mirror of
https://github.com/grub4dos/ntloader.git
synced 2025-05-09 20:21:08 +08:00
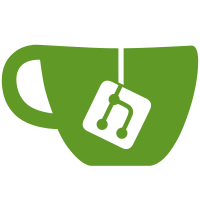
* import wimboot v28 * remove -Wno-array-bounds * addd * reg * cmline * charset * update bcd.bat * reg prefix * fix * bcd * rootfs * fsuuid * vdisk * partmap * biosdisk * efidisk * efi * fix * fix * fix reg * fix bcd * bcd * initrd * payload
60 lines
1.4 KiB
C
60 lines
1.4 KiB
C
#ifndef _ROTATE_H
|
|
#define _ROTATE_H
|
|
|
|
/** @file
|
|
*
|
|
* Bit operations
|
|
*/
|
|
|
|
#include <stdint.h>
|
|
|
|
static inline __attribute__ ((always_inline)) uint8_t
|
|
rol8 (uint8_t data, unsigned int rotation)
|
|
{
|
|
return ((data << rotation) | (data >> (8 - rotation)));
|
|
}
|
|
|
|
static inline __attribute__ ((always_inline)) uint8_t
|
|
ror8 (uint8_t data, unsigned int rotation)
|
|
{
|
|
return ((data >> rotation) | (data << (8 - rotation)));
|
|
}
|
|
|
|
static inline __attribute__ ((always_inline)) uint16_t
|
|
rol16 (uint16_t data, unsigned int rotation)
|
|
{
|
|
return ((data << rotation) | (data >> (16 - rotation)));
|
|
}
|
|
|
|
static inline __attribute__ ((always_inline)) uint16_t
|
|
ror16 (uint16_t data, unsigned int rotation)
|
|
{
|
|
return ((data >> rotation) | (data << (16 - rotation)));
|
|
}
|
|
|
|
static inline __attribute__ ((always_inline)) uint32_t
|
|
rol32 (uint32_t data, unsigned int rotation)
|
|
{
|
|
return ((data << rotation) | (data >> (32 - rotation)));
|
|
}
|
|
|
|
static inline __attribute__ ((always_inline)) uint32_t
|
|
ror32 (uint32_t data, unsigned int rotation)
|
|
{
|
|
return ((data >> rotation) | (data << (32 - rotation)));
|
|
}
|
|
|
|
static inline __attribute__ ((always_inline)) uint64_t
|
|
rol64 (uint64_t data, unsigned int rotation)
|
|
{
|
|
return ((data << rotation) | (data >> (64 - rotation)));
|
|
}
|
|
|
|
static inline __attribute__ ((always_inline)) uint64_t
|
|
ror64 (uint64_t data, unsigned int rotation)
|
|
{
|
|
return ((data >> rotation) | (data << (64 - rotation)));
|
|
}
|
|
|
|
#endif /* _ROTATE_H */
|