mirror of
https://github.com/riscv/riscv-opcodes.git
synced 2025-05-08 10:45:47 +08:00
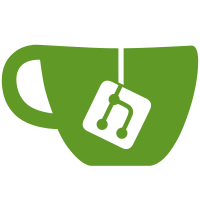
* Remove wildcard imports Use explicit imports rather than wildcards. This is more maintainable. * Enable Pylint in CI and fix its errors The main fixes were: * Specify encoding for all file opens. By default it depends on environment variables which is bad. * Use `with` to open files. Otherwise they don't necessarily get closed. There were also a few minor things like using `enumerate`, not using objects as default arguments, etc. In some cases I slightly refactored the code.
31 lines
836 B
Python
31 lines
836 B
Python
import logging
|
|
import pprint
|
|
from pathlib import Path
|
|
|
|
from constants import csrs, csrs32
|
|
from shared_utils import InstrDict
|
|
|
|
pp = pprint.PrettyPrinter(indent=2)
|
|
logging.basicConfig(level=logging.INFO, format="%(levelname)s:: %(message)s")
|
|
|
|
|
|
def make_sverilog(instr_dict: InstrDict):
|
|
names_str = ""
|
|
for i in instr_dict:
|
|
names_str += f" localparam [31:0] {i.upper().replace('.','_'):<18s} = 32'b{instr_dict[i]['encoding'].replace('-','?')};\n"
|
|
names_str += " /* CSR Addresses */\n"
|
|
for num, name in csrs + csrs32:
|
|
names_str += (
|
|
f" localparam logic [11:0] CSR_{name.upper()} = 12'h{hex(num)[2:]};\n"
|
|
)
|
|
|
|
Path("inst.sverilog").write_text(
|
|
f"""
|
|
/* Automatically generated by parse_opcodes */
|
|
package riscv_instr;
|
|
{names_str}
|
|
endpackage
|
|
""",
|
|
encoding="utf-8",
|
|
)
|