mirror of
https://git.yoctoproject.org/poky-contrib
synced 2025-05-08 23:52:25 +08:00
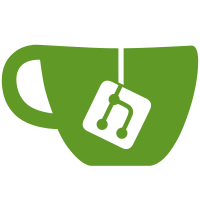
The 3.0.1 release of SPDX has been officially released with a few minor modifications. Regenerate the bindings to use this version. (From OE-Core rev: 54233a7d6fe414d22449fb02fac26b66a820b17a) Signed-off-by: Joshua Watt <JPEWhacker@gmail.com> Signed-off-by: Mathieu Dubois-Briand <mathieu.dubois-briand@bootlin.com> Signed-off-by: Richard Purdie <richard.purdie@linuxfoundation.org>
5594 lines
258 KiB
Python
5594 lines
258 KiB
Python
#! /usr/bin/env python3
|
|
#
|
|
# Generated Python bindings from a SHACL model
|
|
#
|
|
# This file was automatically generated by shacl2code. DO NOT MANUALLY MODIFY IT
|
|
#
|
|
# SPDX-License-Identifier: MIT
|
|
|
|
import functools
|
|
import hashlib
|
|
import json
|
|
import re
|
|
import sys
|
|
import threading
|
|
import time
|
|
from contextlib import contextmanager
|
|
from datetime import datetime, timezone, timedelta
|
|
from enum import Enum
|
|
from abc import ABC, abstractmethod
|
|
|
|
|
|
def check_type(obj, types):
|
|
if not isinstance(obj, types):
|
|
if isinstance(types, (list, tuple)):
|
|
raise TypeError(
|
|
f"Value must be one of type: {', '.join(t.__name__ for t in types)}. Got {type(obj)}"
|
|
)
|
|
raise TypeError(f"Value must be of type {types.__name__}. Got {type(obj)}")
|
|
|
|
|
|
class Property(ABC):
|
|
"""
|
|
A generic SHACL object property. The different types will derive from this
|
|
class
|
|
"""
|
|
|
|
def __init__(self, *, pattern=None):
|
|
self.pattern = pattern
|
|
|
|
def init(self):
|
|
return None
|
|
|
|
def validate(self, value):
|
|
check_type(value, self.VALID_TYPES)
|
|
if self.pattern is not None and not re.search(
|
|
self.pattern, self.to_string(value)
|
|
):
|
|
raise ValueError(
|
|
f"Value is not correctly formatted. Got '{self.to_string(value)}'"
|
|
)
|
|
|
|
def set(self, value):
|
|
return value
|
|
|
|
def check_min_count(self, value, min_count):
|
|
return min_count == 1
|
|
|
|
def check_max_count(self, value, max_count):
|
|
return max_count == 1
|
|
|
|
def elide(self, value):
|
|
return value is None
|
|
|
|
def walk(self, value, callback, path):
|
|
callback(value, path)
|
|
|
|
def iter_objects(self, value, recursive, visited):
|
|
return []
|
|
|
|
def link_prop(self, value, objectset, missing, visited):
|
|
return value
|
|
|
|
def to_string(self, value):
|
|
return str(value)
|
|
|
|
@abstractmethod
|
|
def encode(self, encoder, value, state):
|
|
pass
|
|
|
|
@abstractmethod
|
|
def decode(self, decoder, *, objectset=None):
|
|
pass
|
|
|
|
|
|
class StringProp(Property):
|
|
"""
|
|
A scalar string property for an SHACL object
|
|
"""
|
|
|
|
VALID_TYPES = str
|
|
|
|
def set(self, value):
|
|
return str(value)
|
|
|
|
def encode(self, encoder, value, state):
|
|
encoder.write_string(value)
|
|
|
|
def decode(self, decoder, *, objectset=None):
|
|
return decoder.read_string()
|
|
|
|
|
|
class AnyURIProp(StringProp):
|
|
def encode(self, encoder, value, state):
|
|
encoder.write_iri(value)
|
|
|
|
def decode(self, decoder, *, objectset=None):
|
|
return decoder.read_iri()
|
|
|
|
|
|
class DateTimeProp(Property):
|
|
"""
|
|
A Date/Time Object with optional timezone
|
|
"""
|
|
|
|
VALID_TYPES = datetime
|
|
UTC_FORMAT_STR = "%Y-%m-%dT%H:%M:%SZ"
|
|
REGEX = r"^\d{4}-\d{2}-\d{2}T\d{2}:\d{2}:\d{2}(Z|[+-]\d{2}:\d{2})?$"
|
|
|
|
def set(self, value):
|
|
return self._normalize(value)
|
|
|
|
def encode(self, encoder, value, state):
|
|
encoder.write_datetime(self.to_string(value))
|
|
|
|
def decode(self, decoder, *, objectset=None):
|
|
s = decoder.read_datetime()
|
|
if s is None:
|
|
return None
|
|
v = self.from_string(s)
|
|
return self._normalize(v)
|
|
|
|
def _normalize(self, value):
|
|
if value.utcoffset() is None:
|
|
value = value.astimezone()
|
|
offset = value.utcoffset()
|
|
seconds = offset % timedelta(minutes=-1 if offset.total_seconds() < 0 else 1)
|
|
if seconds:
|
|
offset = offset - seconds
|
|
value = value.replace(tzinfo=timezone(offset))
|
|
value = value.replace(microsecond=0)
|
|
return value
|
|
|
|
def to_string(self, value):
|
|
value = self._normalize(value)
|
|
if value.tzinfo == timezone.utc:
|
|
return value.strftime(self.UTC_FORMAT_STR)
|
|
return value.isoformat()
|
|
|
|
def from_string(self, value):
|
|
if not re.match(self.REGEX, value):
|
|
raise ValueError(f"'{value}' is not a correctly formatted datetime")
|
|
if "Z" in value:
|
|
d = datetime(
|
|
*(time.strptime(value, self.UTC_FORMAT_STR)[0:6]),
|
|
tzinfo=timezone.utc,
|
|
)
|
|
else:
|
|
d = datetime.fromisoformat(value)
|
|
|
|
return self._normalize(d)
|
|
|
|
|
|
class DateTimeStampProp(DateTimeProp):
|
|
"""
|
|
A Date/Time Object with required timestamp
|
|
"""
|
|
|
|
REGEX = r"^\d{4}-\d{2}-\d{2}T\d{2}:\d{2}:\d{2}(Z|[+-]\d{2}:\d{2})$"
|
|
|
|
|
|
class IntegerProp(Property):
|
|
VALID_TYPES = int
|
|
|
|
def set(self, value):
|
|
return int(value)
|
|
|
|
def encode(self, encoder, value, state):
|
|
encoder.write_integer(value)
|
|
|
|
def decode(self, decoder, *, objectset=None):
|
|
return decoder.read_integer()
|
|
|
|
|
|
class PositiveIntegerProp(IntegerProp):
|
|
def validate(self, value):
|
|
super().validate(value)
|
|
if value < 1:
|
|
raise ValueError(f"Value must be >=1. Got {value}")
|
|
|
|
|
|
class NonNegativeIntegerProp(IntegerProp):
|
|
def validate(self, value):
|
|
super().validate(value)
|
|
if value < 0:
|
|
raise ValueError(f"Value must be >= 0. Got {value}")
|
|
|
|
|
|
class BooleanProp(Property):
|
|
VALID_TYPES = bool
|
|
|
|
def set(self, value):
|
|
return bool(value)
|
|
|
|
def encode(self, encoder, value, state):
|
|
encoder.write_bool(value)
|
|
|
|
def decode(self, decoder, *, objectset=None):
|
|
return decoder.read_bool()
|
|
|
|
|
|
class FloatProp(Property):
|
|
VALID_TYPES = (float, int)
|
|
|
|
def set(self, value):
|
|
return float(value)
|
|
|
|
def encode(self, encoder, value, state):
|
|
encoder.write_float(value)
|
|
|
|
def decode(self, decoder, *, objectset=None):
|
|
return decoder.read_float()
|
|
|
|
|
|
class IRIProp(Property):
|
|
def __init__(self, context=[], *, pattern=None):
|
|
super().__init__(pattern=pattern)
|
|
self.context = context
|
|
|
|
def compact(self, value):
|
|
for iri, compact in self.context:
|
|
if value == iri:
|
|
return compact
|
|
return None
|
|
|
|
def expand(self, value):
|
|
for iri, compact in self.context:
|
|
if value == compact:
|
|
return iri
|
|
return None
|
|
|
|
def iri_values(self):
|
|
return (iri for iri, _ in self.context)
|
|
|
|
|
|
class ObjectProp(IRIProp):
|
|
"""
|
|
A scalar SHACL object property of a SHACL object
|
|
"""
|
|
|
|
def __init__(self, cls, required, context=[]):
|
|
super().__init__(context)
|
|
self.cls = cls
|
|
self.required = required
|
|
|
|
def init(self):
|
|
if self.required and not self.cls.IS_ABSTRACT:
|
|
return self.cls()
|
|
return None
|
|
|
|
def validate(self, value):
|
|
check_type(value, (self.cls, str))
|
|
|
|
def walk(self, value, callback, path):
|
|
if value is None:
|
|
return
|
|
|
|
if not isinstance(value, str):
|
|
value.walk(callback, path)
|
|
else:
|
|
callback(value, path)
|
|
|
|
def iter_objects(self, value, recursive, visited):
|
|
if value is None or isinstance(value, str):
|
|
return
|
|
|
|
if value not in visited:
|
|
visited.add(value)
|
|
yield value
|
|
|
|
if recursive:
|
|
for c in value.iter_objects(recursive=True, visited=visited):
|
|
yield c
|
|
|
|
def encode(self, encoder, value, state):
|
|
if value is None:
|
|
raise ValueError("Object cannot be None")
|
|
|
|
if isinstance(value, str):
|
|
encoder.write_iri(value, self.compact(value))
|
|
return
|
|
|
|
return value.encode(encoder, state)
|
|
|
|
def decode(self, decoder, *, objectset=None):
|
|
iri = decoder.read_iri()
|
|
if iri is None:
|
|
return self.cls.decode(decoder, objectset=objectset)
|
|
|
|
iri = self.expand(iri) or iri
|
|
|
|
if objectset is None:
|
|
return iri
|
|
|
|
obj = objectset.find_by_id(iri)
|
|
if obj is None:
|
|
return iri
|
|
|
|
self.validate(obj)
|
|
return obj
|
|
|
|
def link_prop(self, value, objectset, missing, visited):
|
|
if value is None:
|
|
return value
|
|
|
|
if isinstance(value, str):
|
|
o = objectset.find_by_id(value)
|
|
if o is not None:
|
|
self.validate(o)
|
|
return o
|
|
|
|
if missing is not None:
|
|
missing.add(value)
|
|
|
|
return value
|
|
|
|
# De-duplicate IDs
|
|
if value._id:
|
|
value = objectset.find_by_id(value._id, value)
|
|
self.validate(value)
|
|
|
|
value.link_helper(objectset, missing, visited)
|
|
return value
|
|
|
|
|
|
class ListProxy(object):
|
|
def __init__(self, prop, data=None):
|
|
if data is None:
|
|
self.__data = []
|
|
else:
|
|
self.__data = data
|
|
self.__prop = prop
|
|
|
|
def append(self, value):
|
|
self.__prop.validate(value)
|
|
self.__data.append(self.__prop.set(value))
|
|
|
|
def insert(self, idx, value):
|
|
self.__prop.validate(value)
|
|
self.__data.insert(idx, self.__prop.set(value))
|
|
|
|
def extend(self, items):
|
|
for i in items:
|
|
self.append(i)
|
|
|
|
def sort(self, *args, **kwargs):
|
|
self.__data.sort(*args, **kwargs)
|
|
|
|
def __getitem__(self, key):
|
|
return self.__data[key]
|
|
|
|
def __setitem__(self, key, value):
|
|
if isinstance(key, slice):
|
|
for v in value:
|
|
self.__prop.validate(v)
|
|
self.__data[key] = [self.__prop.set(v) for v in value]
|
|
else:
|
|
self.__prop.validate(value)
|
|
self.__data[key] = self.__prop.set(value)
|
|
|
|
def __delitem__(self, key):
|
|
del self.__data[key]
|
|
|
|
def __contains__(self, item):
|
|
return item in self.__data
|
|
|
|
def __iter__(self):
|
|
return iter(self.__data)
|
|
|
|
def __len__(self):
|
|
return len(self.__data)
|
|
|
|
def __str__(self):
|
|
return str(self.__data)
|
|
|
|
def __repr__(self):
|
|
return repr(self.__data)
|
|
|
|
def __eq__(self, other):
|
|
if isinstance(other, ListProxy):
|
|
return self.__data == other.__data
|
|
|
|
return self.__data == other
|
|
|
|
|
|
class ListProp(Property):
|
|
"""
|
|
A list of SHACL properties
|
|
"""
|
|
|
|
VALID_TYPES = (list, ListProxy)
|
|
|
|
def __init__(self, prop):
|
|
super().__init__()
|
|
self.prop = prop
|
|
|
|
def init(self):
|
|
return ListProxy(self.prop)
|
|
|
|
def validate(self, value):
|
|
super().validate(value)
|
|
|
|
for i in value:
|
|
self.prop.validate(i)
|
|
|
|
def set(self, value):
|
|
if isinstance(value, ListProxy):
|
|
return value
|
|
|
|
return ListProxy(self.prop, [self.prop.set(d) for d in value])
|
|
|
|
def check_min_count(self, value, min_count):
|
|
check_type(value, ListProxy)
|
|
return len(value) >= min_count
|
|
|
|
def check_max_count(self, value, max_count):
|
|
check_type(value, ListProxy)
|
|
return len(value) <= max_count
|
|
|
|
def elide(self, value):
|
|
check_type(value, ListProxy)
|
|
return len(value) == 0
|
|
|
|
def walk(self, value, callback, path):
|
|
callback(value, path)
|
|
for idx, v in enumerate(value):
|
|
self.prop.walk(v, callback, path + [f"[{idx}]"])
|
|
|
|
def iter_objects(self, value, recursive, visited):
|
|
for v in value:
|
|
for c in self.prop.iter_objects(v, recursive, visited):
|
|
yield c
|
|
|
|
def link_prop(self, value, objectset, missing, visited):
|
|
if isinstance(value, ListProxy):
|
|
data = [self.prop.link_prop(v, objectset, missing, visited) for v in value]
|
|
else:
|
|
data = [self.prop.link_prop(v, objectset, missing, visited) for v in value]
|
|
|
|
return ListProxy(self.prop, data=data)
|
|
|
|
def encode(self, encoder, value, state):
|
|
check_type(value, ListProxy)
|
|
|
|
with encoder.write_list() as list_s:
|
|
for v in value:
|
|
with list_s.write_list_item() as item_s:
|
|
self.prop.encode(item_s, v, state)
|
|
|
|
def decode(self, decoder, *, objectset=None):
|
|
data = []
|
|
for val_d in decoder.read_list():
|
|
v = self.prop.decode(val_d, objectset=objectset)
|
|
self.prop.validate(v)
|
|
data.append(v)
|
|
|
|
return ListProxy(self.prop, data=data)
|
|
|
|
|
|
class EnumProp(IRIProp):
|
|
VALID_TYPES = str
|
|
|
|
def __init__(self, values, *, pattern=None):
|
|
super().__init__(values, pattern=pattern)
|
|
|
|
def validate(self, value):
|
|
super().validate(value)
|
|
|
|
valid_values = self.iri_values()
|
|
if value not in valid_values:
|
|
raise ValueError(
|
|
f"'{value}' is not a valid value. Choose one of {' '.join(valid_values)}"
|
|
)
|
|
|
|
def encode(self, encoder, value, state):
|
|
encoder.write_enum(value, self, self.compact(value))
|
|
|
|
def decode(self, decoder, *, objectset=None):
|
|
v = decoder.read_enum(self)
|
|
return self.expand(v) or v
|
|
|
|
|
|
class NodeKind(Enum):
|
|
BlankNode = 1
|
|
IRI = 2
|
|
BlankNodeOrIRI = 3
|
|
|
|
|
|
def is_IRI(s):
|
|
if not isinstance(s, str):
|
|
return False
|
|
if s.startswith("_:"):
|
|
return False
|
|
if ":" not in s:
|
|
return False
|
|
return True
|
|
|
|
|
|
def is_blank_node(s):
|
|
if not isinstance(s, str):
|
|
return False
|
|
if not s.startswith("_:"):
|
|
return False
|
|
return True
|
|
|
|
|
|
def register(type_iri, *, compact_type=None, abstract=False):
|
|
def add_class(key, c):
|
|
assert (
|
|
key not in SHACLObject.CLASSES
|
|
), f"{key} already registered to {SHACLObject.CLASSES[key].__name__}"
|
|
SHACLObject.CLASSES[key] = c
|
|
|
|
def decorator(c):
|
|
global NAMED_INDIVIDUALS
|
|
|
|
assert issubclass(
|
|
c, SHACLObject
|
|
), f"{c.__name__} is not derived from SHACLObject"
|
|
|
|
c._OBJ_TYPE = type_iri
|
|
c.IS_ABSTRACT = abstract
|
|
add_class(type_iri, c)
|
|
|
|
c._OBJ_COMPACT_TYPE = compact_type
|
|
if compact_type:
|
|
add_class(compact_type, c)
|
|
|
|
NAMED_INDIVIDUALS |= set(c.NAMED_INDIVIDUALS.values())
|
|
|
|
# Registration is deferred until the first instance of class is created
|
|
# so that it has access to any other defined class
|
|
c._NEEDS_REG = True
|
|
return c
|
|
|
|
return decorator
|
|
|
|
|
|
register_lock = threading.Lock()
|
|
NAMED_INDIVIDUALS = set()
|
|
|
|
|
|
@functools.total_ordering
|
|
class SHACLObject(object):
|
|
CLASSES = {}
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
ID_ALIAS = None
|
|
IS_ABSTRACT = True
|
|
|
|
def __init__(self, **kwargs):
|
|
if self._is_abstract():
|
|
raise NotImplementedError(
|
|
f"{self.__class__.__name__} is abstract and cannot be implemented"
|
|
)
|
|
|
|
with register_lock:
|
|
cls = self.__class__
|
|
if cls._NEEDS_REG:
|
|
cls._OBJ_PROPERTIES = {}
|
|
cls._OBJ_IRIS = {}
|
|
cls._register_props()
|
|
cls._NEEDS_REG = False
|
|
|
|
self.__dict__["_obj_data"] = {}
|
|
self.__dict__["_obj_metadata"] = {}
|
|
|
|
for iri, prop, _, _, _, _ in self.__iter_props():
|
|
self.__dict__["_obj_data"][iri] = prop.init()
|
|
|
|
for k, v in kwargs.items():
|
|
setattr(self, k, v)
|
|
|
|
def _is_abstract(self):
|
|
return self.__class__.IS_ABSTRACT
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
cls._add_property("_id", StringProp(), iri="@id")
|
|
|
|
@classmethod
|
|
def _add_property(
|
|
cls,
|
|
pyname,
|
|
prop,
|
|
iri,
|
|
min_count=None,
|
|
max_count=None,
|
|
compact=None,
|
|
):
|
|
if pyname in cls._OBJ_IRIS:
|
|
raise KeyError(f"'{pyname}' is already defined for '{cls.__name__}'")
|
|
if iri in cls._OBJ_PROPERTIES:
|
|
raise KeyError(f"'{iri}' is already defined for '{cls.__name__}'")
|
|
|
|
while hasattr(cls, pyname):
|
|
pyname = pyname + "_"
|
|
|
|
pyname = sys.intern(pyname)
|
|
iri = sys.intern(iri)
|
|
|
|
cls._OBJ_IRIS[pyname] = iri
|
|
cls._OBJ_PROPERTIES[iri] = (prop, min_count, max_count, pyname, compact)
|
|
|
|
def __setattr__(self, name, value):
|
|
if name == self.ID_ALIAS:
|
|
self["@id"] = value
|
|
return
|
|
|
|
try:
|
|
iri = self._OBJ_IRIS[name]
|
|
self[iri] = value
|
|
except KeyError:
|
|
raise AttributeError(
|
|
f"'{name}' is not a valid property of {self.__class__.__name__}"
|
|
)
|
|
|
|
def __getattr__(self, name):
|
|
if name in self._OBJ_IRIS:
|
|
return self.__dict__["_obj_data"][self._OBJ_IRIS[name]]
|
|
|
|
if name == self.ID_ALIAS:
|
|
return self.__dict__["_obj_data"]["@id"]
|
|
|
|
if name == "_metadata":
|
|
return self.__dict__["_obj_metadata"]
|
|
|
|
if name == "_IRI":
|
|
return self._OBJ_IRIS
|
|
|
|
if name == "TYPE":
|
|
return self.__class__._OBJ_TYPE
|
|
|
|
if name == "COMPACT_TYPE":
|
|
return self.__class__._OBJ_COMPACT_TYPE
|
|
|
|
raise AttributeError(
|
|
f"'{name}' is not a valid property of {self.__class__.__name__}"
|
|
)
|
|
|
|
def __delattr__(self, name):
|
|
if name == self.ID_ALIAS:
|
|
del self["@id"]
|
|
return
|
|
|
|
try:
|
|
iri = self._OBJ_IRIS[name]
|
|
del self[iri]
|
|
except KeyError:
|
|
raise AttributeError(
|
|
f"'{name}' is not a valid property of {self.__class__.__name__}"
|
|
)
|
|
|
|
def __get_prop(self, iri):
|
|
if iri not in self._OBJ_PROPERTIES:
|
|
raise KeyError(
|
|
f"'{iri}' is not a valid property of {self.__class__.__name__}"
|
|
)
|
|
|
|
return self._OBJ_PROPERTIES[iri]
|
|
|
|
def __iter_props(self):
|
|
for iri, v in self._OBJ_PROPERTIES.items():
|
|
yield iri, *v
|
|
|
|
def __getitem__(self, iri):
|
|
return self.__dict__["_obj_data"][iri]
|
|
|
|
def __setitem__(self, iri, value):
|
|
if iri == "@id":
|
|
if self.NODE_KIND == NodeKind.BlankNode:
|
|
if not is_blank_node(value):
|
|
raise ValueError(
|
|
f"{self.__class__.__name__} ({id(self)}) can only have local reference. Property '{iri}' cannot be set to '{value}' and must start with '_:'"
|
|
)
|
|
elif self.NODE_KIND == NodeKind.IRI:
|
|
if not is_IRI(value):
|
|
raise ValueError(
|
|
f"{self.__class__.__name__} ({id(self)}) can only have an IRI value. Property '{iri}' cannot be set to '{value}'"
|
|
)
|
|
else:
|
|
if not is_blank_node(value) and not is_IRI(value):
|
|
raise ValueError(
|
|
f"{self.__class__.__name__} ({id(self)}) Has invalid Property '{iri}' '{value}'. Must be a blank node or IRI"
|
|
)
|
|
|
|
prop, _, _, _, _ = self.__get_prop(iri)
|
|
prop.validate(value)
|
|
self.__dict__["_obj_data"][iri] = prop.set(value)
|
|
|
|
def __delitem__(self, iri):
|
|
prop, _, _, _, _ = self.__get_prop(iri)
|
|
self.__dict__["_obj_data"][iri] = prop.init()
|
|
|
|
def __iter__(self):
|
|
return self._OBJ_PROPERTIES.keys()
|
|
|
|
def walk(self, callback, path=None):
|
|
"""
|
|
Walk object tree, invoking the callback for each item
|
|
|
|
Callback has the form:
|
|
|
|
def callback(object, path):
|
|
"""
|
|
if path is None:
|
|
path = ["."]
