mirror of
https://git.rtems.org/rtems-source-builder
synced 2024-10-09 07:15:10 +08:00
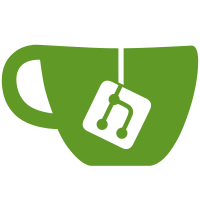
The RSB documentation is now in ReST format and part of the RTEMS Documentation project. See https://docs.rtems.org/. Remove support for the GPL based asciidoc tool and remove the asciidoc package from the RSB. Add the Python Markdown package and update the reporter to use Markdown for HTML generation. The resuling HTML report is a single self contained file. Closes #3047.
92 lines
2.7 KiB
Python
92 lines
2.7 KiB
Python
'''
|
|
Abbreviation Extension for Python-Markdown
|
|
==========================================
|
|
|
|
This extension adds abbreviation handling to Python-Markdown.
|
|
|
|
See <https://pythonhosted.org/Markdown/extensions/abbreviations.html>
|
|
for documentation.
|
|
|
|
Oringinal code Copyright 2007-2008 [Waylan Limberg](http://achinghead.com/) and
|
|
[Seemant Kulleen](http://www.kulleen.org/)
|
|
|
|
All changes Copyright 2008-2014 The Python Markdown Project
|
|
|
|
License: [BSD](http://www.opensource.org/licenses/bsd-license.php)
|
|
|
|
'''
|
|
|
|
from __future__ import absolute_import
|
|
from __future__ import unicode_literals
|
|
from . import Extension
|
|
from ..preprocessors import Preprocessor
|
|
from ..inlinepatterns import Pattern
|
|
from ..util import etree, AtomicString
|
|
import re
|
|
|
|
# Global Vars
|
|
ABBR_REF_RE = re.compile(r'[*]\[(?P<abbr>[^\]]*)\][ ]?:\s*(?P<title>.*)')
|
|
|
|
|
|
class AbbrExtension(Extension):
|
|
""" Abbreviation Extension for Python-Markdown. """
|
|
|
|
def extendMarkdown(self, md, md_globals):
|
|
""" Insert AbbrPreprocessor before ReferencePreprocessor. """
|
|
md.preprocessors.add('abbr', AbbrPreprocessor(md), '<reference')
|
|
|
|
|
|
class AbbrPreprocessor(Preprocessor):
|
|
""" Abbreviation Preprocessor - parse text for abbr references. """
|
|
|
|
def run(self, lines):
|
|
'''
|
|
Find and remove all Abbreviation references from the text.
|
|
Each reference is set as a new AbbrPattern in the markdown instance.
|
|
|
|
'''
|
|
new_text = []
|
|
for line in lines:
|
|
m = ABBR_REF_RE.match(line)
|
|
if m:
|
|
abbr = m.group('abbr').strip()
|
|
title = m.group('title').strip()
|
|
self.markdown.inlinePatterns['abbr-%s' % abbr] = \
|
|
AbbrPattern(self._generate_pattern(abbr), title)
|
|
else:
|
|
new_text.append(line)
|
|
return new_text
|
|
|
|
def _generate_pattern(self, text):
|
|
'''
|
|
Given a string, returns an regex pattern to match that string.
|
|
|
|
'HTML' -> r'(?P<abbr>[H][T][M][L])'
|
|
|
|
Note: we force each char as a literal match (in brackets) as we don't
|
|
know what they will be beforehand.
|
|
|
|
'''
|
|
chars = list(text)
|
|
for i in range(len(chars)):
|
|
chars[i] = r'[%s]' % chars[i]
|
|
return r'(?P<abbr>\b%s\b)' % (r''.join(chars))
|
|
|
|
|
|
class AbbrPattern(Pattern):
|
|
""" Abbreviation inline pattern. """
|
|
|
|
def __init__(self, pattern, title):
|
|
super(AbbrPattern, self).__init__(pattern)
|
|
self.title = title
|
|
|
|
def handleMatch(self, m):
|
|
abbr = etree.Element('abbr')
|
|
abbr.text = AtomicString(m.group('abbr'))
|
|
abbr.set('title', self.title)
|
|
return abbr
|
|
|
|
|
|
def makeExtension(*args, **kwargs):
|
|
return AbbrExtension(*args, **kwargs)
|