
|
|
#include <cstdint>
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
class cmListFileFunction;
|
|
struct cmListFileArgument;
|
|
class cmMakefile;
|
|
|
|
namespace cmDebugger {
|
|
|
|
class cmDebuggerStackFrame
|
|
{
|
|
static std::atomic<std::int64_t> NextId;
|
|
std::int64_t Id;
|
|
std::string FileName;
|
|
cmListFileFunction const& Function;
|
|
cmMakefile* Makefile;
|
|
|
|
public:
|
|
cmDebuggerStackFrame(cmMakefile* mf, std::string sourcePath,
|
|
cmListFileFunction const& lff);
|
|
int64_t GetId() const noexcept { return this->Id; }
|
|
std::string const& GetFileName() const noexcept { return this->FileName; }
|
|
int64_t GetLine() const noexcept;
|
|
cmMakefile* GetMakefile() const noexcept { return this->Makefile; }
|
|
cmListFileFunction const& GetFunction() const noexcept
|
|
{
|
|
return this->Function;
|
|
}
|
|
std::vector<cmListFileArgument> const& GetArguments() const noexcept;
|
|
};
|
|
|
|
} // namespace cmDebugger
|