|
|
|
|
if callback(self, path):
|
|
for iri, prop, _, _, _, _ in self.__iter_props():
|
|
prop.walk(self.__dict__["_obj_data"][iri], callback, path + [f".{iri}"])
|
|
|
|
def property_keys(self):
|
|
for iri, _, _, _, pyname, compact in self.__iter_props():
|
|
if iri == "@id":
|
|
compact = self.ID_ALIAS
|
|
yield pyname, iri, compact
|
|
|
|
def iter_objects(self, *, recursive=False, visited=None):
|
|
"""
|
|
Iterate of all objects that are a child of this one
|
|
"""
|
|
if visited is None:
|
|
visited = set()
|
|
|
|
for iri, prop, _, _, _, _ in self.__iter_props():
|
|
for c in prop.iter_objects(
|
|
self.__dict__["_obj_data"][iri], recursive=recursive, visited=visited
|
|
):
|
|
yield c
|
|
|
|
def encode(self, encoder, state):
|
|
idname = self.ID_ALIAS or self._OBJ_IRIS["_id"]
|
|
if not self._id and self.NODE_KIND == NodeKind.IRI:
|
|
raise ValueError(
|
|
f"{self.__class__.__name__} ({id(self)}) must have a IRI for property '{idname}'"
|
|
)
|
|
|
|
if state.is_written(self):
|
|
encoder.write_iri(state.get_object_id(self))
|
|
return
|
|
|
|
state.add_written(self)
|
|
|
|
with encoder.write_object(
|
|
self,
|
|
state.get_object_id(self),
|
|
bool(self._id) or state.is_refed(self),
|
|
) as obj_s:
|
|
self._encode_properties(obj_s, state)
|
|
|
|
def _encode_properties(self, encoder, state):
|
|
for iri, prop, min_count, max_count, pyname, compact in self.__iter_props():
|
|
value = self.__dict__["_obj_data"][iri]
|
|
if prop.elide(value):
|
|
if min_count:
|
|
raise ValueError(
|
|
f"Property '{pyname}' in {self.__class__.__name__} ({id(self)}) is required (currently {value!r})"
|
|
)
|
|
continue
|
|
|
|
if min_count is not None:
|
|
if not prop.check_min_count(value, min_count):
|
|
raise ValueError(
|
|
f"Property '{pyname}' in {self.__class__.__name__} ({id(self)}) requires a minimum of {min_count} elements"
|
|
)
|
|
|
|
if max_count is not None:
|
|
if not prop.check_max_count(value, max_count):
|
|
raise ValueError(
|
|
f"Property '{pyname}' in {self.__class__.__name__} ({id(self)}) requires a maximum of {max_count} elements"
|
|
)
|
|
|
|
if iri == self._OBJ_IRIS["_id"]:
|
|
continue
|
|
|
|
with encoder.write_property(iri, compact) as prop_s:
|
|
prop.encode(prop_s, value, state)
|
|
|
|
@classmethod
|
|
def _make_object(cls, typ):
|
|
if typ not in cls.CLASSES:
|
|
raise TypeError(f"Unknown type {typ}")
|
|
|
|
return cls.CLASSES[typ]()
|
|
|
|
@classmethod
|
|
def decode(cls, decoder, *, objectset=None):
|
|
typ, obj_d = decoder.read_object()
|
|
if typ is None:
|
|
raise TypeError("Unable to determine type for object")
|
|
|
|
obj = cls._make_object(typ)
|
|
for key in (obj.ID_ALIAS, obj._OBJ_IRIS["_id"]):
|
|
with obj_d.read_property(key) as prop_d:
|
|
if prop_d is None:
|
|
continue
|
|
|
|
_id = prop_d.read_iri()
|
|
if _id is None:
|
|
raise TypeError(f"Object key '{key}' is the wrong type")
|
|
|
|
obj._id = _id
|
|
break
|
|
|
|
if obj.NODE_KIND == NodeKind.IRI and not obj._id:
|
|
raise ValueError("Object is missing required IRI")
|
|
|
|
if objectset is not None:
|
|
if obj._id:
|
|
v = objectset.find_by_id(_id)
|
|
if v is not None:
|
|
return v
|
|
|
|
obj._decode_properties(obj_d, objectset=objectset)
|
|
|
|
if objectset is not None:
|
|
objectset.add_index(obj)
|
|
return obj
|
|
|
|
def _decode_properties(self, decoder, objectset=None):
|
|
for key in decoder.object_keys():
|
|
if not self._decode_prop(decoder, key, objectset=objectset):
|
|
raise KeyError(f"Unknown property '{key}'")
|
|
|
|
def _decode_prop(self, decoder, key, objectset=None):
|
|
if key in (self._OBJ_IRIS["_id"], self.ID_ALIAS):
|
|
return True
|
|
|
|
for iri, prop, _, _, _, compact in self.__iter_props():
|
|
if compact == key:
|
|
read_key = compact
|
|
elif iri == key:
|
|
read_key = iri
|
|
else:
|
|
continue
|
|
|
|
with decoder.read_property(read_key) as prop_d:
|
|
v = prop.decode(prop_d, objectset=objectset)
|
|
prop.validate(v)
|
|
self.__dict__["_obj_data"][iri] = v
|
|
return True
|
|
|
|
return False
|
|
|
|
def link_helper(self, objectset, missing, visited):
|
|
if self in visited:
|
|
return
|
|
|
|
visited.add(self)
|
|
|
|
for iri, prop, _, _, _, _ in self.__iter_props():
|
|
self.__dict__["_obj_data"][iri] = prop.link_prop(
|
|
self.__dict__["_obj_data"][iri],
|
|
objectset,
|
|
missing,
|
|
visited,
|
|
)
|
|
|
|
def __str__(self):
|
|
parts = [
|
|
f"{self.__class__.__name__}(",
|
|
]
|
|
if self._id:
|
|
parts.append(f"@id='{self._id}'")
|
|
parts.append(")")
|
|
return "".join(parts)
|
|
|
|
def __hash__(self):
|
|
return super().__hash__()
|
|
|
|
def __eq__(self, other):
|
|
return super().__eq__(other)
|
|
|
|
def __lt__(self, other):
|
|
def sort_key(obj):
|
|
if isinstance(obj, str):
|
|
return (obj, "", "", "")
|
|
return (
|
|
obj._id or "",
|
|
obj.TYPE,
|
|
getattr(obj, "name", None) or "",
|
|
id(obj),
|
|
)
|
|
|
|
return sort_key(self) < sort_key(other)
|
|
|
|
|
|
class SHACLExtensibleObject(object):
|
|
CLOSED = False
|
|
|
|
def __init__(self, typ=None, **kwargs):
|
|
if typ:
|
|
self.__dict__["_obj_TYPE"] = (typ, None)
|
|
else:
|
|
self.__dict__["_obj_TYPE"] = (self._OBJ_TYPE, self._OBJ_COMPACT_TYPE)
|
|
super().__init__(**kwargs)
|
|
|
|
def _is_abstract(self):
|
|
# Unknown classes are assumed to not be abstract so that they can be
|
|
# deserialized
|
|
typ = self.__dict__["_obj_TYPE"][0]
|
|
if typ in self.__class__.CLASSES:
|
|
return self.__class__.CLASSES[typ].IS_ABSTRACT
|
|
|
|
return False
|
|
|
|
@classmethod
|
|
def _make_object(cls, typ):
|
|
# Check for a known type, and if so, deserialize as that instead
|
|
if typ in cls.CLASSES:
|
|
return cls.CLASSES[typ]()
|
|
|
|
obj = cls(typ)
|
|
return obj
|
|
|
|
def _decode_properties(self, decoder, objectset=None):
|
|
def decode_value(d):
|
|
if not d.is_list():
|
|
return d.read_value()
|
|
|
|
return [decode_value(val_d) for val_d in d.read_list()]
|
|
|
|
if self.CLOSED:
|
|
super()._decode_properties(decoder, objectset=objectset)
|
|
return
|
|
|
|
for key in decoder.object_keys():
|
|
if self._decode_prop(decoder, key, objectset=objectset):
|
|
continue
|
|
|
|
if not is_IRI(key):
|
|
raise KeyError(
|
|
f"Extensible object properties must be IRIs. Got '{key}'"
|
|
)
|
|
|
|
with decoder.read_property(key) as prop_d:
|
|
self.__dict__["_obj_data"][key] = decode_value(prop_d)
|
|
|
|
def _encode_properties(self, encoder, state):
|
|
def encode_value(encoder, v):
|
|
if isinstance(v, bool):
|
|
encoder.write_bool(v)
|
|
elif isinstance(v, str):
|
|
encoder.write_string(v)
|
|
elif isinstance(v, int):
|
|
encoder.write_integer(v)
|
|
elif isinstance(v, float):
|
|
encoder.write_float(v)
|
|
elif isinstance(v, list):
|
|
with encoder.write_list() as list_s:
|
|
for i in v:
|
|
with list_s.write_list_item() as item_s:
|
|
encode_value(item_s, i)
|
|
else:
|
|
raise TypeError(
|
|
f"Unsupported serialized type {type(v)} with value '{v}'"
|
|
)
|
|
|
|
super()._encode_properties(encoder, state)
|
|
if self.CLOSED:
|
|
return
|
|
|
|
for iri, value in self.__dict__["_obj_data"].items():
|
|
if iri in self._OBJ_PROPERTIES:
|
|
continue
|
|
|
|
with encoder.write_property(iri) as prop_s:
|
|
encode_value(prop_s, value)
|
|
|
|
def __setitem__(self, iri, value):
|
|
try:
|
|
super().__setitem__(iri, value)
|
|
except KeyError:
|
|
if self.CLOSED:
|
|
raise
|
|
|
|
if not is_IRI(iri):
|
|
raise KeyError(f"Key '{iri}' must be an IRI")
|
|
self.__dict__["_obj_data"][iri] = value
|
|
|
|
def __delitem__(self, iri):
|
|
try:
|
|
super().__delitem__(iri)
|
|
except KeyError:
|
|
if self.CLOSED:
|
|
raise
|
|
|
|
if not is_IRI(iri):
|
|
raise KeyError(f"Key '{iri}' must be an IRI")
|
|
del self.__dict__["_obj_data"][iri]
|
|
|
|
def __getattr__(self, name):
|
|
if name == "TYPE":
|
|
return self.__dict__["_obj_TYPE"][0]
|
|
if name == "COMPACT_TYPE":
|
|
return self.__dict__["_obj_TYPE"][1]
|
|
return super().__getattr__(name)
|
|
|
|
def property_keys(self):
|
|
iris = set()
|
|
for pyname, iri, compact in super().property_keys():
|
|
iris.add(iri)
|
|
yield pyname, iri, compact
|
|
|
|
if self.CLOSED:
|
|
return
|
|
|
|
for iri in self.__dict__["_obj_data"].keys():
|
|
if iri not in iris:
|
|
yield None, iri, None
|
|
|
|
|
|
class SHACLObjectSet(object):
|
|
def __init__(self, objects=[], *, link=False):
|
|
self.objects = set()
|
|
self.missing_ids = set()
|
|
for o in objects:
|
|
self.objects.add(o)
|
|
self.create_index()
|
|
if link:
|
|
self._link()
|
|
|
|
def create_index(self):
|
|
"""
|
|
(re)Create object index
|
|
|
|
Creates or recreates the indices for the object set to enable fast
|
|
lookup. All objects and their children are walked and indexed
|
|
"""
|
|
self.obj_by_id = {}
|
|
self.obj_by_type = {}
|
|
for o in self.foreach():
|
|
self.add_index(o)
|
|
|
|
def add_index(self, obj):
|
|
"""
|
|
Add object to index
|
|
|
|
Adds the object to all appropriate indices
|
|
"""
|
|
|
|
def reg_type(typ, compact, o, exact):
|
|
self.obj_by_type.setdefault(typ, set()).add((exact, o))
|
|
if compact:
|
|
self.obj_by_type.setdefault(compact, set()).add((exact, o))
|
|
|
|
if not isinstance(obj, SHACLObject):
|
|
raise TypeError("Object is not of type SHACLObject")
|
|
|
|
for typ in SHACLObject.CLASSES.values():
|
|
if isinstance(obj, typ):
|
|
reg_type(
|
|
typ._OBJ_TYPE, typ._OBJ_COMPACT_TYPE, obj, obj.__class__ is typ
|
|
)
|
|
|
|
# This covers custom extensions
|
|
reg_type(obj.TYPE, obj.COMPACT_TYPE, obj, True)
|
|
|
|
if not obj._id:
|
|
return
|
|
|
|
self.missing_ids.discard(obj._id)
|
|
|
|
if obj._id in self.obj_by_id:
|
|
return
|
|
|
|
self.obj_by_id[obj._id] = obj
|
|
|
|
def add(self, obj):
|
|
"""
|
|
Add object to object set
|
|
|
|
Adds a SHACLObject to the object set and index it.
|
|
|
|
NOTE: Child objects of the attached object are not indexes
|
|
"""
|
|
if not isinstance(obj, SHACLObject):
|
|
raise TypeError("Object is not of type SHACLObject")
|
|
|
|
if obj not in self.objects:
|
|
self.objects.add(obj)
|
|
self.add_index(obj)
|
|
return obj
|
|
|
|
def update(self, *others):
|
|
"""
|
|
Update object set adding all objects in each other iterable
|
|
"""
|
|
for o in others:
|
|
for obj in o:
|
|
self.add(obj)
|
|
|
|
def __contains__(self, item):
|
|
"""
|
|
Returns True if the item is in the object set
|
|
"""
|
|
return item in self.objects
|
|
|
|
def link(self):
|
|
"""
|
|
Link object set
|
|
|
|
Links the object in the object set by replacing string object
|
|
references with references to the objects themselves. e.g.
|
|
a property that references object "https://foo/bar" by a string
|
|
reference will be replaced with an actual reference to the object in
|
|
the object set with the same ID if it exists in the object set
|
|
|
|
If multiple objects with the same ID are found, the duplicates are
|
|
eliminated
|
|
"""
|
|
self.create_index()
|
|
return self._link()
|
|
|
|
def _link(self):
|
|
global NAMED_INDIVIDUALS
|
|
|
|
self.missing_ids = set()
|
|
visited = set()
|
|
|
|
new_objects = set()
|
|
|
|
for o in self.objects:
|
|
if o._id:
|
|
o = self.find_by_id(o._id, o)
|
|
o.link_helper(self, self.missing_ids, visited)
|
|
new_objects.add(o)
|
|
|
|
self.objects = new_objects
|
|
|
|
# Remove blank nodes
|
|
obj_by_id = {}
|
|
for _id, obj in self.obj_by_id.items():
|
|
if _id.startswith("_:"):
|
|
del obj._id
|
|
else:
|
|
obj_by_id[_id] = obj
|
|
self.obj_by_id = obj_by_id
|
|
|
|
# Named individuals aren't considered missing
|
|
self.missing_ids -= NAMED_INDIVIDUALS
|
|
|
|
return self.missing_ids
|
|
|
|
def find_by_id(self, _id, default=None):
|
|
"""
|
|
Find object by ID
|
|
|
|
Returns objects that match the specified ID, or default if there is no
|
|
object with the specified ID
|
|
"""
|
|
if _id not in self.obj_by_id:
|
|
return default
|
|
return self.obj_by_id[_id]
|
|
|
|
def foreach(self):
|
|
"""
|
|
Iterate over every object in the object set, and all child objects
|
|
"""
|
|
visited = set()
|
|
for o in self.objects:
|
|
if o not in visited:
|
|
yield o
|
|
visited.add(o)
|
|
|
|
for child in o.iter_objects(recursive=True, visited=visited):
|
|
yield child
|
|
|
|
def foreach_type(self, typ, *, match_subclass=True):
|
|
"""
|
|
Iterate over each object of a specified type (or subclass there of)
|
|
|
|
If match_subclass is True, and class derived from typ will also match
|
|
(similar to isinstance()). If False, only exact matches will be
|
|
returned
|
|
"""
|
|
if not isinstance(typ, str):
|
|
if not issubclass(typ, SHACLObject):
|
|
raise TypeError(f"Type must be derived from SHACLObject, got {typ}")
|
|
typ = typ._OBJ_TYPE
|
|
|
|
if typ not in self.obj_by_type:
|
|
return
|
|
|
|
for exact, o in self.obj_by_type[typ]:
|
|
if match_subclass or exact:
|
|
yield o
|
|
|
|
def merge(self, *objectsets):
|
|
"""
|
|
Merge object sets
|
|
|
|
Returns a new object set that is the combination of this object set and
|
|
all provided arguments
|
|
"""
|
|
new_objects = set()
|
|
new_objects |= self.objects
|
|
for d in objectsets:
|
|
new_objects |= d.objects
|
|
|
|
return SHACLObjectSet(new_objects, link=True)
|
|
|
|
def encode(self, encoder, force_list=False, *, key=None):
|
|
"""
|
|
Serialize a list of objects to a serialization encoder
|
|
|
|
If force_list is true, a list will always be written using the encoder.
|
|
"""
|
|
ref_counts = {}
|
|
state = EncodeState()
|
|
|
|
def walk_callback(value, path):
|
|
nonlocal state
|
|
nonlocal ref_counts
|
|
|
|
if not isinstance(value, SHACLObject):
|
|
return True
|
|
|
|
# Remove blank node ID for re-assignment
|
|
if value._id and value._id.startswith("_:"):
|
|
del value._id
|
|
|
|
if value._id:
|
|
state.add_refed(value)
|
|
|
|
# If the object is referenced more than once, add it to the set of
|
|
# referenced objects
|
|
ref_counts.setdefault(value, 0)
|
|
ref_counts[value] += 1
|
|
if ref_counts[value] > 1:
|
|
state.add_refed(value)
|
|
return False
|
|
|
|
return True
|
|
|
|
for o in self.objects:
|
|
if o._id:
|
|
state.add_refed(o)
|
|
o.walk(walk_callback)
|
|
|
|
use_list = force_list or len(self.objects) > 1
|
|
|
|
if use_list:
|
|
# If we are making a list add all the objects referred to by reference
|
|
# to the list
|
|
objects = list(self.objects | state.ref_objects)
|
|
else:
|
|
objects = list(self.objects)
|
|
|
|
objects.sort(key=key)
|
|
|
|
if use_list:
|
|
# Ensure top level objects are only written in the top level graph
|
|
# node, and referenced by ID everywhere else. This is done by setting
|
|
# the flag that indicates this object has been written for all the top
|
|
# level objects, then clearing it right before serializing the object.
|
|
#
|
|
# In this way, if an object is referenced before it is supposed to be
|
|
# serialized into the @graph, it will serialize as a string instead of
|
|
# the actual object
|
|
for o in objects:
|
|
state.written_objects.add(o)
|
|
|
|
with encoder.write_list() as list_s:
|
|
for o in objects:
|
|
# Allow this specific object to be written now
|
|
state.written_objects.remove(o)
|
|
with list_s.write_list_item() as item_s:
|
|
o.encode(item_s, state)
|
|
|
|
elif objects:
|
|
objects[0].encode(encoder, state)
|
|
|
|
def decode(self, decoder):
|
|
self.create_index()
|
|
|
|
for obj_d in decoder.read_list():
|
|
o = SHACLObject.decode(obj_d, objectset=self)
|
|
self.objects.add(o)
|
|
|
|
self._link()
|
|
|
|
|
|
class EncodeState(object):
|
|
def __init__(self):
|
|
self.ref_objects = set()
|
|
self.written_objects = set()
|
|
self.blank_objects = {}
|
|
|
|
def get_object_id(self, o):
|
|
if o._id:
|
|
return o._id
|
|
|
|
if o not in self.blank_objects:
|
|
_id = f"_:{o.__class__.__name__}{len(self.blank_objects)}"
|
|
self.blank_objects[o] = _id
|
|
|
|
return self.blank_objects[o]
|
|
|
|
def is_refed(self, o):
|
|
return o in self.ref_objects
|
|
|
|
def add_refed(self, o):
|
|
self.ref_objects.add(o)
|
|
|
|
def is_written(self, o):
|
|
return o in self.written_objects
|
|
|
|
def add_written(self, o):
|
|
self.written_objects.add(o)
|
|
|
|
|
|
class Decoder(ABC):
|
|
@abstractmethod
|
|
def read_value(self):
|
|
"""
|
|
Consume next item
|
|
|
|
Consumes the next item of any type
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def read_string(self):
|
|
"""
|
|
Consume the next item as a string.
|
|
|
|
Returns the string value of the next item, or `None` if the next item
|
|
is not a string
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def read_datetime(self):
|
|
"""
|
|
Consumes the next item as a date & time string
|
|
|
|
Returns the string value of the next item, if it is a ISO datetime, or
|
|
`None` if the next item is not a ISO datetime string.
|
|
|
|
Note that validation of the string is done by the caller, so a minimal
|
|
implementation can just check if the next item is a string without
|
|
worrying about the format
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def read_integer(self):
|
|
"""
|
|
Consumes the next item as an integer
|
|
|
|
Returns the integer value of the next item, or `None` if the next item
|
|
is not an integer
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def read_iri(self):
|
|
"""
|
|
Consumes the next item as an IRI string
|
|
|
|
Returns the string value of the next item an IRI, or `None` if the next
|
|
item is not an IRI.
|
|
|
|
The returned string should be either a fully-qualified IRI, or a blank
|
|
node ID
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def read_enum(self, e):
|
|
"""
|
|
Consumes the next item as an Enum value string
|
|
|
|
Returns the fully qualified IRI of the next enum item, or `None` if the
|
|
next item is not an enum value.
|
|
|
|
The callee is responsible for validating that the returned IRI is
|
|
actually a member of the specified Enum, so the `Decoder` does not need
|
|
to check that, but can if it wishes
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def read_bool(self):
|
|
"""
|
|
Consume the next item as a boolean value
|
|
|
|
Returns the boolean value of the next item, or `None` if the next item
|
|
is not a boolean
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def read_float(self):
|
|
"""
|
|
Consume the next item as a float value
|
|
|
|
Returns the float value of the next item, or `None` if the next item is
|
|
not a float
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def read_list(self):
|
|
"""
|
|
Consume the next item as a list generator
|
|
|
|
This should generate a `Decoder` object for each item in the list. The
|
|
generated `Decoder` can be used to read the corresponding item from the
|
|
list
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def is_list(self):
|
|
"""
|
|
Checks if the next item is a list
|
|
|
|
Returns True if the next item is a list, or False if it is a scalar
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def read_object(self):
|
|
"""
|
|
Consume next item as an object
|
|
|
|
A context manager that "enters" the next item as a object and yields a
|
|
`Decoder` that can read properties from it. If the next item is not an
|
|
object, yields `None`
|
|
|
|
Properties will be read out of the object using `read_property` and
|
|
`read_object_id`
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
@contextmanager
|
|
def read_property(self, key):
|
|
"""
|
|
Read property from object
|
|
|
|
A context manager that yields a `Decoder` that can be used to read the
|
|
value of the property with the given key in current object, or `None`
|
|
if the property does not exist in the current object.
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def object_keys(self):
|
|
"""
|
|
Read property keys from an object
|
|
|
|
Iterates over all the serialized keys for the current object
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def read_object_id(self, alias=None):
|
|
"""
|
|
Read current object ID property
|
|
|
|
Returns the ID of the current object if one is defined, or `None` if
|
|
the current object has no ID.
|
|
|
|
The ID must be a fully qualified IRI or a blank node
|
|
|
|
If `alias` is provided, is is a hint as to another name by which the ID
|
|
might be found, if the `Decoder` supports aliases for an ID
|
|
"""
|
|
pass
|
|
|
|
|
|
class JSONLDDecoder(Decoder):
|
|
def __init__(self, data, root=False):
|
|
self.data = data
|
|
self.root = root
|
|
|
|
def read_value(self):
|
|
if isinstance(self.data, str):
|
|
try:
|
|
return float(self.data)
|
|
except ValueError:
|
|
pass
|
|
return self.data
|
|
|
|
def read_string(self):
|
|
if isinstance(self.data, str):
|
|
return self.data
|
|
return None
|
|
|
|
def read_datetime(self):
|
|
return self.read_string()
|
|
|
|
def read_integer(self):
|
|
if isinstance(self.data, int):
|
|
return self.data
|
|
return None
|
|
|
|
def read_bool(self):
|
|
if isinstance(self.data, bool):
|
|
return self.data
|
|
return None
|
|
|
|
def read_float(self):
|
|
if isinstance(self.data, (int, float, str)):
|
|
return float(self.data)
|
|
return None
|
|
|
|
def read_iri(self):
|
|
if isinstance(self.data, str):
|
|
return self.data
|
|
return None
|
|
|
|
def read_enum(self, e):
|
|
if isinstance(self.data, str):
|
|
return self.data
|
|
return None
|
|
|
|
def read_list(self):
|
|
if self.is_list():
|
|
for v in self.data:
|
|
yield self.__class__(v)
|
|
else:
|
|
yield self
|
|
|
|
def is_list(self):
|
|
return isinstance(self.data, (list, tuple, set))
|
|
|
|
def __get_value(self, *keys):
|
|
for k in keys:
|
|
if k and k in self.data:
|
|
return self.data[k]
|
|
return None
|
|
|
|
@contextmanager
|
|
def read_property(self, key):
|
|
v = self.__get_value(key)
|
|
if v is not None:
|
|
yield self.__class__(v)
|
|
else:
|
|
yield None
|
|
|
|
def object_keys(self):
|
|
for key in self.data.keys():
|
|
if key in ("@type", "type"):
|
|
continue
|
|
if self.root and key == "@context":
|
|
continue
|
|
yield key
|
|
|
|
def read_object(self):
|
|
typ = self.__get_value("@type", "type")
|
|
if typ is not None:
|
|
return typ, self
|
|
|
|
return None, self
|
|
|
|
def read_object_id(self, alias=None):
|
|
return self.__get_value(alias, "@id")
|
|
|
|
|
|
class JSONLDDeserializer(object):
|
|
def deserialize_data(self, data, objectset: SHACLObjectSet):
|
|
if "@graph" in data:
|
|
h = JSONLDDecoder(data["@graph"], True)
|
|
else:
|
|
h = JSONLDDecoder(data, True)
|
|
|
|
objectset.decode(h)
|
|
|
|
def read(self, f, objectset: SHACLObjectSet):
|
|
data = json.load(f)
|
|
self.deserialize_data(data, objectset)
|
|
|
|
|
|
class Encoder(ABC):
|
|
@abstractmethod
|
|
def write_string(self, v):
|
|
"""
|
|
Write a string value
|
|
|
|
Encodes the value as a string in the output
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def write_datetime(self, v):
|
|
"""
|
|
Write a date & time string
|
|
|
|
Encodes the value as an ISO datetime string
|
|
|
|
Note: The provided string is already correctly encoded as an ISO datetime
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def write_integer(self, v):
|
|
"""
|
|
Write an integer value
|
|
|
|
Encodes the value as an integer in the output
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def write_iri(self, v, compact=None):
|
|
"""
|
|
Write IRI
|
|
|
|
Encodes the string as an IRI. Note that the string will be either a
|
|
fully qualified IRI or a blank node ID. If `compact` is provided and
|
|
the serialization supports compacted IRIs, it should be preferred to
|
|
the full IRI
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def write_enum(self, v, e, compact=None):
|
|
"""
|
|
Write enum value IRI
|
|
|
|
Encodes the string enum value IRI. Note that the string will be a fully
|
|
qualified IRI. If `compact` is provided and the serialization supports
|
|
compacted IRIs, it should be preferred to the full IRI.
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def write_bool(self, v):
|
|
"""
|
|
Write boolean
|
|
|
|
Encodes the value as a boolean in the output
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
def write_float(self, v):
|
|
"""
|
|
Write float
|
|
|
|
Encodes the value as a floating point number in the output
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
@contextmanager
|
|
def write_object(self, o, _id, needs_id):
|
|
"""
|
|
Write object
|
|
|
|
A context manager that yields an `Encoder` that can be used to encode
|
|
the given object properties.
|
|
|
|
The provided ID will always be a valid ID (even if o._id is `None`), in
|
|
case the `Encoder` _must_ have an ID. `needs_id` is a hint to indicate
|
|
to the `Encoder` if an ID must be written or not (if that is even an
|
|
option). If it is `True`, the `Encoder` must encode an ID for the
|
|
object. If `False`, the encoder is not required to encode an ID and may
|
|
omit it.
|
|
|
|
The ID will be either a fully qualified IRI, or a blank node IRI.
|
|
|
|
Properties will be written the object using `write_property`
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
@contextmanager
|
|
def write_property(self, iri, compact=None):
|
|
"""
|
|
Write object property
|
|
|
|
A context manager that yields an `Encoder` that can be used to encode
|
|
the value for the property with the given IRI in the current object
|
|
|
|
Note that the IRI will be fully qualified. If `compact` is provided and
|
|
the serialization supports compacted IRIs, it should be preferred to
|
|
the full IRI.
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
@contextmanager
|
|
def write_list(self):
|
|
"""
|
|
Write list
|
|
|
|
A context manager that yields an `Encoder` that can be used to encode a
|
|
list.
|
|
|
|
Each item of the list will be added using `write_list_item`
|
|
"""
|
|
pass
|
|
|
|
@abstractmethod
|
|
@contextmanager
|
|
def write_list_item(self):
|
|
"""
|
|
Write list item
|
|
|
|
A context manager that yields an `Encoder` that can be used to encode
|
|
the value for a list item
|
|
"""
|
|
pass
|
|
|
|
|
|
class JSONLDEncoder(Encoder):
|
|
def __init__(self, data=None):
|
|
self.data = data
|
|
|
|
def write_string(self, v):
|
|
self.data = v
|
|
|
|
def write_datetime(self, v):
|
|
self.data = v
|
|
|
|
def write_integer(self, v):
|
|
self.data = v
|
|
|
|
def write_iri(self, v, compact=None):
|
|
self.write_string(compact or v)
|
|
|
|
def write_enum(self, v, e, compact=None):
|
|
self.write_string(compact or v)
|
|
|
|
def write_bool(self, v):
|
|
self.data = v
|
|
|
|
def write_float(self, v):
|
|
self.data = str(v)
|
|
|
|
@contextmanager
|
|
def write_property(self, iri, compact=None):
|
|
s = self.__class__(None)
|
|
yield s
|
|
if s.data is not None:
|
|
self.data[compact or iri] = s.data
|
|
|
|
@contextmanager
|
|
def write_object(self, o, _id, needs_id):
|
|
self.data = {
|
|
"type": o.COMPACT_TYPE or o.TYPE,
|
|
}
|
|
if needs_id:
|
|
self.data[o.ID_ALIAS or "@id"] = _id
|
|
yield self
|
|
|
|
@contextmanager
|
|
def write_list(self):
|
|
self.data = []
|
|
yield self
|
|
if not self.data:
|
|
self.data = None
|
|
|
|
@contextmanager
|
|
def write_list_item(self):
|
|
s = self.__class__(None)
|
|
yield s
|
|
if s.data is not None:
|
|
self.data.append(s.data)
|
|
|
|
|
|
class JSONLDSerializer(object):
|
|
def __init__(self, **args):
|
|
self.args = args
|
|
|
|
def serialize_data(
|
|
self,
|
|
objectset: SHACLObjectSet,
|
|
force_at_graph=False,
|
|
):
|
|
h = JSONLDEncoder()
|
|
objectset.encode(h, force_at_graph)
|
|
data = {}
|
|
if len(CONTEXT_URLS) == 1:
|
|
data["@context"] = CONTEXT_URLS[0]
|
|
elif CONTEXT_URLS:
|
|
data["@context"] = CONTEXT_URLS
|
|
|
|
if isinstance(h.data, list):
|
|
data["@graph"] = h.data
|
|
else:
|
|
for k, v in h.data.items():
|
|
data[k] = v
|
|
|
|
return data
|
|
|
|
def write(
|
|
self,
|
|
objectset: SHACLObjectSet,
|
|
f,
|
|
force_at_graph=False,
|
|
**kwargs,
|
|
):
|
|
"""
|
|
Write a SHACLObjectSet to a JSON LD file
|
|
|
|
If force_at_graph is True, a @graph node will always be written
|
|
"""
|
|
data = self.serialize_data(objectset, force_at_graph)
|
|
|
|
args = {**self.args, **kwargs}
|
|
|
|
sha1 = hashlib.sha1()
|
|
for chunk in json.JSONEncoder(**args).iterencode(data):
|
|
chunk = chunk.encode("utf-8")
|
|
f.write(chunk)
|
|
sha1.update(chunk)
|
|
|
|
return sha1.hexdigest()
|
|
|
|
|
|
class JSONLDInlineEncoder(Encoder):
|
|
def __init__(self, f, sha1):
|
|
self.f = f
|
|
self.comma = False
|
|
self.sha1 = sha1
|
|
|
|
def write(self, s):
|
|
s = s.encode("utf-8")
|
|
self.f.write(s)
|
|
self.sha1.update(s)
|
|
|
|
def _write_comma(self):
|
|
if self.comma:
|
|
self.write(",")
|
|
self.comma = False
|
|
|
|
def write_string(self, v):
|
|
self.write(json.dumps(v))
|
|
|
|
def write_datetime(self, v):
|
|
self.write_string(v)
|
|
|
|
def write_integer(self, v):
|
|
self.write(f"{v}")
|
|
|
|
def write_iri(self, v, compact=None):
|
|
self.write_string(compact or v)
|
|
|
|
def write_enum(self, v, e, compact=None):
|
|
self.write_iri(v, compact)
|
|
|
|
def write_bool(self, v):
|
|
if v:
|
|
self.write("true")
|
|
else:
|
|
self.write("false")
|
|
|
|
def write_float(self, v):
|
|
self.write(json.dumps(str(v)))
|
|
|
|
@contextmanager
|
|
def write_property(self, iri, compact=None):
|
|
self._write_comma()
|
|
self.write_string(compact or iri)
|
|
self.write(":")
|
|
yield self
|
|
self.comma = True
|
|
|
|
@contextmanager
|
|
def write_object(self, o, _id, needs_id):
|
|
self._write_comma()
|
|
|
|
self.write("{")
|
|
self.write_string("type")
|
|
self.write(":")
|
|
self.write_string(o.COMPACT_TYPE or o.TYPE)
|
|
self.comma = True
|
|
|
|
if needs_id:
|
|
self._write_comma()
|
|
self.write_string(o.ID_ALIAS or "@id")
|
|
self.write(":")
|
|
self.write_string(_id)
|
|
self.comma = True
|
|
|
|
self.comma = True
|
|
yield self
|
|
|
|
self.write("}")
|
|
self.comma = True
|
|
|
|
@contextmanager
|
|
def write_list(self):
|
|
self._write_comma()
|
|
self.write("[")
|
|
yield self.__class__(self.f, self.sha1)
|
|
self.write("]")
|
|
self.comma = True
|
|
|
|
@contextmanager
|
|
def write_list_item(self):
|
|
self._write_comma()
|
|
yield self.__class__(self.f, self.sha1)
|
|
self.comma = True
|
|
|
|
|
|
class JSONLDInlineSerializer(object):
|
|
def write(
|
|
self,
|
|
objectset: SHACLObjectSet,
|
|
f,
|
|
force_at_graph=False,
|
|
):
|
|
"""
|
|
Write a SHACLObjectSet to a JSON LD file
|
|
|
|
Note: force_at_graph is included for compatibility, but ignored. This
|
|
serializer always writes out a graph
|
|
"""
|
|
sha1 = hashlib.sha1()
|
|
h = JSONLDInlineEncoder(f, sha1)
|
|
h.write('{"@context":')
|
|
if len(CONTEXT_URLS) == 1:
|
|
h.write(f'"{CONTEXT_URLS[0]}"')
|
|
elif CONTEXT_URLS:
|
|
h.write('["')
|
|
h.write('","'.join(CONTEXT_URLS))
|
|
h.write('"]')
|
|
h.write(",")
|
|
|
|
h.write('"@graph":')
|
|
|
|
objectset.encode(h, True)
|
|
h.write("}")
|
|
return sha1.hexdigest()
|
|
|
|
|
|
def print_tree(objects, all_fields=False):
|
|
"""
|
|
Print object tree
|
|
"""
|
|
seen = set()
|
|
|
|
def callback(value, path):
|
|
nonlocal seen
|
|
|
|
s = (" " * (len(path) - 1)) + f"{path[-1]}"
|
|
if isinstance(value, SHACLObject):
|
|
s += f" {value} ({id(value)})"
|
|
is_empty = False
|
|
elif isinstance(value, ListProxy):
|
|
is_empty = len(value) == 0
|
|
if is_empty:
|
|
s += " []"
|
|
else:
|
|
s += f" {value!r}"
|
|
is_empty = value is None
|
|
|
|
if all_fields or not is_empty:
|
|
print(s)
|
|
|
|
if isinstance(value, SHACLObject):
|
|
if value in seen:
|
|
return False
|
|
seen.add(value)
|
|
return True
|
|
|
|
return True
|
|
|
|
for o in objects:
|
|
o.walk(callback)
|
|
|
|
|
|
# fmt: off
|
|
"""Format Guard"""
|
|
|
|
|
|
CONTEXT_URLS = [
|
|
"https://spdx.org/rdf/3.0.1/spdx-context.jsonld",
|
|
]
|
|
|
|
|
|
# CLASSES
|
|
# A class for describing the energy consumption incurred by an AI model in
|
|
# different stages of its lifecycle.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/AI/EnergyConsumption", compact_type="ai_EnergyConsumption", abstract=False)
|
|
class ai_EnergyConsumption(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Specifies the amount of energy consumed when finetuning the AI model that is
|
|
# being used in the AI system.
|
|
cls._add_property(
|
|
"ai_finetuningEnergyConsumption",
|
|
ListProp(ObjectProp(ai_EnergyConsumptionDescription, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/finetuningEnergyConsumption",
|
|
compact="ai_finetuningEnergyConsumption",
|
|
)
|
|
# Specifies the amount of energy consumed during inference time by an AI model
|
|
# that is being used in the AI system.
|
|
cls._add_property(
|
|
"ai_inferenceEnergyConsumption",
|
|
ListProp(ObjectProp(ai_EnergyConsumptionDescription, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/inferenceEnergyConsumption",
|
|
compact="ai_inferenceEnergyConsumption",
|
|
)
|
|
# Specifies the amount of energy consumed when training the AI model that is
|
|
# being used in the AI system.
|
|
cls._add_property(
|
|
"ai_trainingEnergyConsumption",
|
|
ListProp(ObjectProp(ai_EnergyConsumptionDescription, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/trainingEnergyConsumption",
|
|
compact="ai_trainingEnergyConsumption",
|
|
)
|
|
|
|
|
|
# The class that helps note down the quantity of energy consumption and the unit
|
|
# used for measurement.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/AI/EnergyConsumptionDescription", compact_type="ai_EnergyConsumptionDescription", abstract=False)
|
|
class ai_EnergyConsumptionDescription(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Represents the energy quantity.
|
|
cls._add_property(
|
|
"ai_energyQuantity",
|
|
FloatProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/energyQuantity",
|
|
min_count=1,
|
|
compact="ai_energyQuantity",
|
|
)
|
|
# Specifies the unit in which energy is measured.
|
|
cls._add_property(
|
|
"ai_energyUnit",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/AI/EnergyUnitType/kilowattHour", "kilowattHour"),
|
|
("https://spdx.org/rdf/3.0.1/terms/AI/EnergyUnitType/megajoule", "megajoule"),
|
|
("https://spdx.org/rdf/3.0.1/terms/AI/EnergyUnitType/other", "other"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/energyUnit",
|
|
min_count=1,
|
|
compact="ai_energyUnit",
|
|
)
|
|
|
|
|
|
# Specifies the unit of energy consumption.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/AI/EnergyUnitType", compact_type="ai_EnergyUnitType", abstract=False)
|
|
class ai_EnergyUnitType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"kilowattHour": "https://spdx.org/rdf/3.0.1/terms/AI/EnergyUnitType/kilowattHour",
|
|
"megajoule": "https://spdx.org/rdf/3.0.1/terms/AI/EnergyUnitType/megajoule",
|
|
"other": "https://spdx.org/rdf/3.0.1/terms/AI/EnergyUnitType/other",
|
|
}
|
|
# Kilowatt-hour.
|
|
kilowattHour = "https://spdx.org/rdf/3.0.1/terms/AI/EnergyUnitType/kilowattHour"
|
|
# Megajoule.
|
|
megajoule = "https://spdx.org/rdf/3.0.1/terms/AI/EnergyUnitType/megajoule"
|
|
# Any other units of energy measurement.
|
|
other = "https://spdx.org/rdf/3.0.1/terms/AI/EnergyUnitType/other"
|
|
|
|
|
|
# Specifies the safety risk level.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType", compact_type="ai_SafetyRiskAssessmentType", abstract=False)
|
|
class ai_SafetyRiskAssessmentType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"high": "https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType/high",
|
|
"low": "https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType/low",
|
|
"medium": "https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType/medium",
|
|
"serious": "https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType/serious",
|
|
}
|
|
# The second-highest level of risk posed by an AI system.
|
|
high = "https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType/high"
|
|
# Low/no risk is posed by an AI system.
|
|
low = "https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType/low"
|
|
# The third-highest level of risk posed by an AI system.
|
|
medium = "https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType/medium"
|
|
# The highest level of risk posed by an AI system.
|
|
serious = "https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType/serious"
|
|
|
|
|
|
# Specifies the type of an annotation.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/AnnotationType", compact_type="AnnotationType", abstract=False)
|
|
class AnnotationType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"other": "https://spdx.org/rdf/3.0.1/terms/Core/AnnotationType/other",
|
|
"review": "https://spdx.org/rdf/3.0.1/terms/Core/AnnotationType/review",
|
|
}
|
|
# Used to store extra information about an Element which is not part of a review (e.g. extra information provided during the creation of the Element).
|
|
other = "https://spdx.org/rdf/3.0.1/terms/Core/AnnotationType/other"
|
|
# Used when someone reviews the Element.
|
|
review = "https://spdx.org/rdf/3.0.1/terms/Core/AnnotationType/review"
|
|
|
|
|
|
# Provides information about the creation of the Element.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/CreationInfo", compact_type="CreationInfo", abstract=False)
|
|
class CreationInfo(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provide consumers with comments by the creator of the Element about the
|
|
# Element.
|
|
cls._add_property(
|
|
"comment",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/comment",
|
|
compact="comment",
|
|
)
|
|
# Identifies when the Element was originally created.
|
|
cls._add_property(
|
|
"created",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/created",
|
|
min_count=1,
|
|
compact="created",
|
|
)
|
|
# Identifies who or what created the Element.
|
|
cls._add_property(
|
|
"createdBy",
|
|
ListProp(ObjectProp(Agent, False, context=[
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SpdxOrganization", "SpdxOrganization"),
|
|
],)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/createdBy",
|
|
min_count=1,
|
|
compact="createdBy",
|
|
)
|
|
# Identifies the tooling that was used during the creation of the Element.
|
|
cls._add_property(
|
|
"createdUsing",
|
|
ListProp(ObjectProp(Tool, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/createdUsing",
|
|
compact="createdUsing",
|
|
)
|
|
# Provides a reference number that can be used to understand how to parse and
|
|
# interpret an Element.
|
|
cls._add_property(
|
|
"specVersion",
|
|
StringProp(pattern=r"^(0|[1-9]\d*)\.(0|[1-9]\d*)\.(0|[1-9]\d*)(?:-((?:0|[1-9]\d*|\d*[a-zA-Z-][0-9a-zA-Z-]*)(?:\.(?:0|[1-9]\d*|\d*[a-zA-Z-][0-9a-zA-Z-]*))*))?(?:\+([0-9a-zA-Z-]+(?:\.[0-9a-zA-Z-]+)*))?$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/specVersion",
|
|
min_count=1,
|
|
compact="specVersion",
|
|
)
|
|
|
|
|
|
# A key with an associated value.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/DictionaryEntry", compact_type="DictionaryEntry", abstract=False)
|
|
class DictionaryEntry(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# A key used in a generic key-value pair.
|
|
cls._add_property(
|
|
"key",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/key",
|
|
min_count=1,
|
|
compact="key",
|
|
)
|
|
# A value used in a generic key-value pair.
|
|
cls._add_property(
|
|
"value",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/value",
|
|
compact="value",
|
|
)
|
|
|
|
|
|
# Base domain class from which all other SPDX-3.0 domain classes derive.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/Element", compact_type="Element", abstract=True)
|
|
class Element(SHACLObject):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provide consumers with comments by the creator of the Element about the
|
|
# Element.
|
|
cls._add_property(
|
|
"comment",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/comment",
|
|
compact="comment",
|
|
)
|
|
# Provides information about the creation of the Element.
|
|
cls._add_property(
|
|
"creationInfo",
|
|
ObjectProp(CreationInfo, True),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/creationInfo",
|
|
min_count=1,
|
|
compact="creationInfo",
|
|
)
|
|
# Provides a detailed description of the Element.
|
|
cls._add_property(
|
|
"description",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/description",
|
|
compact="description",
|
|
)
|
|
# Specifies an Extension characterization of some aspect of an Element.
|
|
cls._add_property(
|
|
"extension",
|
|
ListProp(ObjectProp(extension_Extension, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/extension",
|
|
compact="extension",
|
|
)
|
|
# Provides a reference to a resource outside the scope of SPDX-3.0 content
|
|
# that uniquely identifies an Element.
|
|
cls._add_property(
|
|
"externalIdentifier",
|
|
ListProp(ObjectProp(ExternalIdentifier, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/externalIdentifier",
|
|
compact="externalIdentifier",
|
|
)
|
|
# Points to a resource outside the scope of the SPDX-3.0 content
|
|
# that provides additional characteristics of an Element.
|
|
cls._add_property(
|
|
"externalRef",
|
|
ListProp(ObjectProp(ExternalRef, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/externalRef",
|
|
compact="externalRef",
|
|
)
|
|
# Identifies the name of an Element as designated by the creator.
|
|
cls._add_property(
|
|
"name",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/name",
|
|
compact="name",
|
|
)
|
|
# A short description of an Element.
|
|
cls._add_property(
|
|
"summary",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/summary",
|
|
compact="summary",
|
|
)
|
|
# Provides an IntegrityMethod with which the integrity of an Element can be
|
|
# asserted.
|
|
cls._add_property(
|
|
"verifiedUsing",
|
|
ListProp(ObjectProp(IntegrityMethod, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/verifiedUsing",
|
|
compact="verifiedUsing",
|
|
)
|
|
|
|
|
|
# A collection of Elements, not necessarily with unifying context.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/ElementCollection", compact_type="ElementCollection", abstract=True)
|
|
class ElementCollection(Element):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Refers to one or more Elements that are part of an ElementCollection.
|
|
cls._add_property(
|
|
"element",
|
|
ListProp(ObjectProp(Element, False, context=[
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/NoneElement", "NoneElement"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoAssertionLicense", "expandedlicensing_NoAssertionLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoneLicense", "expandedlicensing_NoneLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SpdxOrganization", "SpdxOrganization"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/NoAssertionElement", "NoAssertionElement"),
|
|
],)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/element",
|
|
compact="element",
|
|
)
|
|
# Describes one a profile which the creator of this ElementCollection intends to
|
|
# conform to.
|
|
cls._add_property(
|
|
"profileConformance",
|
|
ListProp(EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/ai", "ai"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/build", "build"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/core", "core"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/dataset", "dataset"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/expandedLicensing", "expandedLicensing"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/extension", "extension"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/lite", "lite"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/security", "security"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/simpleLicensing", "simpleLicensing"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/software", "software"),
|
|
])),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/profileConformance",
|
|
compact="profileConformance",
|
|
)
|
|
# This property is used to denote the root Element(s) of a tree of elements contained in a BOM.
|
|
cls._add_property(
|
|
"rootElement",
|
|
ListProp(ObjectProp(Element, False, context=[
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/NoneElement", "NoneElement"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoAssertionLicense", "expandedlicensing_NoAssertionLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoneLicense", "expandedlicensing_NoneLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SpdxOrganization", "SpdxOrganization"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/NoAssertionElement", "NoAssertionElement"),
|
|
],)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/rootElement",
|
|
compact="rootElement",
|
|
)
|
|
|
|
|
|
# A reference to a resource identifier defined outside the scope of SPDX-3.0 content that uniquely identifies an Element.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifier", compact_type="ExternalIdentifier", abstract=False)
|
|
class ExternalIdentifier(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provide consumers with comments by the creator of the Element about the
|
|
# Element.
|
|
cls._add_property(
|
|
"comment",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/comment",
|
|
compact="comment",
|
|
)
|
|
# Specifies the type of the external identifier.
|
|
cls._add_property(
|
|
"externalIdentifierType",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/cpe22", "cpe22"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/cpe23", "cpe23"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/cve", "cve"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/email", "email"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/gitoid", "gitoid"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/other", "other"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/packageUrl", "packageUrl"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/securityOther", "securityOther"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/swhid", "swhid"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/swid", "swid"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/urlScheme", "urlScheme"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/externalIdentifierType",
|
|
min_count=1,
|
|
compact="externalIdentifierType",
|
|
)
|
|
# Uniquely identifies an external element.
|
|
cls._add_property(
|
|
"identifier",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/identifier",
|
|
min_count=1,
|
|
compact="identifier",
|
|
)
|
|
# Provides the location for more information regarding an external identifier.
|
|
cls._add_property(
|
|
"identifierLocator",
|
|
ListProp(AnyURIProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/identifierLocator",
|
|
compact="identifierLocator",
|
|
)
|
|
# An entity that is authorized to issue identification credentials.
|
|
cls._add_property(
|
|
"issuingAuthority",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/issuingAuthority",
|
|
compact="issuingAuthority",
|
|
)
|
|
|
|
|
|
# Specifies the type of an external identifier.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType", compact_type="ExternalIdentifierType", abstract=False)
|
|
class ExternalIdentifierType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"cpe22": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/cpe22",
|
|
"cpe23": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/cpe23",
|
|
"cve": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/cve",
|
|
"email": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/email",
|
|
"gitoid": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/gitoid",
|
|
"other": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/other",
|
|
"packageUrl": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/packageUrl",
|
|
"securityOther": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/securityOther",
|
|
"swhid": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/swhid",
|
|
"swid": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/swid",
|
|
"urlScheme": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/urlScheme",
|
|
}
|
|
# [Common Platform Enumeration Specification 2.2](https://cpe.mitre.org/files/cpe-specification_2.2.pdf)
|
|
cpe22 = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/cpe22"
|
|
# [Common Platform Enumeration: Naming Specification Version 2.3](https://csrc.nist.gov/publications/detail/nistir/7695/final)
|
|
cpe23 = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/cpe23"
|
|
# Common Vulnerabilities and Exposures identifiers, an identifier for a specific software flaw defined within the official CVE Dictionary and that conforms to the [CVE specification](https://csrc.nist.gov/glossary/term/cve_id).
|
|
cve = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/cve"
|
|
# Email address, as defined in [RFC 3696](https://datatracker.ietf.org/doc/rfc3986/) Section 3.
|
|
email = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/email"
|
|
# [Gitoid](https://www.iana.org/assignments/uri-schemes/prov/gitoid), stands for [Git Object ID](https://git-scm.com/book/en/v2/Git-Internals-Git-Objects). A gitoid of type blob is a unique hash of a binary artifact. A gitoid may represent either an [Artifact Identifier](https://github.com/omnibor/spec/blob/eb1ee5c961c16215eb8709b2975d193a2007a35d/spec/SPEC.md#artifact-identifier-types) for the software artifact or an [Input Manifest Identifier](https://github.com/omnibor/spec/blob/eb1ee5c961c16215eb8709b2975d193a2007a35d/spec/SPEC.md#input-manifest-identifier) for the software artifact's associated [Artifact Input Manifest](https://github.com/omnibor/spec/blob/eb1ee5c961c16215eb8709b2975d193a2007a35d/spec/SPEC.md#artifact-input-manifest); this ambiguity exists because the Artifact Input Manifest is itself an artifact, and the gitoid of that artifact is its valid identifier. Gitoids calculated on software artifacts (Snippet, File, or Package Elements) should be recorded in the SPDX 3.0 SoftwareArtifact's contentIdentifier property. Gitoids calculated on the Artifact Input Manifest (Input Manifest Identifier) should be recorded in the SPDX 3.0 Element's externalIdentifier property. See [OmniBOR Specification](https://github.com/omnibor/spec/), a minimalistic specification for describing software [Artifact Dependency Graphs](https://github.com/omnibor/spec/blob/eb1ee5c961c16215eb8709b2975d193a2007a35d/spec/SPEC.md#artifact-dependency-graph-adg).
|
|
gitoid = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/gitoid"
|
|
# Used when the type does not match any of the other options.
|
|
other = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/other"
|
|
# Package URL, as defined in the corresponding [Annex](../../../annexes/pkg-url-specification.md) of this specification.
|
|
packageUrl = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/packageUrl"
|
|
# Used when there is a security related identifier of unspecified type.
|
|
securityOther = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/securityOther"
|
|
# SoftWare Hash IDentifier, a persistent intrinsic identifier for digital artifacts, such as files, trees (also known as directories or folders), commits, and other objects typically found in version control systems. The format of the identifiers is defined in the [SWHID specification](https://www.swhid.org/specification/v1.1/4.Syntax) (ISO/IEC DIS 18670). They typically look like `swh:1:cnt:94a9ed024d3859793618152ea559a168bbcbb5e2`.
|
|
swhid = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/swhid"
|
|
# Concise Software Identification (CoSWID) tag, as defined in [RFC 9393](https://datatracker.ietf.org/doc/rfc9393/) Section 2.3.
|
|
swid = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/swid"
|
|
# [Uniform Resource Identifier (URI) Schemes](https://www.iana.org/assignments/uri-schemes/uri-schemes.xhtml). The scheme used in order to locate a resource.
|
|
urlScheme = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalIdentifierType/urlScheme"
|
|
|
|
|
|
# A map of Element identifiers that are used within an SpdxDocument but defined
|
|
# external to that SpdxDocument.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/ExternalMap", compact_type="ExternalMap", abstract=False)
|
|
class ExternalMap(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Artifact representing a serialization instance of SPDX data containing the
|
|
# definition of a particular Element.
|
|
cls._add_property(
|
|
"definingArtifact",
|
|
ObjectProp(Artifact, False),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/definingArtifact",
|
|
compact="definingArtifact",
|
|
)
|
|
# Identifies an external Element used within an SpdxDocument but defined
|
|
# external to that SpdxDocument.
|
|
cls._add_property(
|
|
"externalSpdxId",
|
|
AnyURIProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/externalSpdxId",
|
|
min_count=1,
|
|
compact="externalSpdxId",
|
|
)
|
|
# Provides an indication of where to retrieve an external Element.
|
|
cls._add_property(
|
|
"locationHint",
|
|
AnyURIProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/locationHint",
|
|
compact="locationHint",
|
|
)
|
|
# Provides an IntegrityMethod with which the integrity of an Element can be
|
|
# asserted.
|
|
cls._add_property(
|
|
"verifiedUsing",
|
|
ListProp(ObjectProp(IntegrityMethod, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/verifiedUsing",
|
|
compact="verifiedUsing",
|
|
)
|
|
|
|
|
|
# A reference to a resource outside the scope of SPDX-3.0 content related to an Element.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRef", compact_type="ExternalRef", abstract=False)
|
|
class ExternalRef(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provide consumers with comments by the creator of the Element about the
|
|
# Element.
|
|
cls._add_property(
|
|
"comment",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/comment",
|
|
compact="comment",
|
|
)
|
|
# Provides information about the content type of an Element or a Property.
|
|
cls._add_property(
|
|
"contentType",
|
|
StringProp(pattern=r"^[^\/]+\/[^\/]+$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/contentType",
|
|
compact="contentType",
|
|
)
|
|
# Specifies the type of the external reference.
|
|
cls._add_property(
|
|
"externalRefType",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/altDownloadLocation", "altDownloadLocation"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/altWebPage", "altWebPage"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/binaryArtifact", "binaryArtifact"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/bower", "bower"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/buildMeta", "buildMeta"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/buildSystem", "buildSystem"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/certificationReport", "certificationReport"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/chat", "chat"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/componentAnalysisReport", "componentAnalysisReport"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/cwe", "cwe"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/documentation", "documentation"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/dynamicAnalysisReport", "dynamicAnalysisReport"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/eolNotice", "eolNotice"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/exportControlAssessment", "exportControlAssessment"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/funding", "funding"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/issueTracker", "issueTracker"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/license", "license"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/mailingList", "mailingList"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/mavenCentral", "mavenCentral"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/metrics", "metrics"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/npm", "npm"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/nuget", "nuget"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/other", "other"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/privacyAssessment", "privacyAssessment"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/productMetadata", "productMetadata"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/purchaseOrder", "purchaseOrder"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/qualityAssessmentReport", "qualityAssessmentReport"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/releaseHistory", "releaseHistory"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/releaseNotes", "releaseNotes"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/riskAssessment", "riskAssessment"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/runtimeAnalysisReport", "runtimeAnalysisReport"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/secureSoftwareAttestation", "secureSoftwareAttestation"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityAdversaryModel", "securityAdversaryModel"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityAdvisory", "securityAdvisory"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityFix", "securityFix"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityOther", "securityOther"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityPenTestReport", "securityPenTestReport"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityPolicy", "securityPolicy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityThreatModel", "securityThreatModel"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/socialMedia", "socialMedia"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/sourceArtifact", "sourceArtifact"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/staticAnalysisReport", "staticAnalysisReport"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/support", "support"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/vcs", "vcs"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/vulnerabilityDisclosureReport", "vulnerabilityDisclosureReport"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/vulnerabilityExploitabilityAssessment", "vulnerabilityExploitabilityAssessment"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/externalRefType",
|
|
compact="externalRefType",
|
|
)
|
|
# Provides the location of an external reference.
|
|
cls._add_property(
|
|
"locator",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/locator",
|
|
compact="locator",
|
|
)
|
|
|
|
|
|
# Specifies the type of an external reference.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType", compact_type="ExternalRefType", abstract=False)
|
|
class ExternalRefType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"altDownloadLocation": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/altDownloadLocation",
|
|
"altWebPage": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/altWebPage",
|
|
"binaryArtifact": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/binaryArtifact",
|
|
"bower": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/bower",
|
|
"buildMeta": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/buildMeta",
|
|
"buildSystem": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/buildSystem",
|
|
"certificationReport": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/certificationReport",
|
|
"chat": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/chat",
|
|
"componentAnalysisReport": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/componentAnalysisReport",
|
|
"cwe": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/cwe",
|
|
"documentation": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/documentation",
|
|
"dynamicAnalysisReport": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/dynamicAnalysisReport",
|
|
"eolNotice": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/eolNotice",
|
|
"exportControlAssessment": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/exportControlAssessment",
|
|
"funding": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/funding",
|
|
"issueTracker": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/issueTracker",
|
|
"license": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/license",
|
|
"mailingList": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/mailingList",
|
|
"mavenCentral": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/mavenCentral",
|
|
"metrics": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/metrics",
|
|
"npm": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/npm",
|
|
"nuget": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/nuget",
|
|
"other": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/other",
|
|
"privacyAssessment": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/privacyAssessment",
|
|
"productMetadata": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/productMetadata",
|
|
"purchaseOrder": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/purchaseOrder",
|
|
"qualityAssessmentReport": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/qualityAssessmentReport",
|
|
"releaseHistory": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/releaseHistory",
|
|
"releaseNotes": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/releaseNotes",
|
|
"riskAssessment": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/riskAssessment",
|
|
"runtimeAnalysisReport": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/runtimeAnalysisReport",
|
|
"secureSoftwareAttestation": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/secureSoftwareAttestation",
|
|
"securityAdversaryModel": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityAdversaryModel",
|
|
"securityAdvisory": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityAdvisory",
|
|
"securityFix": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityFix",
|
|
"securityOther": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityOther",
|
|
"securityPenTestReport": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityPenTestReport",
|
|
"securityPolicy": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityPolicy",
|
|
"securityThreatModel": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityThreatModel",
|
|
"socialMedia": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/socialMedia",
|
|
"sourceArtifact": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/sourceArtifact",
|
|
"staticAnalysisReport": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/staticAnalysisReport",
|
|
"support": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/support",
|
|
"vcs": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/vcs",
|
|
"vulnerabilityDisclosureReport": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/vulnerabilityDisclosureReport",
|
|
"vulnerabilityExploitabilityAssessment": "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/vulnerabilityExploitabilityAssessment",
|
|
}
|
|
# A reference to an alternative download location.
|
|
altDownloadLocation = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/altDownloadLocation"
|
|
# A reference to an alternative web page.
|
|
altWebPage = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/altWebPage"
|
|
# A reference to binary artifacts related to a package.
|
|
binaryArtifact = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/binaryArtifact"
|
|
# A reference to a Bower package. The package locator format, looks like `package#version`, is defined in the "install" section of [Bower API documentation](https://bower.io/docs/api/#install).
|
|
bower = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/bower"
|
|
# A reference build metadata related to a published package.
|
|
buildMeta = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/buildMeta"
|
|
# A reference build system used to create or publish the package.
|
|
buildSystem = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/buildSystem"
|
|
# A reference to a certification report for a package from an accredited/independent body.
|
|
certificationReport = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/certificationReport"
|
|
# A reference to the instant messaging system used by the maintainer for a package.
|
|
chat = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/chat"
|
|
# A reference to a Software Composition Analysis (SCA) report.
|
|
componentAnalysisReport = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/componentAnalysisReport"
|
|
# [Common Weakness Enumeration](https://csrc.nist.gov/glossary/term/common_weakness_enumeration). A reference to a source of software flaw defined within the official [CWE List](https://cwe.mitre.org/data/) that conforms to the [CWE specification](https://cwe.mitre.org/).
|
|
cwe = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/cwe"
|
|
# A reference to the documentation for a package.
|
|
documentation = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/documentation"
|
|
# A reference to a dynamic analysis report for a package.
|
|
dynamicAnalysisReport = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/dynamicAnalysisReport"
|
|
# A reference to the End Of Sale (EOS) and/or End Of Life (EOL) information related to a package.
|
|
eolNotice = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/eolNotice"
|
|
# A reference to a export control assessment for a package.
|
|
exportControlAssessment = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/exportControlAssessment"
|
|
# A reference to funding information related to a package.
|
|
funding = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/funding"
|
|
# A reference to the issue tracker for a package.
|
|
issueTracker = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/issueTracker"
|
|
# A reference to additional license information related to an artifact.
|
|
license = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/license"
|
|
# A reference to the mailing list used by the maintainer for a package.
|
|
mailingList = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/mailingList"
|
|
# A reference to a Maven repository artifact. The artifact locator format is defined in the [Maven documentation](https://maven.apache.org/guides/mini/guide-naming-conventions.html) and looks like `groupId:artifactId[:version]`.
|
|
mavenCentral = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/mavenCentral"
|
|
# A reference to metrics related to package such as OpenSSF scorecards.
|
|
metrics = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/metrics"
|
|
# A reference to an npm package. The package locator format is defined in the [npm documentation](https://docs.npmjs.com/cli/v10/configuring-npm/package-json) and looks like `package@version`.
|
|
npm = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/npm"
|
|
# A reference to a NuGet package. The package locator format is defined in the [NuGet documentation](https://docs.nuget.org) and looks like `package/version`.
|
|
nuget = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/nuget"
|
|
# Used when the type does not match any of the other options.
|
|
other = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/other"
|
|
# A reference to a privacy assessment for a package.
|
|
privacyAssessment = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/privacyAssessment"
|
|
# A reference to additional product metadata such as reference within organization's product catalog.
|
|
productMetadata = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/productMetadata"
|
|
# A reference to a purchase order for a package.
|
|
purchaseOrder = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/purchaseOrder"
|
|
# A reference to a quality assessment for a package.
|
|
qualityAssessmentReport = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/qualityAssessmentReport"
|
|
# A reference to a published list of releases for a package.
|
|
releaseHistory = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/releaseHistory"
|
|
# A reference to the release notes for a package.
|
|
releaseNotes = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/releaseNotes"
|
|
# A reference to a risk assessment for a package.
|
|
riskAssessment = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/riskAssessment"
|
|
# A reference to a runtime analysis report for a package.
|
|
runtimeAnalysisReport = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/runtimeAnalysisReport"
|
|
# A reference to information assuring that the software is developed using security practices as defined by [NIST SP 800-218 Secure Software Development Framework (SSDF) Version 1.1](https://csrc.nist.gov/pubs/sp/800/218/final) or [CISA Secure Software Development Attestation Form](https://www.cisa.gov/resources-tools/resources/secure-software-development-attestation-form).
|
|
secureSoftwareAttestation = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/secureSoftwareAttestation"
|
|
# A reference to the security adversary model for a package.
|
|
securityAdversaryModel = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityAdversaryModel"
|
|
# A reference to a published security advisory (where advisory as defined per [ISO 29147:2018](https://www.iso.org/standard/72311.html)) that may affect one or more elements, e.g., vendor advisories or specific NVD entries.
|
|
securityAdvisory = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityAdvisory"
|
|
# A reference to the patch or source code that fixes a vulnerability.
|
|
securityFix = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityFix"
|
|
# A reference to related security information of unspecified type.
|
|
securityOther = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityOther"
|
|
# A reference to a [penetration test](https://en.wikipedia.org/wiki/Penetration_test) report for a package.
|
|
securityPenTestReport = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityPenTestReport"
|
|
# A reference to instructions for reporting newly discovered security vulnerabilities for a package.
|
|
securityPolicy = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityPolicy"
|
|
# A reference the [security threat model](https://en.wikipedia.org/wiki/Threat_model) for a package.
|
|
securityThreatModel = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/securityThreatModel"
|
|
# A reference to a social media channel for a package.
|
|
socialMedia = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/socialMedia"
|
|
# A reference to an artifact containing the sources for a package.
|
|
sourceArtifact = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/sourceArtifact"
|
|
# A reference to a static analysis report for a package.
|
|
staticAnalysisReport = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/staticAnalysisReport"
|
|
# A reference to the software support channel or other support information for a package.
|
|
support = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/support"
|
|
# A reference to a version control system related to a software artifact.
|
|
vcs = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/vcs"
|
|
# A reference to a Vulnerability Disclosure Report (VDR) which provides the software supplier's analysis and findings describing the impact (or lack of impact) that reported vulnerabilities have on packages or products in the supplier's SBOM as defined in [NIST SP 800-161 Cybersecurity Supply Chain Risk Management Practices for Systems and Organizations](https://csrc.nist.gov/pubs/sp/800/161/r1/final).
|
|
vulnerabilityDisclosureReport = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/vulnerabilityDisclosureReport"
|
|
# A reference to a Vulnerability Exploitability eXchange (VEX) statement which provides information on whether a product is impacted by a specific vulnerability in an included package and, if affected, whether there are actions recommended to remediate. See also [NTIA VEX one-page summary](https://ntia.gov/files/ntia/publications/vex_one-page_summary.pdf).
|
|
vulnerabilityExploitabilityAssessment = "https://spdx.org/rdf/3.0.1/terms/Core/ExternalRefType/vulnerabilityExploitabilityAssessment"
|
|
|
|
|
|
# A mathematical algorithm that maps data of arbitrary size to a bit string.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm", compact_type="HashAlgorithm", abstract=False)
|
|
class HashAlgorithm(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"adler32": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/adler32",
|
|
"blake2b256": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake2b256",
|
|
"blake2b384": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake2b384",
|
|
"blake2b512": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake2b512",
|
|
"blake3": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake3",
|
|
"crystalsDilithium": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/crystalsDilithium",
|
|
"crystalsKyber": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/crystalsKyber",
|
|
"falcon": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/falcon",
|
|
"md2": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md2",
|
|
"md4": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md4",
|
|
"md5": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md5",
|
|
"md6": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md6",
|
|
"other": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/other",
|
|
"sha1": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha1",
|
|
"sha224": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha224",
|
|
"sha256": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha256",
|
|
"sha384": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha384",
|
|
"sha3_224": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_224",
|
|
"sha3_256": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_256",
|
|
"sha3_384": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_384",
|
|
"sha3_512": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_512",
|
|
"sha512": "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha512",
|
|
}
|
|
# Adler-32 checksum is part of the widely used zlib compression library as defined in [RFC 1950](https://datatracker.ietf.org/doc/rfc1950/) Section 2.3.
|
|
adler32 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/adler32"
|
|
# BLAKE2b algorithm with a digest size of 256, as defined in [RFC 7693](https://datatracker.ietf.org/doc/rfc7693/) Section 4.
|
|
blake2b256 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake2b256"
|
|
# BLAKE2b algorithm with a digest size of 384, as defined in [RFC 7693](https://datatracker.ietf.org/doc/rfc7693/) Section 4.
|
|
blake2b384 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake2b384"
|
|
# BLAKE2b algorithm with a digest size of 512, as defined in [RFC 7693](https://datatracker.ietf.org/doc/rfc7693/) Section 4.
|
|
blake2b512 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake2b512"
|
|
# [BLAKE3](https://github.com/BLAKE3-team/BLAKE3-specs/blob/master/blake3.pdf)
|
|
blake3 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake3"
|
|
# [Dilithium](https://pq-crystals.org/dilithium/)
|
|
crystalsDilithium = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/crystalsDilithium"
|
|
# [Kyber](https://pq-crystals.org/kyber/)
|
|
crystalsKyber = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/crystalsKyber"
|
|
# [FALCON](https://falcon-sign.info/falcon.pdf)
|
|
falcon = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/falcon"
|
|
# MD2 message-digest algorithm, as defined in [RFC 1319](https://datatracker.ietf.org/doc/rfc1319/).
|
|
md2 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md2"
|
|
# MD4 message-digest algorithm, as defined in [RFC 1186](https://datatracker.ietf.org/doc/rfc1186/).
|
|
md4 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md4"
|
|
# MD5 message-digest algorithm, as defined in [RFC 1321](https://datatracker.ietf.org/doc/rfc1321/).
|
|
md5 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md5"
|
|
# [MD6 hash function](https://people.csail.mit.edu/rivest/pubs/RABCx08.pdf)
|
|
md6 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md6"
|
|
# any hashing algorithm that does not exist in this list of entries
|
|
other = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/other"
|
|
# SHA-1, a secure hashing algorithm, as defined in [RFC 3174](https://datatracker.ietf.org/doc/rfc3174/).
|
|
sha1 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha1"
|
|
# SHA-2 with a digest length of 224, as defined in [RFC 3874](https://datatracker.ietf.org/doc/rfc3874/).
|
|
sha224 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha224"
|
|
# SHA-2 with a digest length of 256, as defined in [RFC 6234](https://datatracker.ietf.org/doc/rfc6234/).
|
|
sha256 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha256"
|
|
# SHA-2 with a digest length of 384, as defined in [RFC 6234](https://datatracker.ietf.org/doc/rfc6234/).
|
|
sha384 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha384"
|
|
# SHA-3 with a digest length of 224, as defined in [FIPS 202](https://csrc.nist.gov/pubs/fips/202/final).
|
|
sha3_224 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_224"
|
|
# SHA-3 with a digest length of 256, as defined in [FIPS 202](https://csrc.nist.gov/pubs/fips/202/final).
|
|
sha3_256 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_256"
|
|
# SHA-3 with a digest length of 384, as defined in [FIPS 202](https://csrc.nist.gov/pubs/fips/202/final).
|
|
sha3_384 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_384"
|
|
# SHA-3 with a digest length of 512, as defined in [FIPS 202](https://csrc.nist.gov/pubs/fips/202/final).
|
|
sha3_512 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_512"
|
|
# SHA-2 with a digest length of 512, as defined in [RFC 6234](https://datatracker.ietf.org/doc/rfc6234/).
|
|
sha512 = "https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha512"
|
|
|
|
|
|
# A concrete subclass of Element used by Individuals in the
|
|
# Core profile.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/IndividualElement", compact_type="IndividualElement", abstract=False)
|
|
class IndividualElement(Element):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
"NoAssertionElement": "https://spdx.org/rdf/3.0.1/terms/Core/NoAssertionElement",
|
|
"NoneElement": "https://spdx.org/rdf/3.0.1/terms/Core/NoneElement",
|
|
}
|
|
# An Individual Value for Element representing a set of Elements of unknown
|
|
# identify or cardinality (number).
|
|
NoAssertionElement = "https://spdx.org/rdf/3.0.1/terms/Core/NoAssertionElement"
|
|
# An Individual Value for Element representing a set of Elements with
|
|
# cardinality (number/count) of zero.
|
|
NoneElement = "https://spdx.org/rdf/3.0.1/terms/Core/NoneElement"
|
|
|
|
|
|
# Provides an independently reproducible mechanism that permits verification of a specific Element.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/IntegrityMethod", compact_type="IntegrityMethod", abstract=True)
|
|
class IntegrityMethod(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provide consumers with comments by the creator of the Element about the
|
|
# Element.
|
|
cls._add_property(
|
|
"comment",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/comment",
|
|
compact="comment",
|
|
)
|
|
|
|
|
|
# Provide an enumerated set of lifecycle phases that can provide context to relationships.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType", compact_type="LifecycleScopeType", abstract=False)
|
|
class LifecycleScopeType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"build": "https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/build",
|
|
"design": "https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/design",
|
|
"development": "https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/development",
|
|
"other": "https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/other",
|
|
"runtime": "https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/runtime",
|
|
"test": "https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/test",
|
|
}
|
|
# A relationship has specific context implications during an element's build phase, during development.
|
|
build = "https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/build"
|
|
# A relationship has specific context implications during an element's design.
|
|
design = "https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/design"
|
|
# A relationship has specific context implications during development phase of an element.
|
|
development = "https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/development"
|
|
# A relationship has other specific context information necessary to capture that the above set of enumerations does not handle.
|
|
other = "https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/other"
|
|
# A relationship has specific context implications during the execution phase of an element.
|
|
runtime = "https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/runtime"
|
|
# A relationship has specific context implications during an element's testing phase, during development.
|
|
test = "https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/test"
|
|
|
|
|
|
# A mapping between prefixes and namespace partial URIs.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/NamespaceMap", compact_type="NamespaceMap", abstract=False)
|
|
class NamespaceMap(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provides an unambiguous mechanism for conveying a URI fragment portion of an
|
|
# Element ID.
|
|
cls._add_property(
|
|
"namespace",
|
|
AnyURIProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/namespace",
|
|
min_count=1,
|
|
compact="namespace",
|
|
)
|
|
# A substitute for a URI.
|
|
cls._add_property(
|
|
"prefix",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/prefix",
|
|
min_count=1,
|
|
compact="prefix",
|
|
)
|
|
|
|
|
|
# An SPDX version 2.X compatible verification method for software packages.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/PackageVerificationCode", compact_type="PackageVerificationCode", abstract=False)
|
|
class PackageVerificationCode(IntegrityMethod):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Specifies the algorithm used for calculating the hash value.
|
|
cls._add_property(
|
|
"algorithm",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/adler32", "adler32"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake2b256", "blake2b256"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake2b384", "blake2b384"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake2b512", "blake2b512"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake3", "blake3"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/crystalsDilithium", "crystalsDilithium"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/crystalsKyber", "crystalsKyber"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/falcon", "falcon"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md2", "md2"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md4", "md4"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md5", "md5"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md6", "md6"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/other", "other"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha1", "sha1"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha224", "sha224"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha256", "sha256"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha384", "sha384"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_224", "sha3_224"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_256", "sha3_256"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_384", "sha3_384"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_512", "sha3_512"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha512", "sha512"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/algorithm",
|
|
min_count=1,
|
|
compact="algorithm",
|
|
)
|
|
# The result of applying a hash algorithm to an Element.
|
|
cls._add_property(
|
|
"hashValue",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/hashValue",
|
|
min_count=1,
|
|
compact="hashValue",
|
|
)
|
|
# The relative file name of a file to be excluded from the
|
|
# `PackageVerificationCode`.
|
|
cls._add_property(
|
|
"packageVerificationCodeExcludedFile",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/packageVerificationCodeExcludedFile",
|
|
compact="packageVerificationCodeExcludedFile",
|
|
)
|
|
|
|
|
|
# A tuple of two positive integers that define a range.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/PositiveIntegerRange", compact_type="PositiveIntegerRange", abstract=False)
|
|
class PositiveIntegerRange(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Defines the beginning of a range.
|
|
cls._add_property(
|
|
"beginIntegerRange",
|
|
PositiveIntegerProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/beginIntegerRange",
|
|
min_count=1,
|
|
compact="beginIntegerRange",
|
|
)
|
|
# Defines the end of a range.
|
|
cls._add_property(
|
|
"endIntegerRange",
|
|
PositiveIntegerProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/endIntegerRange",
|
|
min_count=1,
|
|
compact="endIntegerRange",
|
|
)
|
|
|
|
|
|
# Categories of presence or absence.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/PresenceType", compact_type="PresenceType", abstract=False)
|
|
class PresenceType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"no": "https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/no",
|
|
"noAssertion": "https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/noAssertion",
|
|
"yes": "https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/yes",
|
|
}
|
|
# Indicates absence of the field.
|
|
no = "https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/no"
|
|
# Makes no assertion about the field.
|
|
noAssertion = "https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/noAssertion"
|
|
# Indicates presence of the field.
|
|
yes = "https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/yes"
|
|
|
|
|
|
# Enumeration of the valid profiles.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType", compact_type="ProfileIdentifierType", abstract=False)
|
|
class ProfileIdentifierType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"ai": "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/ai",
|
|
"build": "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/build",
|
|
"core": "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/core",
|
|
"dataset": "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/dataset",
|
|
"expandedLicensing": "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/expandedLicensing",
|
|
"extension": "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/extension",
|
|
"lite": "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/lite",
|
|
"security": "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/security",
|
|
"simpleLicensing": "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/simpleLicensing",
|
|
"software": "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/software",
|
|
}
|
|
# the element follows the AI profile specification
|
|
ai = "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/ai"
|
|
# the element follows the Build profile specification
|
|
build = "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/build"
|
|
# the element follows the Core profile specification
|
|
core = "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/core"
|
|
# the element follows the Dataset profile specification
|
|
dataset = "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/dataset"
|
|
# the element follows the ExpandedLicensing profile specification
|
|
expandedLicensing = "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/expandedLicensing"
|
|
# the element follows the Extension profile specification
|
|
extension = "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/extension"
|
|
# the element follows the Lite profile specification
|
|
lite = "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/lite"
|
|
# the element follows the Security profile specification
|
|
security = "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/security"
|
|
# the element follows the SimpleLicensing profile specification
|
|
simpleLicensing = "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/simpleLicensing"
|
|
# the element follows the Software profile specification
|
|
software = "https://spdx.org/rdf/3.0.1/terms/Core/ProfileIdentifierType/software"
|
|
|
|
|
|
# Describes a relationship between one or more elements.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/Relationship", compact_type="Relationship", abstract=False)
|
|
class Relationship(Element):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provides information about the completeness of relationships.
|
|
cls._add_property(
|
|
"completeness",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipCompleteness/complete", "complete"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipCompleteness/incomplete", "incomplete"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipCompleteness/noAssertion", "noAssertion"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/completeness",
|
|
compact="completeness",
|
|
)
|
|
# Specifies the time from which an element is no longer applicable / valid.
|
|
cls._add_property(
|
|
"endTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/endTime",
|
|
compact="endTime",
|
|
)
|
|
# References the Element on the left-hand side of a relationship.
|
|
cls._add_property(
|
|
"from_",
|
|
ObjectProp(Element, True, context=[
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/NoneElement", "NoneElement"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoAssertionLicense", "expandedlicensing_NoAssertionLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoneLicense", "expandedlicensing_NoneLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SpdxOrganization", "SpdxOrganization"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/NoAssertionElement", "NoAssertionElement"),
|
|
],),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/from",
|
|
min_count=1,
|
|
compact="from",
|
|
)
|
|
# Information about the relationship between two Elements.
|
|
cls._add_property(
|
|
"relationshipType",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/affects", "affects"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/amendedBy", "amendedBy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/ancestorOf", "ancestorOf"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/availableFrom", "availableFrom"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/configures", "configures"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/contains", "contains"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/coordinatedBy", "coordinatedBy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/copiedTo", "copiedTo"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/delegatedTo", "delegatedTo"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/dependsOn", "dependsOn"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/descendantOf", "descendantOf"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/describes", "describes"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/doesNotAffect", "doesNotAffect"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/expandsTo", "expandsTo"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/exploitCreatedBy", "exploitCreatedBy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/fixedBy", "fixedBy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/fixedIn", "fixedIn"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/foundBy", "foundBy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/generates", "generates"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasAddedFile", "hasAddedFile"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasAssessmentFor", "hasAssessmentFor"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasAssociatedVulnerability", "hasAssociatedVulnerability"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasConcludedLicense", "hasConcludedLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDataFile", "hasDataFile"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDeclaredLicense", "hasDeclaredLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDeletedFile", "hasDeletedFile"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDependencyManifest", "hasDependencyManifest"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDistributionArtifact", "hasDistributionArtifact"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDocumentation", "hasDocumentation"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDynamicLink", "hasDynamicLink"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasEvidence", "hasEvidence"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasExample", "hasExample"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasHost", "hasHost"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasInput", "hasInput"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasMetadata", "hasMetadata"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasOptionalComponent", "hasOptionalComponent"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasOptionalDependency", "hasOptionalDependency"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasOutput", "hasOutput"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasPrerequisite", "hasPrerequisite"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasProvidedDependency", "hasProvidedDependency"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasRequirement", "hasRequirement"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasSpecification", "hasSpecification"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasStaticLink", "hasStaticLink"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasTest", "hasTest"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasTestCase", "hasTestCase"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasVariant", "hasVariant"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/invokedBy", "invokedBy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/modifiedBy", "modifiedBy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/other", "other"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/packagedBy", "packagedBy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/patchedBy", "patchedBy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/publishedBy", "publishedBy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/reportedBy", "reportedBy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/republishedBy", "republishedBy"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/serializedInArtifact", "serializedInArtifact"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/testedOn", "testedOn"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/trainedOn", "trainedOn"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/underInvestigationFor", "underInvestigationFor"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/usesTool", "usesTool"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/relationshipType",
|
|
min_count=1,
|
|
compact="relationshipType",
|
|
)
|
|
# Specifies the time from which an element is applicable / valid.
|
|
cls._add_property(
|
|
"startTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/startTime",
|
|
compact="startTime",
|
|
)
|
|
# References an Element on the right-hand side of a relationship.
|
|
cls._add_property(
|
|
"to",
|
|
ListProp(ObjectProp(Element, False, context=[
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/NoneElement", "NoneElement"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoAssertionLicense", "expandedlicensing_NoAssertionLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoneLicense", "expandedlicensing_NoneLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SpdxOrganization", "SpdxOrganization"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/NoAssertionElement", "NoAssertionElement"),
|
|
],)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/to",
|
|
min_count=1,
|
|
compact="to",
|
|
)
|
|
|
|
|
|
# Indicates whether a relationship is known to be complete, incomplete, or if no assertion is made with respect to relationship completeness.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipCompleteness", compact_type="RelationshipCompleteness", abstract=False)
|
|
class RelationshipCompleteness(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"complete": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipCompleteness/complete",
|
|
"incomplete": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipCompleteness/incomplete",
|
|
"noAssertion": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipCompleteness/noAssertion",
|
|
}
|
|
# The relationship is known to be exhaustive.
|
|
complete = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipCompleteness/complete"
|
|
# The relationship is known not to be exhaustive.
|
|
incomplete = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipCompleteness/incomplete"
|
|
# No assertion can be made about the completeness of the relationship.
|
|
noAssertion = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipCompleteness/noAssertion"
|
|
|
|
|
|
# Information about the relationship between two Elements.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType", compact_type="RelationshipType", abstract=False)
|
|
class RelationshipType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"affects": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/affects",
|
|
"amendedBy": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/amendedBy",
|
|
"ancestorOf": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/ancestorOf",
|
|
"availableFrom": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/availableFrom",
|
|
"configures": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/configures",
|
|
"contains": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/contains",
|
|
"coordinatedBy": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/coordinatedBy",
|
|
"copiedTo": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/copiedTo",
|
|
"delegatedTo": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/delegatedTo",
|
|
"dependsOn": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/dependsOn",
|
|
"descendantOf": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/descendantOf",
|
|
"describes": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/describes",
|
|
"doesNotAffect": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/doesNotAffect",
|
|
"expandsTo": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/expandsTo",
|
|
"exploitCreatedBy": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/exploitCreatedBy",
|
|
"fixedBy": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/fixedBy",
|
|
"fixedIn": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/fixedIn",
|
|
"foundBy": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/foundBy",
|
|
"generates": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/generates",
|
|
"hasAddedFile": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasAddedFile",
|
|
"hasAssessmentFor": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasAssessmentFor",
|
|
"hasAssociatedVulnerability": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasAssociatedVulnerability",
|
|
"hasConcludedLicense": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasConcludedLicense",
|
|
"hasDataFile": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDataFile",
|
|
"hasDeclaredLicense": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDeclaredLicense",
|
|
"hasDeletedFile": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDeletedFile",
|
|
"hasDependencyManifest": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDependencyManifest",
|
|
"hasDistributionArtifact": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDistributionArtifact",
|
|
"hasDocumentation": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDocumentation",
|
|
"hasDynamicLink": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDynamicLink",
|
|
"hasEvidence": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasEvidence",
|
|
"hasExample": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasExample",
|
|
"hasHost": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasHost",
|
|
"hasInput": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasInput",
|
|
"hasMetadata": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasMetadata",
|
|
"hasOptionalComponent": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasOptionalComponent",
|
|
"hasOptionalDependency": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasOptionalDependency",
|
|
"hasOutput": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasOutput",
|
|
"hasPrerequisite": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasPrerequisite",
|
|
"hasProvidedDependency": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasProvidedDependency",
|
|
"hasRequirement": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasRequirement",
|
|
"hasSpecification": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasSpecification",
|
|
"hasStaticLink": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasStaticLink",
|
|
"hasTest": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasTest",
|
|
"hasTestCase": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasTestCase",
|
|
"hasVariant": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasVariant",
|
|
"invokedBy": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/invokedBy",
|
|
"modifiedBy": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/modifiedBy",
|
|
"other": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/other",
|
|
"packagedBy": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/packagedBy",
|
|
"patchedBy": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/patchedBy",
|
|
"publishedBy": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/publishedBy",
|
|
"reportedBy": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/reportedBy",
|
|
"republishedBy": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/republishedBy",
|
|
"serializedInArtifact": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/serializedInArtifact",
|
|
"testedOn": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/testedOn",
|
|
"trainedOn": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/trainedOn",
|
|
"underInvestigationFor": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/underInvestigationFor",
|
|
"usesTool": "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/usesTool",
|
|
}
|
|
# The `from` Vulnerability affects each `to` Element. The use of the `affects` type is constrained to `VexAffectedVulnAssessmentRelationship` classed relationships.
|
|
affects = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/affects"
|
|
# The `from` Element is amended by each `to` Element.
|
|
amendedBy = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/amendedBy"
|
|
# The `from` Element is an ancestor of each `to` Element.
|
|
ancestorOf = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/ancestorOf"
|
|
# The `from` Element is available from the additional supplier described by each `to` Element.
|
|
availableFrom = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/availableFrom"
|
|
# The `from` Element is a configuration applied to each `to` Element, during a LifecycleScopeType period.
|
|
configures = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/configures"
|
|
# The `from` Element contains each `to` Element.
|
|
contains = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/contains"
|
|
# The `from` Vulnerability is coordinatedBy the `to` Agent(s) (vendor, researcher, or consumer agent).
|
|
coordinatedBy = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/coordinatedBy"
|
|
# The `from` Element has been copied to each `to` Element.
|
|
copiedTo = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/copiedTo"
|
|
# The `from` Agent is delegating an action to the Agent of the `to` Relationship (which must be of type invokedBy), during a LifecycleScopeType (e.g. the `to` invokedBy Relationship is being done on behalf of `from`).
|
|
delegatedTo = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/delegatedTo"
|
|
# The `from` Element depends on each `to` Element, during a LifecycleScopeType period.
|
|
dependsOn = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/dependsOn"
|
|
# The `from` Element is a descendant of each `to` Element.
|
|
descendantOf = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/descendantOf"
|
|
# The `from` Element describes each `to` Element. To denote the root(s) of a tree of elements in a collection, the rootElement property should be used.
|
|
describes = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/describes"
|
|
# The `from` Vulnerability has no impact on each `to` Element. The use of the `doesNotAffect` is constrained to `VexNotAffectedVulnAssessmentRelationship` classed relationships.
|
|
doesNotAffect = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/doesNotAffect"
|
|
# The `from` archive expands out as an artifact described by each `to` Element.
|
|
expandsTo = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/expandsTo"
|
|
# The `from` Vulnerability has had an exploit created against it by each `to` Agent.
|
|
exploitCreatedBy = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/exploitCreatedBy"
|
|
# Designates a `from` Vulnerability has been fixed by the `to` Agent(s).
|
|
fixedBy = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/fixedBy"
|
|
# A `from` Vulnerability has been fixed in each `to` Element. The use of the `fixedIn` type is constrained to `VexFixedVulnAssessmentRelationship` classed relationships.
|
|
fixedIn = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/fixedIn"
|
|
# Designates a `from` Vulnerability was originally discovered by the `to` Agent(s).
|
|
foundBy = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/foundBy"
|
|
# The `from` Element generates each `to` Element.
|
|
generates = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/generates"
|
|
# Every `to` Element is a file added to the `from` Element (`from` hasAddedFile `to`).
|
|
hasAddedFile = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasAddedFile"
|
|
# Relates a `from` Vulnerability and each `to` Element with a security assessment. To be used with `VulnAssessmentRelationship` types.
|
|
hasAssessmentFor = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasAssessmentFor"
|
|
# Used to associate a `from` Artifact with each `to` Vulnerability.
|
|
hasAssociatedVulnerability = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasAssociatedVulnerability"
|
|
# The `from` SoftwareArtifact is concluded by the SPDX data creator to be governed by each `to` license.
|
|
hasConcludedLicense = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasConcludedLicense"
|
|
# The `from` Element treats each `to` Element as a data file. A data file is an artifact that stores data required or optional for the `from` Element's functionality. A data file can be a database file, an index file, a log file, an AI model file, a calibration data file, a temporary file, a backup file, and more. For AI training dataset, test dataset, test artifact, configuration data, build input data, and build output data, please consider using the more specific relationship types: `trainedOn`, `testedOn`, `hasTest`, `configures`, `hasInput`, and `hasOutput`, respectively. This relationship does not imply dependency.
|
|
hasDataFile = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDataFile"
|
|
# The `from` SoftwareArtifact was discovered to actually contain each `to` license, for example as detected by use of automated tooling.
|
|
hasDeclaredLicense = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDeclaredLicense"
|
|
# Every `to` Element is a file deleted from the `from` Element (`from` hasDeletedFile `to`).
|
|
hasDeletedFile = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDeletedFile"
|
|
# The `from` Element has manifest files that contain dependency information in each `to` Element.
|
|
hasDependencyManifest = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDependencyManifest"
|
|
# The `from` Element is distributed as an artifact in each `to` Element (e.g. an RPM or archive file).
|
|
hasDistributionArtifact = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDistributionArtifact"
|
|
# The `from` Element is documented by each `to` Element.
|
|
hasDocumentation = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDocumentation"
|
|
# The `from` Element dynamically links in each `to` Element, during a LifecycleScopeType period.
|
|
hasDynamicLink = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasDynamicLink"
|
|
# Every `to` Element is considered as evidence for the `from` Element (`from` hasEvidence `to`).
|
|
hasEvidence = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasEvidence"
|
|
# Every `to` Element is an example for the `from` Element (`from` hasExample `to`).
|
|
hasExample = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasExample"
|
|
# The `from` Build was run on the `to` Element during a LifecycleScopeType period (e.g. the host that the build runs on).
|
|
hasHost = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasHost"
|
|
# The `from` Build has each `to` Element as an input, during a LifecycleScopeType period.
|
|
hasInput = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasInput"
|
|
# Every `to` Element is metadata about the `from` Element (`from` hasMetadata `to`).
|
|
hasMetadata = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasMetadata"
|
|
# Every `to` Element is an optional component of the `from` Element (`from` hasOptionalComponent `to`).
|
|
hasOptionalComponent = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasOptionalComponent"
|
|
# The `from` Element optionally depends on each `to` Element, during a LifecycleScopeType period.
|
|
hasOptionalDependency = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasOptionalDependency"
|
|
# The `from` Build element generates each `to` Element as an output, during a LifecycleScopeType period.
|
|
hasOutput = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasOutput"
|
|
# The `from` Element has a prerequisite on each `to` Element, during a LifecycleScopeType period.
|
|
hasPrerequisite = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasPrerequisite"
|
|
# The `from` Element has a dependency on each `to` Element, dependency is not in the distributed artifact, but assumed to be provided, during a LifecycleScopeType period.
|
|
hasProvidedDependency = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasProvidedDependency"
|
|
# The `from` Element has a requirement on each `to` Element, during a LifecycleScopeType period.
|
|
hasRequirement = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasRequirement"
|
|
# Every `to` Element is a specification for the `from` Element (`from` hasSpecification `to`), during a LifecycleScopeType period.
|
|
hasSpecification = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasSpecification"
|
|
# The `from` Element statically links in each `to` Element, during a LifecycleScopeType period.
|
|
hasStaticLink = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasStaticLink"
|
|
# Every `to` Element is a test artifact for the `from` Element (`from` hasTest `to`), during a LifecycleScopeType period.
|
|
hasTest = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasTest"
|
|
# Every `to` Element is a test case for the `from` Element (`from` hasTestCase `to`).
|
|
hasTestCase = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasTestCase"
|
|
# Every `to` Element is a variant the `from` Element (`from` hasVariant `to`).
|
|
hasVariant = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/hasVariant"
|
|
# The `from` Element was invoked by the `to` Agent, during a LifecycleScopeType period (for example, a Build element that describes a build step).
|
|
invokedBy = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/invokedBy"
|
|
# The `from` Element is modified by each `to` Element.
|
|
modifiedBy = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/modifiedBy"
|
|
# Every `to` Element is related to the `from` Element where the relationship type is not described by any of the SPDX relationship types (this relationship is directionless).
|
|
other = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/other"
|
|
# Every `to` Element is a packaged instance of the `from` Element (`from` packagedBy `to`).
|
|
packagedBy = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/packagedBy"
|
|
# Every `to` Element is a patch for the `from` Element (`from` patchedBy `to`).
|
|
patchedBy = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/patchedBy"
|
|
# Designates a `from` Vulnerability was made available for public use or reference by each `to` Agent.
|
|
publishedBy = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/publishedBy"
|
|
# Designates a `from` Vulnerability was first reported to a project, vendor, or tracking database for formal identification by each `to` Agent.
|
|
reportedBy = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/reportedBy"
|
|
# Designates a `from` Vulnerability's details were tracked, aggregated, and/or enriched to improve context (i.e. NVD) by each `to` Agent.
|
|
republishedBy = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/republishedBy"
|
|
# The `from` SpdxDocument can be found in a serialized form in each `to` Artifact.
|
|
serializedInArtifact = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/serializedInArtifact"
|
|
# The `from` Element has been tested on the `to` Element(s).
|
|
testedOn = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/testedOn"
|
|
# The `from` Element has been trained on the `to` Element(s).
|
|
trainedOn = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/trainedOn"
|
|
# The `from` Vulnerability impact is being investigated for each `to` Element. The use of the `underInvestigationFor` type is constrained to `VexUnderInvestigationVulnAssessmentRelationship` classed relationships.
|
|
underInvestigationFor = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/underInvestigationFor"
|
|
# The `from` Element uses each `to` Element as a tool, during a LifecycleScopeType period.
|
|
usesTool = "https://spdx.org/rdf/3.0.1/terms/Core/RelationshipType/usesTool"
|
|
|
|
|
|
# A collection of SPDX Elements that could potentially be serialized.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/SpdxDocument", compact_type="SpdxDocument", abstract=False)
|
|
class SpdxDocument(ElementCollection):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provides the license under which the SPDX documentation of the Element can be
|
|
# used.
|
|
cls._add_property(
|
|
"dataLicense",
|
|
ObjectProp(simplelicensing_AnyLicenseInfo, False, context=[
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoAssertionLicense", "expandedlicensing_NoAssertionLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoneLicense", "expandedlicensing_NoneLicense"),
|
|
],),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/dataLicense",
|
|
compact="dataLicense",
|
|
)
|
|
# Provides an ExternalMap of Element identifiers.
|
|
cls._add_property(
|
|
"import_",
|
|
ListProp(ObjectProp(ExternalMap, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/import",
|
|
compact="import",
|
|
)
|
|
# Provides a NamespaceMap of prefixes and associated namespace partial URIs applicable to an SpdxDocument and independent of any specific serialization format or instance.
|
|
cls._add_property(
|
|
"namespaceMap",
|
|
ListProp(ObjectProp(NamespaceMap, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/namespaceMap",
|
|
compact="namespaceMap",
|
|
)
|
|
|
|
|
|
# Indicates the type of support that is associated with an artifact.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/SupportType", compact_type="SupportType", abstract=False)
|
|
class SupportType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"deployed": "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/deployed",
|
|
"development": "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/development",
|
|
"endOfSupport": "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/endOfSupport",
|
|
"limitedSupport": "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/limitedSupport",
|
|
"noAssertion": "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/noAssertion",
|
|
"noSupport": "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/noSupport",
|
|
"support": "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/support",
|
|
}
|
|
# in addition to being supported by the supplier, the software is known to have been deployed and is in use. For a software as a service provider, this implies the software is now available as a service.
|
|
deployed = "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/deployed"
|
|
# the artifact is in active development and is not considered ready for formal support from the supplier.
|
|
development = "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/development"
|
|
# there is a defined end of support for the artifact from the supplier. This may also be referred to as end of life. There is a validUntilDate that can be used to signal when support ends for the artifact.
|
|
endOfSupport = "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/endOfSupport"
|
|
# the artifact has been released, and there is limited support available from the supplier. There is a validUntilDate that can provide additional information about the duration of support.
|
|
limitedSupport = "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/limitedSupport"
|
|
# no assertion about the type of support is made. This is considered the default if no other support type is used.
|
|
noAssertion = "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/noAssertion"
|
|
# there is no support for the artifact from the supplier, consumer assumes any support obligations.
|
|
noSupport = "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/noSupport"
|
|
# the artifact has been released, and is supported from the supplier. There is a validUntilDate that can provide additional information about the duration of support.
|
|
support = "https://spdx.org/rdf/3.0.1/terms/Core/SupportType/support"
|
|
|
|
|
|
# An element of hardware and/or software utilized to carry out a particular function.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/Tool", compact_type="Tool", abstract=False)
|
|
class Tool(Element):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
|
|
# Categories of confidentiality level.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType", compact_type="dataset_ConfidentialityLevelType", abstract=False)
|
|
class dataset_ConfidentialityLevelType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"amber": "https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType/amber",
|
|
"clear": "https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType/clear",
|
|
"green": "https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType/green",
|
|
"red": "https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType/red",
|
|
}
|
|
# Data points in the dataset can be shared only with specific organizations and their clients on a need to know basis.
|
|
amber = "https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType/amber"
|
|
# Dataset may be distributed freely, without restriction.
|
|
clear = "https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType/clear"
|
|
# Dataset can be shared within a community of peers and partners.
|
|
green = "https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType/green"
|
|
# Data points in the dataset are highly confidential and can only be shared with named recipients.
|
|
red = "https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType/red"
|
|
|
|
|
|
# Availability of dataset.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType", compact_type="dataset_DatasetAvailabilityType", abstract=False)
|
|
class dataset_DatasetAvailabilityType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"clickthrough": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/clickthrough",
|
|
"directDownload": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/directDownload",
|
|
"query": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/query",
|
|
"registration": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/registration",
|
|
"scrapingScript": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/scrapingScript",
|
|
}
|
|
# the dataset is not publicly available and can only be accessed after affirmatively accepting terms on a clickthrough webpage.
|
|
clickthrough = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/clickthrough"
|
|
# the dataset is publicly available and can be downloaded directly.
|
|
directDownload = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/directDownload"
|
|
# the dataset is publicly available, but not all at once, and can only be accessed through queries which return parts of the dataset.
|
|
query = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/query"
|
|
# the dataset is not publicly available and an email registration is required before accessing the dataset, although without an affirmative acceptance of terms.
|
|
registration = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/registration"
|
|
# the dataset provider is not making available the underlying data and the dataset must be reassembled, typically using the provided script for scraping the data.
|
|
scrapingScript = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/scrapingScript"
|
|
|
|
|
|
# Enumeration of dataset types.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType", compact_type="dataset_DatasetType", abstract=False)
|
|
class dataset_DatasetType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"audio": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/audio",
|
|
"categorical": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/categorical",
|
|
"graph": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/graph",
|
|
"image": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/image",
|
|
"noAssertion": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/noAssertion",
|
|
"numeric": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/numeric",
|
|
"other": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/other",
|
|
"sensor": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/sensor",
|
|
"structured": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/structured",
|
|
"syntactic": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/syntactic",
|
|
"text": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/text",
|
|
"timeseries": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/timeseries",
|
|
"timestamp": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/timestamp",
|
|
"video": "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/video",
|
|
}
|
|
# data is audio based, such as a collection of music from the 80s.
|
|
audio = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/audio"
|
|
# data that is classified into a discrete number of categories, such as the eye color of a population of people.
|
|
categorical = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/categorical"
|
|
# data is in the form of a graph where entries are somehow related to each other through edges, such a social network of friends.
|
|
graph = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/graph"
|
|
# data is a collection of images such as pictures of animals.
|
|
image = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/image"
|
|
# data type is not known.
|
|
noAssertion = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/noAssertion"
|
|
# data consists only of numeric entries.
|
|
numeric = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/numeric"
|
|
# data is of a type not included in this list.
|
|
other = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/other"
|
|
# data is recorded from a physical sensor, such as a thermometer reading or biometric device.
|
|
sensor = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/sensor"
|
|
# data is stored in tabular format or retrieved from a relational database.
|
|
structured = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/structured"
|
|
# data describes the syntax or semantics of a language or text, such as a parse tree used for natural language processing.
|
|
syntactic = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/syntactic"
|
|
# data consists of unstructured text, such as a book, Wikipedia article (without images), or transcript.
|
|
text = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/text"
|
|
# data is recorded in an ordered sequence of timestamped entries, such as the price of a stock over the course of a day.
|
|
timeseries = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/timeseries"
|
|
# data is recorded with a timestamp for each entry, but not necessarily ordered or at specific intervals, such as when a taxi ride starts and ends.
|
|
timestamp = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/timestamp"
|
|
# data is video based, such as a collection of movie clips featuring Tom Hanks.
|
|
video = "https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/video"
|
|
|
|
|
|
# Abstract class for additional text intended to be added to a License, but
|
|
# which is not itself a standalone License.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/LicenseAddition", compact_type="expandedlicensing_LicenseAddition", abstract=True)
|
|
class expandedlicensing_LicenseAddition(Element):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Identifies the full text of a LicenseAddition.
|
|
cls._add_property(
|
|
"expandedlicensing_additionText",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/additionText",
|
|
min_count=1,
|
|
compact="expandedlicensing_additionText",
|
|
)
|
|
# Specifies whether an additional text identifier has been marked as deprecated.
|
|
cls._add_property(
|
|
"expandedlicensing_isDeprecatedAdditionId",
|
|
BooleanProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/isDeprecatedAdditionId",
|
|
compact="expandedlicensing_isDeprecatedAdditionId",
|
|
)
|
|
# Identifies all the text and metadata associated with a license in the license
|
|
# XML format.
|
|
cls._add_property(
|
|
"expandedlicensing_licenseXml",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/licenseXml",
|
|
compact="expandedlicensing_licenseXml",
|
|
)
|
|
# Specifies the licenseId that is preferred to be used in place of a deprecated
|
|
# License or LicenseAddition.
|
|
cls._add_property(
|
|
"expandedlicensing_obsoletedBy",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/obsoletedBy",
|
|
compact="expandedlicensing_obsoletedBy",
|
|
)
|
|
# Contains a URL where the License or LicenseAddition can be found in use.
|
|
cls._add_property(
|
|
"expandedlicensing_seeAlso",
|
|
ListProp(AnyURIProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/seeAlso",
|
|
compact="expandedlicensing_seeAlso",
|
|
)
|
|
# Identifies the full text of a LicenseAddition, in SPDX templating format.
|
|
cls._add_property(
|
|
"expandedlicensing_standardAdditionTemplate",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/standardAdditionTemplate",
|
|
compact="expandedlicensing_standardAdditionTemplate",
|
|
)
|
|
|
|
|
|
# A license exception that is listed on the SPDX Exceptions list.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/ListedLicenseException", compact_type="expandedlicensing_ListedLicenseException", abstract=False)
|
|
class expandedlicensing_ListedLicenseException(expandedlicensing_LicenseAddition):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Specifies the SPDX License List version in which this license or exception
|
|
# identifier was deprecated.
|
|
cls._add_property(
|
|
"expandedlicensing_deprecatedVersion",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/deprecatedVersion",
|
|
compact="expandedlicensing_deprecatedVersion",
|
|
)
|
|
# Specifies the SPDX License List version in which this ListedLicense or
|
|
# ListedLicenseException identifier was first added.
|
|
cls._add_property(
|
|
"expandedlicensing_listVersionAdded",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/listVersionAdded",
|
|
compact="expandedlicensing_listVersionAdded",
|
|
)
|
|
|
|
|
|
# A property name with an associated value.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Extension/CdxPropertyEntry", compact_type="extension_CdxPropertyEntry", abstract=False)
|
|
class extension_CdxPropertyEntry(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# A name used in a CdxPropertyEntry name-value pair.
|
|
cls._add_property(
|
|
"extension_cdxPropName",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Extension/cdxPropName",
|
|
min_count=1,
|
|
compact="extension_cdxPropName",
|
|
)
|
|
# A value used in a CdxPropertyEntry name-value pair.
|
|
cls._add_property(
|
|
"extension_cdxPropValue",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Extension/cdxPropValue",
|
|
compact="extension_cdxPropValue",
|
|
)
|
|
|
|
|
|
# A characterization of some aspect of an Element that is associated with the Element in a generalized fashion.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Extension/Extension", compact_type="extension_Extension", abstract=True)
|
|
class extension_Extension(SHACLExtensibleObject, SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
|
|
# Specifies the CVSS base, temporal, threat, or environmental severity type.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType", compact_type="security_CvssSeverityType", abstract=False)
|
|
class security_CvssSeverityType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"critical": "https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/critical",
|
|
"high": "https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/high",
|
|
"low": "https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/low",
|
|
"medium": "https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/medium",
|
|
"none": "https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/none",
|
|
}
|
|
# When a CVSS score is between 9.0 - 10.0
|
|
critical = "https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/critical"
|
|
# When a CVSS score is between 7.0 - 8.9
|
|
high = "https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/high"
|
|
# When a CVSS score is between 0.1 - 3.9
|
|
low = "https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/low"
|
|
# When a CVSS score is between 4.0 - 6.9
|
|
medium = "https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/medium"
|
|
# When a CVSS score is 0.0
|
|
none = "https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/none"
|
|
|
|
|
|
# Specifies the exploit catalog type.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/ExploitCatalogType", compact_type="security_ExploitCatalogType", abstract=False)
|
|
class security_ExploitCatalogType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"kev": "https://spdx.org/rdf/3.0.1/terms/Security/ExploitCatalogType/kev",
|
|
"other": "https://spdx.org/rdf/3.0.1/terms/Security/ExploitCatalogType/other",
|
|
}
|
|
# CISA's Known Exploited Vulnerability (KEV) Catalog
|
|
kev = "https://spdx.org/rdf/3.0.1/terms/Security/ExploitCatalogType/kev"
|
|
# Other exploit catalogs
|
|
other = "https://spdx.org/rdf/3.0.1/terms/Security/ExploitCatalogType/other"
|
|
|
|
|
|
# Specifies the SSVC decision type.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType", compact_type="security_SsvcDecisionType", abstract=False)
|
|
class security_SsvcDecisionType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"act": "https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType/act",
|
|
"attend": "https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType/attend",
|
|
"track": "https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType/track",
|
|
"trackStar": "https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType/trackStar",
|
|
}
|
|
# The vulnerability requires attention from the organization's internal, supervisory-level and leadership-level individuals. Necessary actions include requesting assistance or information about the vulnerability, as well as publishing a notification either internally and/or externally. Typically, internal groups would meet to determine the overall response and then execute agreed upon actions. CISA recommends remediating Act vulnerabilities as soon as possible.
|
|
act = "https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType/act"
|
|
# The vulnerability requires attention from the organization's internal, supervisory-level individuals. Necessary actions include requesting assistance or information about the vulnerability, and may involve publishing a notification either internally and/or externally. CISA recommends remediating Attend vulnerabilities sooner than standard update timelines.
|
|
attend = "https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType/attend"
|
|
# The vulnerability does not require action at this time. The organization would continue to track the vulnerability and reassess it if new information becomes available. CISA recommends remediating Track vulnerabilities within standard update timelines.
|
|
track = "https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType/track"
|
|
# ("Track\*" in the SSVC spec) The vulnerability contains specific characteristics that may require closer monitoring for changes. CISA recommends remediating Track\* vulnerabilities within standard update timelines.
|
|
trackStar = "https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType/trackStar"
|
|
|
|
|
|
# Specifies the VEX justification type.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType", compact_type="security_VexJustificationType", abstract=False)
|
|
class security_VexJustificationType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"componentNotPresent": "https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/componentNotPresent",
|
|
"inlineMitigationsAlreadyExist": "https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/inlineMitigationsAlreadyExist",
|
|
"vulnerableCodeCannotBeControlledByAdversary": "https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/vulnerableCodeCannotBeControlledByAdversary",
|
|
"vulnerableCodeNotInExecutePath": "https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/vulnerableCodeNotInExecutePath",
|
|
"vulnerableCodeNotPresent": "https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/vulnerableCodeNotPresent",
|
|
}
|
|
# The software is not affected because the vulnerable component is not in the product.
|
|
componentNotPresent = "https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/componentNotPresent"
|
|
# Built-in inline controls or mitigations prevent an adversary from leveraging the vulnerability.
|
|
inlineMitigationsAlreadyExist = "https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/inlineMitigationsAlreadyExist"
|
|
# The vulnerable component is present, and the component contains the vulnerable code. However, vulnerable code is used in such a way that an attacker cannot mount any anticipated attack.
|
|
vulnerableCodeCannotBeControlledByAdversary = "https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/vulnerableCodeCannotBeControlledByAdversary"
|
|
# The affected code is not reachable through the execution of the code, including non-anticipated states of the product.
|
|
vulnerableCodeNotInExecutePath = "https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/vulnerableCodeNotInExecutePath"
|
|
# The product is not affected because the code underlying the vulnerability is not present in the product.
|
|
vulnerableCodeNotPresent = "https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/vulnerableCodeNotPresent"
|
|
|
|
|
|
# Abstract ancestor class for all vulnerability assessments
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/VulnAssessmentRelationship", compact_type="security_VulnAssessmentRelationship", abstract=True)
|
|
class security_VulnAssessmentRelationship(Relationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Identifies who or what supplied the artifact or VulnAssessmentRelationship
|
|
# referenced by the Element.
|
|
cls._add_property(
|
|
"suppliedBy",
|
|
ObjectProp(Agent, False, context=[
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SpdxOrganization", "SpdxOrganization"),
|
|
],),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/suppliedBy",
|
|
compact="suppliedBy",
|
|
)
|
|
# Specifies an Element contained in a piece of software where a vulnerability was
|
|
# found.
|
|
cls._add_property(
|
|
"security_assessedElement",
|
|
ObjectProp(software_SoftwareArtifact, False),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/assessedElement",
|
|
compact="security_assessedElement",
|
|
)
|
|
# Specifies a time when a vulnerability assessment was modified
|
|
cls._add_property(
|
|
"security_modifiedTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/modifiedTime",
|
|
compact="security_modifiedTime",
|
|
)
|
|
# Specifies the time when a vulnerability was published.
|
|
cls._add_property(
|
|
"security_publishedTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/publishedTime",
|
|
compact="security_publishedTime",
|
|
)
|
|
# Specified the time and date when a vulnerability was withdrawn.
|
|
cls._add_property(
|
|
"security_withdrawnTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/withdrawnTime",
|
|
compact="security_withdrawnTime",
|
|
)
|
|
|
|
|
|
# Abstract class representing a license combination consisting of one or more licenses.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/SimpleLicensing/AnyLicenseInfo", compact_type="simplelicensing_AnyLicenseInfo", abstract=True)
|
|
class simplelicensing_AnyLicenseInfo(Element):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
|
|
# An SPDX Element containing an SPDX license expression string.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/SimpleLicensing/LicenseExpression", compact_type="simplelicensing_LicenseExpression", abstract=False)
|
|
class simplelicensing_LicenseExpression(simplelicensing_AnyLicenseInfo):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Maps a LicenseRef or AdditionRef string for a Custom License or a Custom
|
|
# License Addition to its URI ID.
|
|
cls._add_property(
|
|
"simplelicensing_customIdToUri",
|
|
ListProp(ObjectProp(DictionaryEntry, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/SimpleLicensing/customIdToUri",
|
|
compact="simplelicensing_customIdToUri",
|
|
)
|
|
# A string in the license expression format.
|
|
cls._add_property(
|
|
"simplelicensing_licenseExpression",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/SimpleLicensing/licenseExpression",
|
|
min_count=1,
|
|
compact="simplelicensing_licenseExpression",
|
|
)
|
|
# The version of the SPDX License List used in the license expression.
|
|
cls._add_property(
|
|
"simplelicensing_licenseListVersion",
|
|
StringProp(pattern=r"^(0|[1-9]\d*)\.(0|[1-9]\d*)\.(0|[1-9]\d*)(?:-((?:0|[1-9]\d*|\d*[a-zA-Z-][0-9a-zA-Z-]*)(?:\.(?:0|[1-9]\d*|\d*[a-zA-Z-][0-9a-zA-Z-]*))*))?(?:\+([0-9a-zA-Z-]+(?:\.[0-9a-zA-Z-]+)*))?$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/SimpleLicensing/licenseListVersion",
|
|
compact="simplelicensing_licenseListVersion",
|
|
)
|
|
|
|
|
|
# A license or addition that is not listed on the SPDX License List.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/SimpleLicensing/SimpleLicensingText", compact_type="simplelicensing_SimpleLicensingText", abstract=False)
|
|
class simplelicensing_SimpleLicensingText(Element):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Identifies the full text of a License or Addition.
|
|
cls._add_property(
|
|
"simplelicensing_licenseText",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/SimpleLicensing/licenseText",
|
|
min_count=1,
|
|
compact="simplelicensing_licenseText",
|
|
)
|
|
|
|
|
|
# A canonical, unique, immutable identifier
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Software/ContentIdentifier", compact_type="software_ContentIdentifier", abstract=False)
|
|
class software_ContentIdentifier(IntegrityMethod):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Specifies the type of the content identifier.
|
|
cls._add_property(
|
|
"software_contentIdentifierType",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/ContentIdentifierType/gitoid", "gitoid"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/ContentIdentifierType/swhid", "swhid"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/contentIdentifierType",
|
|
min_count=1,
|
|
compact="software_contentIdentifierType",
|
|
)
|
|
# Specifies the value of the content identifier.
|
|
cls._add_property(
|
|
"software_contentIdentifierValue",
|
|
AnyURIProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/contentIdentifierValue",
|
|
min_count=1,
|
|
compact="software_contentIdentifierValue",
|
|
)
|
|
|
|
|
|
# Specifies the type of a content identifier.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Software/ContentIdentifierType", compact_type="software_ContentIdentifierType", abstract=False)
|
|
class software_ContentIdentifierType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"gitoid": "https://spdx.org/rdf/3.0.1/terms/Software/ContentIdentifierType/gitoid",
|
|
"swhid": "https://spdx.org/rdf/3.0.1/terms/Software/ContentIdentifierType/swhid",
|
|
}
|
|
# [Gitoid](https://www.iana.org/assignments/uri-schemes/prov/gitoid), stands for [Git Object ID](https://git-scm.com/book/en/v2/Git-Internals-Git-Objects). A gitoid of type blob is a unique hash of a binary artifact. A gitoid may represent either an [Artifact Identifier](https://github.com/omnibor/spec/blob/eb1ee5c961c16215eb8709b2975d193a2007a35d/spec/SPEC.md#artifact-identifier-types) for the software artifact or an [Input Manifest Identifier](https://github.com/omnibor/spec/blob/eb1ee5c961c16215eb8709b2975d193a2007a35d/spec/SPEC.md#input-manifest-identifier) for the software artifact's associated [Artifact Input Manifest](https://github.com/omnibor/spec/blob/eb1ee5c961c16215eb8709b2975d193a2007a35d/spec/SPEC.md#artifact-input-manifest); this ambiguity exists because the Artifact Input Manifest is itself an artifact, and the gitoid of that artifact is its valid identifier. Gitoids calculated on software artifacts (Snippet, File, or Package Elements) should be recorded in the SPDX 3.0 SoftwareArtifact's contentIdentifier property. Gitoids calculated on the Artifact Input Manifest (Input Manifest Identifier) should be recorded in the SPDX 3.0 Element's externalIdentifier property. See [OmniBOR Specification](https://github.com/omnibor/spec/), a minimalistic specification for describing software [Artifact Dependency Graphs](https://github.com/omnibor/spec/blob/eb1ee5c961c16215eb8709b2975d193a2007a35d/spec/SPEC.md#artifact-dependency-graph-adg).
|
|
gitoid = "https://spdx.org/rdf/3.0.1/terms/Software/ContentIdentifierType/gitoid"
|
|
# SoftWare Hash IDentifier, a persistent intrinsic identifier for digital artifacts, such as files, trees (also known as directories or folders), commits, and other objects typically found in version control systems. The format of the identifiers is defined in the [SWHID specification](https://www.swhid.org/specification/v1.1/4.Syntax) (ISO/IEC DIS 18670). They typically look like `swh:1:cnt:94a9ed024d3859793618152ea559a168bbcbb5e2`.
|
|
swhid = "https://spdx.org/rdf/3.0.1/terms/Software/ContentIdentifierType/swhid"
|
|
|
|
|
|
# Enumeration of the different kinds of SPDX file.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Software/FileKindType", compact_type="software_FileKindType", abstract=False)
|
|
class software_FileKindType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"directory": "https://spdx.org/rdf/3.0.1/terms/Software/FileKindType/directory",
|
|
"file": "https://spdx.org/rdf/3.0.1/terms/Software/FileKindType/file",
|
|
}
|
|
# The file represents a directory and all content stored in that directory.
|
|
directory = "https://spdx.org/rdf/3.0.1/terms/Software/FileKindType/directory"
|
|
# The file represents a single file (default).
|
|
file = "https://spdx.org/rdf/3.0.1/terms/Software/FileKindType/file"
|
|
|
|
|
|
# Provides a set of values to be used to describe the common types of SBOMs that
|
|
# tools may create.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Software/SbomType", compact_type="software_SbomType", abstract=False)
|
|
class software_SbomType(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"analyzed": "https://spdx.org/rdf/3.0.1/terms/Software/SbomType/analyzed",
|
|
"build": "https://spdx.org/rdf/3.0.1/terms/Software/SbomType/build",
|
|
"deployed": "https://spdx.org/rdf/3.0.1/terms/Software/SbomType/deployed",
|
|
"design": "https://spdx.org/rdf/3.0.1/terms/Software/SbomType/design",
|
|
"runtime": "https://spdx.org/rdf/3.0.1/terms/Software/SbomType/runtime",
|
|
"source": "https://spdx.org/rdf/3.0.1/terms/Software/SbomType/source",
|
|
}
|
|
# SBOM generated through analysis of artifacts (e.g., executables, packages, containers, and virtual machine images) after its build. Such analysis generally requires a variety of heuristics. In some contexts, this may also be referred to as a "3rd party" SBOM.
|
|
analyzed = "https://spdx.org/rdf/3.0.1/terms/Software/SbomType/analyzed"
|
|
# SBOM generated as part of the process of building the software to create a releasable artifact (e.g., executable or package) from data such as source files, dependencies, built components, build process ephemeral data, and other SBOMs.
|
|
build = "https://spdx.org/rdf/3.0.1/terms/Software/SbomType/build"
|
|
# SBOM provides an inventory of software that is present on a system. This may be an assembly of other SBOMs that combines analysis of configuration options, and examination of execution behavior in a (potentially simulated) deployment environment.
|
|
deployed = "https://spdx.org/rdf/3.0.1/terms/Software/SbomType/deployed"
|
|
# SBOM of intended, planned software project or product with included components (some of which may not yet exist) for a new software artifact.
|
|
design = "https://spdx.org/rdf/3.0.1/terms/Software/SbomType/design"
|
|
# SBOM generated through instrumenting the system running the software, to capture only components present in the system, as well as external call-outs or dynamically loaded components. In some contexts, this may also be referred to as an "Instrumented" or "Dynamic" SBOM.
|
|
runtime = "https://spdx.org/rdf/3.0.1/terms/Software/SbomType/runtime"
|
|
# SBOM created directly from the development environment, source files, and included dependencies used to build an product artifact.
|
|
source = "https://spdx.org/rdf/3.0.1/terms/Software/SbomType/source"
|
|
|
|
|
|
# Provides information about the primary purpose of an Element.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose", compact_type="software_SoftwarePurpose", abstract=False)
|
|
class software_SoftwarePurpose(SHACLObject):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
"application": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/application",
|
|
"archive": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/archive",
|
|
"bom": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/bom",
|
|
"configuration": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/configuration",
|
|
"container": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/container",
|
|
"data": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/data",
|
|
"device": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/device",
|
|
"deviceDriver": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/deviceDriver",
|
|
"diskImage": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/diskImage",
|
|
"documentation": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/documentation",
|
|
"evidence": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/evidence",
|
|
"executable": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/executable",
|
|
"file": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/file",
|
|
"filesystemImage": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/filesystemImage",
|
|
"firmware": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/firmware",
|
|
"framework": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/framework",
|
|
"install": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/install",
|
|
"library": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/library",
|
|
"manifest": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/manifest",
|
|
"model": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/model",
|
|
"module": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/module",
|
|
"operatingSystem": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/operatingSystem",
|
|
"other": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/other",
|
|
"patch": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/patch",
|
|
"platform": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/platform",
|
|
"requirement": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/requirement",
|
|
"source": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/source",
|
|
"specification": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/specification",
|
|
"test": "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/test",
|
|
}
|
|
# The Element is a software application.
|
|
application = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/application"
|
|
# The Element is an archived collection of one or more files (.tar, .zip, etc.).
|
|
archive = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/archive"
|
|
# The Element is a bill of materials.
|
|
bom = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/bom"
|
|
# The Element is configuration data.
|
|
configuration = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/configuration"
|
|
# The Element is a container image which can be used by a container runtime application.
|
|
container = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/container"
|
|
# The Element is data.
|
|
data = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/data"
|
|
# The Element refers to a chipset, processor, or electronic board.
|
|
device = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/device"
|
|
# The Element represents software that controls hardware devices.
|
|
deviceDriver = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/deviceDriver"
|
|
# The Element refers to a disk image that can be written to a disk, booted in a VM, etc. A disk image typically contains most or all of the components necessary to boot, such as bootloaders, kernels, firmware, userspace, etc.
|
|
diskImage = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/diskImage"
|
|
# The Element is documentation.
|
|
documentation = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/documentation"
|
|
# The Element is the evidence that a specification or requirement has been fulfilled.
|
|
evidence = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/evidence"
|
|
# The Element is an Artifact that can be run on a computer.
|
|
executable = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/executable"
|
|
# The Element is a single file which can be independently distributed (configuration file, statically linked binary, Kubernetes deployment, etc.).
|
|
file = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/file"
|
|
# The Element is a file system image that can be written to a disk (or virtual) partition.
|
|
filesystemImage = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/filesystemImage"
|
|
# The Element provides low level control over a device's hardware.
|
|
firmware = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/firmware"
|
|
# The Element is a software framework.
|
|
framework = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/framework"
|
|
# The Element is used to install software on disk.
|
|
install = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/install"
|
|
# The Element is a software library.
|
|
library = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/library"
|
|
# The Element is a software manifest.
|
|
manifest = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/manifest"
|
|
# The Element is a machine learning or artificial intelligence model.
|
|
model = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/model"
|
|
# The Element is a module of a piece of software.
|
|
module = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/module"
|
|
# The Element is an operating system.
|
|
operatingSystem = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/operatingSystem"
|
|
# The Element doesn't fit into any of the other categories.
|
|
other = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/other"
|
|
# The Element contains a set of changes to update, fix, or improve another Element.
|
|
patch = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/patch"
|
|
# The Element represents a runtime environment.
|
|
platform = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/platform"
|
|
# The Element provides a requirement needed as input for another Element.
|
|
requirement = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/requirement"
|
|
# The Element is a single or a collection of source files.
|
|
source = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/source"
|
|
# The Element is a plan, guideline or strategy how to create, perform or analyze an application.
|
|
specification = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/specification"
|
|
# The Element is a test used to verify functionality on an software element.
|
|
test = "https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/test"
|
|
|
|
|
|
# Class that describes a build instance of software/artifacts.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Build/Build", compact_type="build_Build", abstract=False)
|
|
class build_Build(Element):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Property that describes the time at which a build stops.
|
|
cls._add_property(
|
|
"build_buildEndTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Build/buildEndTime",
|
|
compact="build_buildEndTime",
|
|
)
|
|
# A buildId is a locally unique identifier used by a builder to identify a unique
|
|
# instance of a build produced by it.
|
|
cls._add_property(
|
|
"build_buildId",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Build/buildId",
|
|
compact="build_buildId",
|
|
)
|
|
# Property describing the start time of a build.
|
|
cls._add_property(
|
|
"build_buildStartTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Build/buildStartTime",
|
|
compact="build_buildStartTime",
|
|
)
|
|
# A buildType is a hint that is used to indicate the toolchain, platform, or
|
|
# infrastructure that the build was invoked on.
|
|
cls._add_property(
|
|
"build_buildType",
|
|
AnyURIProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Build/buildType",
|
|
min_count=1,
|
|
compact="build_buildType",
|
|
)
|
|
# Property that describes the digest of the build configuration file used to
|
|
# invoke a build.
|
|
cls._add_property(
|
|
"build_configSourceDigest",
|
|
ListProp(ObjectProp(Hash, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Build/configSourceDigest",
|
|
compact="build_configSourceDigest",
|
|
)
|
|
# Property describes the invocation entrypoint of a build.
|
|
cls._add_property(
|
|
"build_configSourceEntrypoint",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Build/configSourceEntrypoint",
|
|
compact="build_configSourceEntrypoint",
|
|
)
|
|
# Property that describes the URI of the build configuration source file.
|
|
cls._add_property(
|
|
"build_configSourceUri",
|
|
ListProp(AnyURIProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Build/configSourceUri",
|
|
compact="build_configSourceUri",
|
|
)
|
|
# Property describing the session in which a build is invoked.
|
|
cls._add_property(
|
|
"build_environment",
|
|
ListProp(ObjectProp(DictionaryEntry, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Build/environment",
|
|
compact="build_environment",
|
|
)
|
|
# Property describing a parameter used in an instance of a build.
|
|
cls._add_property(
|
|
"build_parameter",
|
|
ListProp(ObjectProp(DictionaryEntry, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Build/parameter",
|
|
compact="build_parameter",
|
|
)
|
|
|
|
|
|
# Agent represents anything with the potential to act on a system.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/Agent", compact_type="Agent", abstract=False)
|
|
class Agent(Element):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
|
|
# An assertion made in relation to one or more elements.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/Annotation", compact_type="Annotation", abstract=False)
|
|
class Annotation(Element):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Describes the type of annotation.
|
|
cls._add_property(
|
|
"annotationType",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/AnnotationType/other", "other"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/AnnotationType/review", "review"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/annotationType",
|
|
min_count=1,
|
|
compact="annotationType",
|
|
)
|
|
# Provides information about the content type of an Element or a Property.
|
|
cls._add_property(
|
|
"contentType",
|
|
StringProp(pattern=r"^[^\/]+\/[^\/]+$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/contentType",
|
|
compact="contentType",
|
|
)
|
|
# Commentary on an assertion that an annotator has made.
|
|
cls._add_property(
|
|
"statement",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/statement",
|
|
compact="statement",
|
|
)
|
|
# An Element an annotator has made an assertion about.
|
|
cls._add_property(
|
|
"subject",
|
|
ObjectProp(Element, True, context=[
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/NoneElement", "NoneElement"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoAssertionLicense", "expandedlicensing_NoAssertionLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoneLicense", "expandedlicensing_NoneLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SpdxOrganization", "SpdxOrganization"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/NoAssertionElement", "NoAssertionElement"),
|
|
],),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/subject",
|
|
min_count=1,
|
|
compact="subject",
|
|
)
|
|
|
|
|
|
# A distinct article or unit within the digital domain.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/Artifact", compact_type="Artifact", abstract=True)
|
|
class Artifact(Element):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Specifies the time an artifact was built.
|
|
cls._add_property(
|
|
"builtTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/builtTime",
|
|
compact="builtTime",
|
|
)
|
|
# Identifies from where or whom the Element originally came.
|
|
cls._add_property(
|
|
"originatedBy",
|
|
ListProp(ObjectProp(Agent, False, context=[
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SpdxOrganization", "SpdxOrganization"),
|
|
],)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/originatedBy",
|
|
compact="originatedBy",
|
|
)
|
|
# Specifies the time an artifact was released.
|
|
cls._add_property(
|
|
"releaseTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/releaseTime",
|
|
compact="releaseTime",
|
|
)
|
|
# The name of a relevant standard that may apply to an artifact.
|
|
cls._add_property(
|
|
"standardName",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/standardName",
|
|
compact="standardName",
|
|
)
|
|
# Identifies who or what supplied the artifact or VulnAssessmentRelationship
|
|
# referenced by the Element.
|
|
cls._add_property(
|
|
"suppliedBy",
|
|
ObjectProp(Agent, False, context=[
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SpdxOrganization", "SpdxOrganization"),
|
|
],),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/suppliedBy",
|
|
compact="suppliedBy",
|
|
)
|
|
# Specifies the level of support associated with an artifact.
|
|
cls._add_property(
|
|
"supportLevel",
|
|
ListProp(EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SupportType/deployed", "deployed"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SupportType/development", "development"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SupportType/endOfSupport", "endOfSupport"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SupportType/limitedSupport", "limitedSupport"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SupportType/noAssertion", "noAssertion"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SupportType/noSupport", "noSupport"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/SupportType/support", "support"),
|
|
])),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/supportLevel",
|
|
compact="supportLevel",
|
|
)
|
|
# Specifies until when the artifact can be used before its usage needs to be
|
|
# reassessed.
|
|
cls._add_property(
|
|
"validUntilTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/validUntilTime",
|
|
compact="validUntilTime",
|
|
)
|
|
|
|
|
|
# A collection of Elements that have a shared context.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/Bundle", compact_type="Bundle", abstract=False)
|
|
class Bundle(ElementCollection):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Gives information about the circumstances or unifying properties
|
|
# that Elements of the bundle have been assembled under.
|
|
cls._add_property(
|
|
"context",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/context",
|
|
compact="context",
|
|
)
|
|
|
|
|
|
# A mathematically calculated representation of a grouping of data.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/Hash", compact_type="Hash", abstract=False)
|
|
class Hash(IntegrityMethod):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Specifies the algorithm used for calculating the hash value.
|
|
cls._add_property(
|
|
"algorithm",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/adler32", "adler32"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake2b256", "blake2b256"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake2b384", "blake2b384"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake2b512", "blake2b512"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/blake3", "blake3"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/crystalsDilithium", "crystalsDilithium"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/crystalsKyber", "crystalsKyber"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/falcon", "falcon"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md2", "md2"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md4", "md4"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md5", "md5"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/md6", "md6"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/other", "other"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha1", "sha1"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha224", "sha224"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha256", "sha256"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha384", "sha384"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_224", "sha3_224"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_256", "sha3_256"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_384", "sha3_384"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha3_512", "sha3_512"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/HashAlgorithm/sha512", "sha512"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/algorithm",
|
|
min_count=1,
|
|
compact="algorithm",
|
|
)
|
|
# The result of applying a hash algorithm to an Element.
|
|
cls._add_property(
|
|
"hashValue",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/hashValue",
|
|
min_count=1,
|
|
compact="hashValue",
|
|
)
|
|
|
|
|
|
# Provide context for a relationship that occurs in the lifecycle.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopedRelationship", compact_type="LifecycleScopedRelationship", abstract=False)
|
|
class LifecycleScopedRelationship(Relationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Capture the scope of information about a specific relationship between elements.
|
|
cls._add_property(
|
|
"scope",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/build", "build"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/design", "design"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/development", "development"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/other", "other"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/runtime", "runtime"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/LifecycleScopeType/test", "test"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/scope",
|
|
compact="scope",
|
|
)
|
|
|
|
|
|
# A group of people who work together in an organized way for a shared purpose.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/Organization", compact_type="Organization", abstract=False)
|
|
class Organization(Agent):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
"SpdxOrganization": "https://spdx.org/rdf/3.0.1/terms/Core/SpdxOrganization",
|
|
}
|
|
# An Organization representing the SPDX Project.
|
|
SpdxOrganization = "https://spdx.org/rdf/3.0.1/terms/Core/SpdxOrganization"
|
|
|
|
|
|
# An individual human being.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/Person", compact_type="Person", abstract=False)
|
|
class Person(Agent):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
|
|
# A software agent.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/SoftwareAgent", compact_type="SoftwareAgent", abstract=False)
|
|
class SoftwareAgent(Agent):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
|
|
# Portion of an AnyLicenseInfo representing a set of licensing information
|
|
# where all elements apply.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/ConjunctiveLicenseSet", compact_type="expandedlicensing_ConjunctiveLicenseSet", abstract=False)
|
|
class expandedlicensing_ConjunctiveLicenseSet(simplelicensing_AnyLicenseInfo):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# A license expression participating in a license set.
|
|
cls._add_property(
|
|
"expandedlicensing_member",
|
|
ListProp(ObjectProp(simplelicensing_AnyLicenseInfo, False, context=[
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoAssertionLicense", "expandedlicensing_NoAssertionLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoneLicense", "expandedlicensing_NoneLicense"),
|
|
],)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/member",
|
|
min_count=2,
|
|
compact="expandedlicensing_member",
|
|
)
|
|
|
|
|
|
# A license addition that is not listed on the SPDX Exceptions List.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/CustomLicenseAddition", compact_type="expandedlicensing_CustomLicenseAddition", abstract=False)
|
|
class expandedlicensing_CustomLicenseAddition(expandedlicensing_LicenseAddition):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
|
|
# Portion of an AnyLicenseInfo representing a set of licensing information where
|
|
# only one of the elements applies.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/DisjunctiveLicenseSet", compact_type="expandedlicensing_DisjunctiveLicenseSet", abstract=False)
|
|
class expandedlicensing_DisjunctiveLicenseSet(simplelicensing_AnyLicenseInfo):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# A license expression participating in a license set.
|
|
cls._add_property(
|
|
"expandedlicensing_member",
|
|
ListProp(ObjectProp(simplelicensing_AnyLicenseInfo, False, context=[
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoAssertionLicense", "expandedlicensing_NoAssertionLicense"),
|
|
("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoneLicense", "expandedlicensing_NoneLicense"),
|
|
],)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/member",
|
|
min_count=2,
|
|
compact="expandedlicensing_member",
|
|
)
|
|
|
|
|
|
# Abstract class representing a License or an OrLaterOperator.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/ExtendableLicense", compact_type="expandedlicensing_ExtendableLicense", abstract=True)
|
|
class expandedlicensing_ExtendableLicense(simplelicensing_AnyLicenseInfo):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
|
|
# A concrete subclass of AnyLicenseInfo used by Individuals in the
|
|
# ExpandedLicensing profile.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/IndividualLicensingInfo", compact_type="expandedlicensing_IndividualLicensingInfo", abstract=False)
|
|
class expandedlicensing_IndividualLicensingInfo(simplelicensing_AnyLicenseInfo):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
"NoAssertionLicense": "https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoAssertionLicense",
|
|
"NoneLicense": "https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoneLicense",
|
|
}
|
|
# An Individual Value for License when no assertion can be made about its actual
|
|
# value.
|
|
NoAssertionLicense = "https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoAssertionLicense"
|
|
# An Individual Value for License where the SPDX data creator determines that no
|
|
# license is present.
|
|
NoneLicense = "https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/NoneLicense"
|
|
|
|
|
|
# Abstract class for the portion of an AnyLicenseInfo representing a license.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/License", compact_type="expandedlicensing_License", abstract=True)
|
|
class expandedlicensing_License(expandedlicensing_ExtendableLicense):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Specifies whether a license or additional text identifier has been marked as
|
|
# deprecated.
|
|
cls._add_property(
|
|
"expandedlicensing_isDeprecatedLicenseId",
|
|
BooleanProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/isDeprecatedLicenseId",
|
|
compact="expandedlicensing_isDeprecatedLicenseId",
|
|
)
|
|
# Specifies whether the License is listed as free by the
|
|
# Free Software Foundation (FSF).
|
|
cls._add_property(
|
|
"expandedlicensing_isFsfLibre",
|
|
BooleanProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/isFsfLibre",
|
|
compact="expandedlicensing_isFsfLibre",
|
|
)
|
|
# Specifies whether the License is listed as approved by the
|
|
# Open Source Initiative (OSI).
|
|
cls._add_property(
|
|
"expandedlicensing_isOsiApproved",
|
|
BooleanProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/isOsiApproved",
|
|
compact="expandedlicensing_isOsiApproved",
|
|
)
|
|
# Identifies all the text and metadata associated with a license in the license
|
|
# XML format.
|
|
cls._add_property(
|
|
"expandedlicensing_licenseXml",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/licenseXml",
|
|
compact="expandedlicensing_licenseXml",
|
|
)
|
|
# Specifies the licenseId that is preferred to be used in place of a deprecated
|
|
# License or LicenseAddition.
|
|
cls._add_property(
|
|
"expandedlicensing_obsoletedBy",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/obsoletedBy",
|
|
compact="expandedlicensing_obsoletedBy",
|
|
)
|
|
# Contains a URL where the License or LicenseAddition can be found in use.
|
|
cls._add_property(
|
|
"expandedlicensing_seeAlso",
|
|
ListProp(AnyURIProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/seeAlso",
|
|
compact="expandedlicensing_seeAlso",
|
|
)
|
|
# Provides a License author's preferred text to indicate that a file is covered
|
|
# by the License.
|
|
cls._add_property(
|
|
"expandedlicensing_standardLicenseHeader",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/standardLicenseHeader",
|
|
compact="expandedlicensing_standardLicenseHeader",
|
|
)
|
|
# Identifies the full text of a License, in SPDX templating format.
|
|
cls._add_property(
|
|
"expandedlicensing_standardLicenseTemplate",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/standardLicenseTemplate",
|
|
compact="expandedlicensing_standardLicenseTemplate",
|
|
)
|
|
# Identifies the full text of a License or Addition.
|
|
cls._add_property(
|
|
"simplelicensing_licenseText",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/SimpleLicensing/licenseText",
|
|
min_count=1,
|
|
compact="simplelicensing_licenseText",
|
|
)
|
|
|
|
|
|
# A license that is listed on the SPDX License List.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/ListedLicense", compact_type="expandedlicensing_ListedLicense", abstract=False)
|
|
class expandedlicensing_ListedLicense(expandedlicensing_License):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Specifies the SPDX License List version in which this license or exception
|
|
# identifier was deprecated.
|
|
cls._add_property(
|
|
"expandedlicensing_deprecatedVersion",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/deprecatedVersion",
|
|
compact="expandedlicensing_deprecatedVersion",
|
|
)
|
|
# Specifies the SPDX License List version in which this ListedLicense or
|
|
# ListedLicenseException identifier was first added.
|
|
cls._add_property(
|
|
"expandedlicensing_listVersionAdded",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/listVersionAdded",
|
|
compact="expandedlicensing_listVersionAdded",
|
|
)
|
|
|
|
|
|
# Portion of an AnyLicenseInfo representing this version, or any later version,
|
|
# of the indicated License.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/OrLaterOperator", compact_type="expandedlicensing_OrLaterOperator", abstract=False)
|
|
class expandedlicensing_OrLaterOperator(expandedlicensing_ExtendableLicense):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# A License participating in an 'or later' model.
|
|
cls._add_property(
|
|
"expandedlicensing_subjectLicense",
|
|
ObjectProp(expandedlicensing_License, True),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/subjectLicense",
|
|
min_count=1,
|
|
compact="expandedlicensing_subjectLicense",
|
|
)
|
|
|
|
|
|
# Portion of an AnyLicenseInfo representing a License which has additional
|
|
# text applied to it.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/WithAdditionOperator", compact_type="expandedlicensing_WithAdditionOperator", abstract=False)
|
|
class expandedlicensing_WithAdditionOperator(simplelicensing_AnyLicenseInfo):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# A LicenseAddition participating in a 'with addition' model.
|
|
cls._add_property(
|
|
"expandedlicensing_subjectAddition",
|
|
ObjectProp(expandedlicensing_LicenseAddition, True),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/subjectAddition",
|
|
min_count=1,
|
|
compact="expandedlicensing_subjectAddition",
|
|
)
|
|
# A License participating in a 'with addition' model.
|
|
cls._add_property(
|
|
"expandedlicensing_subjectExtendableLicense",
|
|
ObjectProp(expandedlicensing_ExtendableLicense, True),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/subjectExtendableLicense",
|
|
min_count=1,
|
|
compact="expandedlicensing_subjectExtendableLicense",
|
|
)
|
|
|
|
|
|
# A type of extension consisting of a list of name value pairs.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Extension/CdxPropertiesExtension", compact_type="extension_CdxPropertiesExtension", abstract=False)
|
|
class extension_CdxPropertiesExtension(extension_Extension):
|
|
NODE_KIND = NodeKind.BlankNodeOrIRI
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provides a map of a property names to a values.
|
|
cls._add_property(
|
|
"extension_cdxProperty",
|
|
ListProp(ObjectProp(extension_CdxPropertyEntry, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Extension/cdxProperty",
|
|
min_count=1,
|
|
compact="extension_cdxProperty",
|
|
)
|
|
|
|
|
|
# Provides a CVSS version 2.0 assessment for a vulnerability.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/CvssV2VulnAssessmentRelationship", compact_type="security_CvssV2VulnAssessmentRelationship", abstract=False)
|
|
class security_CvssV2VulnAssessmentRelationship(security_VulnAssessmentRelationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provides a numerical (0-10) representation of the severity of a vulnerability.
|
|
cls._add_property(
|
|
"security_score",
|
|
FloatProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/score",
|
|
min_count=1,
|
|
compact="security_score",
|
|
)
|
|
# Specifies the CVSS vector string for a vulnerability.
|
|
cls._add_property(
|
|
"security_vectorString",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/vectorString",
|
|
min_count=1,
|
|
compact="security_vectorString",
|
|
)
|
|
|
|
|
|
# Provides a CVSS version 3 assessment for a vulnerability.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/CvssV3VulnAssessmentRelationship", compact_type="security_CvssV3VulnAssessmentRelationship", abstract=False)
|
|
class security_CvssV3VulnAssessmentRelationship(security_VulnAssessmentRelationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provides a numerical (0-10) representation of the severity of a vulnerability.
|
|
cls._add_property(
|
|
"security_score",
|
|
FloatProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/score",
|
|
min_count=1,
|
|
compact="security_score",
|
|
)
|
|
# Specifies the CVSS qualitative severity rating of a vulnerability in relation to a piece of software.
|
|
cls._add_property(
|
|
"security_severity",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/critical", "critical"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/high", "high"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/low", "low"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/medium", "medium"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/none", "none"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/severity",
|
|
min_count=1,
|
|
compact="security_severity",
|
|
)
|
|
# Specifies the CVSS vector string for a vulnerability.
|
|
cls._add_property(
|
|
"security_vectorString",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/vectorString",
|
|
min_count=1,
|
|
compact="security_vectorString",
|
|
)
|
|
|
|
|
|
# Provides a CVSS version 4 assessment for a vulnerability.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/CvssV4VulnAssessmentRelationship", compact_type="security_CvssV4VulnAssessmentRelationship", abstract=False)
|
|
class security_CvssV4VulnAssessmentRelationship(security_VulnAssessmentRelationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provides a numerical (0-10) representation of the severity of a vulnerability.
|
|
cls._add_property(
|
|
"security_score",
|
|
FloatProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/score",
|
|
min_count=1,
|
|
compact="security_score",
|
|
)
|
|
# Specifies the CVSS qualitative severity rating of a vulnerability in relation to a piece of software.
|
|
cls._add_property(
|
|
"security_severity",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/critical", "critical"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/high", "high"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/low", "low"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/medium", "medium"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/CvssSeverityType/none", "none"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/severity",
|
|
min_count=1,
|
|
compact="security_severity",
|
|
)
|
|
# Specifies the CVSS vector string for a vulnerability.
|
|
cls._add_property(
|
|
"security_vectorString",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/vectorString",
|
|
min_count=1,
|
|
compact="security_vectorString",
|
|
)
|
|
|
|
|
|
# Provides an EPSS assessment for a vulnerability.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/EpssVulnAssessmentRelationship", compact_type="security_EpssVulnAssessmentRelationship", abstract=False)
|
|
class security_EpssVulnAssessmentRelationship(security_VulnAssessmentRelationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# The percentile of the current probability score.
|
|
cls._add_property(
|
|
"security_percentile",
|
|
FloatProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/percentile",
|
|
min_count=1,
|
|
compact="security_percentile",
|
|
)
|
|
# A probability score between 0 and 1 of a vulnerability being exploited.
|
|
cls._add_property(
|
|
"security_probability",
|
|
FloatProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/probability",
|
|
min_count=1,
|
|
compact="security_probability",
|
|
)
|
|
|
|
|
|
# Provides an exploit assessment of a vulnerability.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/ExploitCatalogVulnAssessmentRelationship", compact_type="security_ExploitCatalogVulnAssessmentRelationship", abstract=False)
|
|
class security_ExploitCatalogVulnAssessmentRelationship(security_VulnAssessmentRelationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Specifies the exploit catalog type.
|
|
cls._add_property(
|
|
"security_catalogType",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/ExploitCatalogType/kev", "kev"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/ExploitCatalogType/other", "other"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/catalogType",
|
|
min_count=1,
|
|
compact="security_catalogType",
|
|
)
|
|
# Describe that a CVE is known to have an exploit because it's been listed in an exploit catalog.
|
|
cls._add_property(
|
|
"security_exploited",
|
|
BooleanProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/exploited",
|
|
min_count=1,
|
|
compact="security_exploited",
|
|
)
|
|
# Provides the location of an exploit catalog.
|
|
cls._add_property(
|
|
"security_locator",
|
|
AnyURIProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/locator",
|
|
min_count=1,
|
|
compact="security_locator",
|
|
)
|
|
|
|
|
|
# Provides an SSVC assessment for a vulnerability.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/SsvcVulnAssessmentRelationship", compact_type="security_SsvcVulnAssessmentRelationship", abstract=False)
|
|
class security_SsvcVulnAssessmentRelationship(security_VulnAssessmentRelationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provide the enumeration of possible decisions in the
|
|
# [Stakeholder-Specific Vulnerability Categorization (SSVC) decision tree](https://www.cisa.gov/stakeholder-specific-vulnerability-categorization-ssvc).
|
|
cls._add_property(
|
|
"security_decisionType",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType/act", "act"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType/attend", "attend"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType/track", "track"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/SsvcDecisionType/trackStar", "trackStar"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/decisionType",
|
|
min_count=1,
|
|
compact="security_decisionType",
|
|
)
|
|
|
|
|
|
# Abstract ancestor class for all VEX relationships
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/VexVulnAssessmentRelationship", compact_type="security_VexVulnAssessmentRelationship", abstract=True)
|
|
class security_VexVulnAssessmentRelationship(security_VulnAssessmentRelationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Conveys information about how VEX status was determined.
|
|
cls._add_property(
|
|
"security_statusNotes",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/statusNotes",
|
|
compact="security_statusNotes",
|
|
)
|
|
# Specifies the version of a VEX statement.
|
|
cls._add_property(
|
|
"security_vexVersion",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/vexVersion",
|
|
compact="security_vexVersion",
|
|
)
|
|
|
|
|
|
# Specifies a vulnerability and its associated information.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/Vulnerability", compact_type="security_Vulnerability", abstract=False)
|
|
class security_Vulnerability(Artifact):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Specifies a time when a vulnerability assessment was modified
|
|
cls._add_property(
|
|
"security_modifiedTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/modifiedTime",
|
|
compact="security_modifiedTime",
|
|
)
|
|
# Specifies the time when a vulnerability was published.
|
|
cls._add_property(
|
|
"security_publishedTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/publishedTime",
|
|
compact="security_publishedTime",
|
|
)
|
|
# Specified the time and date when a vulnerability was withdrawn.
|
|
cls._add_property(
|
|
"security_withdrawnTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/withdrawnTime",
|
|
compact="security_withdrawnTime",
|
|
)
|
|
|
|
|
|
# A distinct article or unit related to Software.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Software/SoftwareArtifact", compact_type="software_SoftwareArtifact", abstract=True)
|
|
class software_SoftwareArtifact(Artifact):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provides additional purpose information of the software artifact.
|
|
cls._add_property(
|
|
"software_additionalPurpose",
|
|
ListProp(EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/application", "application"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/archive", "archive"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/bom", "bom"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/configuration", "configuration"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/container", "container"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/data", "data"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/device", "device"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/deviceDriver", "deviceDriver"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/diskImage", "diskImage"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/documentation", "documentation"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/evidence", "evidence"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/executable", "executable"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/file", "file"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/filesystemImage", "filesystemImage"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/firmware", "firmware"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/framework", "framework"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/install", "install"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/library", "library"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/manifest", "manifest"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/model", "model"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/module", "module"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/operatingSystem", "operatingSystem"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/other", "other"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/patch", "patch"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/platform", "platform"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/requirement", "requirement"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/source", "source"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/specification", "specification"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/test", "test"),
|
|
])),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/additionalPurpose",
|
|
compact="software_additionalPurpose",
|
|
)
|
|
# Provides a place for the SPDX data creator to record acknowledgement text for
|
|
# a software Package, File or Snippet.
|
|
cls._add_property(
|
|
"software_attributionText",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/attributionText",
|
|
compact="software_attributionText",
|
|
)
|
|
# A canonical, unique, immutable identifier of the artifact content, that may be
|
|
# used for verifying its identity and/or integrity.
|
|
cls._add_property(
|
|
"software_contentIdentifier",
|
|
ListProp(ObjectProp(software_ContentIdentifier, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/contentIdentifier",
|
|
compact="software_contentIdentifier",
|
|
)
|
|
# Identifies the text of one or more copyright notices for a software Package,
|
|
# File or Snippet, if any.
|
|
cls._add_property(
|
|
"software_copyrightText",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/copyrightText",
|
|
compact="software_copyrightText",
|
|
)
|
|
# Provides information about the primary purpose of the software artifact.
|
|
cls._add_property(
|
|
"software_primaryPurpose",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/application", "application"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/archive", "archive"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/bom", "bom"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/configuration", "configuration"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/container", "container"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/data", "data"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/device", "device"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/deviceDriver", "deviceDriver"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/diskImage", "diskImage"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/documentation", "documentation"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/evidence", "evidence"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/executable", "executable"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/file", "file"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/filesystemImage", "filesystemImage"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/firmware", "firmware"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/framework", "framework"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/install", "install"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/library", "library"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/manifest", "manifest"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/model", "model"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/module", "module"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/operatingSystem", "operatingSystem"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/other", "other"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/patch", "patch"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/platform", "platform"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/requirement", "requirement"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/source", "source"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/specification", "specification"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SoftwarePurpose/test", "test"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/primaryPurpose",
|
|
compact="software_primaryPurpose",
|
|
)
|
|
|
|
|
|
# A container for a grouping of SPDX-3.0 content characterizing details
|
|
# (provenence, composition, licensing, etc.) about a product.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Core/Bom", compact_type="Bom", abstract=False)
|
|
class Bom(Bundle):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
|
|
# A license that is not listed on the SPDX License List.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/ExpandedLicensing/CustomLicense", compact_type="expandedlicensing_CustomLicense", abstract=False)
|
|
class expandedlicensing_CustomLicense(expandedlicensing_License):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
|
|
# Connects a vulnerability and an element designating the element as a product
|
|
# affected by the vulnerability.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/VexAffectedVulnAssessmentRelationship", compact_type="security_VexAffectedVulnAssessmentRelationship", abstract=False)
|
|
class security_VexAffectedVulnAssessmentRelationship(security_VexVulnAssessmentRelationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provides advise on how to mitigate or remediate a vulnerability when a VEX product
|
|
# is affected by it.
|
|
cls._add_property(
|
|
"security_actionStatement",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/actionStatement",
|
|
min_count=1,
|
|
compact="security_actionStatement",
|
|
)
|
|
# Records the time when a recommended action was communicated in a VEX statement
|
|
# to mitigate a vulnerability.
|
|
cls._add_property(
|
|
"security_actionStatementTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/actionStatementTime",
|
|
compact="security_actionStatementTime",
|
|
)
|
|
|
|
|
|
# Links a vulnerability and elements representing products (in the VEX sense) where
|
|
# a fix has been applied and are no longer affected.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/VexFixedVulnAssessmentRelationship", compact_type="security_VexFixedVulnAssessmentRelationship", abstract=False)
|
|
class security_VexFixedVulnAssessmentRelationship(security_VexVulnAssessmentRelationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
|
|
# Links a vulnerability and one or more elements designating the latter as products
|
|
# not affected by the vulnerability.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/VexNotAffectedVulnAssessmentRelationship", compact_type="security_VexNotAffectedVulnAssessmentRelationship", abstract=False)
|
|
class security_VexNotAffectedVulnAssessmentRelationship(security_VexVulnAssessmentRelationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Explains why a VEX product is not affected by a vulnerability. It is an
|
|
# alternative in VexNotAffectedVulnAssessmentRelationship to the machine-readable
|
|
# justification label.
|
|
cls._add_property(
|
|
"security_impactStatement",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/impactStatement",
|
|
compact="security_impactStatement",
|
|
)
|
|
# Timestamp of impact statement.
|
|
cls._add_property(
|
|
"security_impactStatementTime",
|
|
DateTimeStampProp(pattern=r"^\d\d\d\d-\d\d-\d\dT\d\d:\d\d:\d\dZ$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/impactStatementTime",
|
|
compact="security_impactStatementTime",
|
|
)
|
|
# Impact justification label to be used when linking a vulnerability to an element
|
|
# representing a VEX product with a VexNotAffectedVulnAssessmentRelationship
|
|
# relationship.
|
|
cls._add_property(
|
|
"security_justificationType",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/componentNotPresent", "componentNotPresent"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/inlineMitigationsAlreadyExist", "inlineMitigationsAlreadyExist"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/vulnerableCodeCannotBeControlledByAdversary", "vulnerableCodeCannotBeControlledByAdversary"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/vulnerableCodeNotInExecutePath", "vulnerableCodeNotInExecutePath"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Security/VexJustificationType/vulnerableCodeNotPresent", "vulnerableCodeNotPresent"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Security/justificationType",
|
|
compact="security_justificationType",
|
|
)
|
|
|
|
|
|
# Designates elements as products where the impact of a vulnerability is being
|
|
# investigated.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Security/VexUnderInvestigationVulnAssessmentRelationship", compact_type="security_VexUnderInvestigationVulnAssessmentRelationship", abstract=False)
|
|
class security_VexUnderInvestigationVulnAssessmentRelationship(security_VexVulnAssessmentRelationship):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
|
|
# Refers to any object that stores content on a computer.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Software/File", compact_type="software_File", abstract=False)
|
|
class software_File(software_SoftwareArtifact):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provides information about the content type of an Element or a Property.
|
|
cls._add_property(
|
|
"contentType",
|
|
StringProp(pattern=r"^[^\/]+\/[^\/]+$",),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Core/contentType",
|
|
compact="contentType",
|
|
)
|
|
# Describes if a given file is a directory or non-directory kind of file.
|
|
cls._add_property(
|
|
"software_fileKind",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/FileKindType/directory", "directory"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/FileKindType/file", "file"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/fileKind",
|
|
compact="software_fileKind",
|
|
)
|
|
|
|
|
|
# Refers to any unit of content that can be associated with a distribution of
|
|
# software.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Software/Package", compact_type="software_Package", abstract=False)
|
|
class software_Package(software_SoftwareArtifact):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Identifies the download Uniform Resource Identifier for the package at the time
|
|
# that the document was created.
|
|
cls._add_property(
|
|
"software_downloadLocation",
|
|
AnyURIProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/downloadLocation",
|
|
compact="software_downloadLocation",
|
|
)
|
|
# A place for the SPDX document creator to record a website that serves as the
|
|
# package's home page.
|
|
cls._add_property(
|
|
"software_homePage",
|
|
AnyURIProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/homePage",
|
|
compact="software_homePage",
|
|
)
|
|
# Provides a place for the SPDX data creator to record the package URL string
|
|
# (in accordance with the Package URL specification) for a software Package.
|
|
cls._add_property(
|
|
"software_packageUrl",
|
|
AnyURIProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/packageUrl",
|
|
compact="software_packageUrl",
|
|
)
|
|
# Identify the version of a package.
|
|
cls._add_property(
|
|
"software_packageVersion",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/packageVersion",
|
|
compact="software_packageVersion",
|
|
)
|
|
# Records any relevant background information or additional comments
|
|
# about the origin of the package.
|
|
cls._add_property(
|
|
"software_sourceInfo",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/sourceInfo",
|
|
compact="software_sourceInfo",
|
|
)
|
|
|
|
|
|
# A collection of SPDX Elements describing a single package.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Software/Sbom", compact_type="software_Sbom", abstract=False)
|
|
class software_Sbom(Bom):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Provides information about the type of an SBOM.
|
|
cls._add_property(
|
|
"software_sbomType",
|
|
ListProp(EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SbomType/analyzed", "analyzed"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SbomType/build", "build"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SbomType/deployed", "deployed"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SbomType/design", "design"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SbomType/runtime", "runtime"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Software/SbomType/source", "source"),
|
|
])),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/sbomType",
|
|
compact="software_sbomType",
|
|
)
|
|
|
|
|
|
# Describes a certain part of a file.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Software/Snippet", compact_type="software_Snippet", abstract=False)
|
|
class software_Snippet(software_SoftwareArtifact):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Defines the byte range in the original host file that the snippet information
|
|
# applies to.
|
|
cls._add_property(
|
|
"software_byteRange",
|
|
ObjectProp(PositiveIntegerRange, False),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/byteRange",
|
|
compact="software_byteRange",
|
|
)
|
|
# Defines the line range in the original host file that the snippet information
|
|
# applies to.
|
|
cls._add_property(
|
|
"software_lineRange",
|
|
ObjectProp(PositiveIntegerRange, False),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/lineRange",
|
|
compact="software_lineRange",
|
|
)
|
|
# Defines the original host file that the snippet information applies to.
|
|
cls._add_property(
|
|
"software_snippetFromFile",
|
|
ObjectProp(software_File, True),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Software/snippetFromFile",
|
|
min_count=1,
|
|
compact="software_snippetFromFile",
|
|
)
|
|
|
|
|
|
# Specifies an AI package and its associated information.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/AI/AIPackage", compact_type="ai_AIPackage", abstract=False)
|
|
class ai_AIPackage(software_Package):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Indicates whether the system can perform a decision or action without human
|
|
# involvement or guidance.
|
|
cls._add_property(
|
|
"ai_autonomyType",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/no", "no"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/noAssertion", "noAssertion"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/yes", "yes"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/autonomyType",
|
|
compact="ai_autonomyType",
|
|
)
|
|
# Captures the domain in which the AI package can be used.
|
|
cls._add_property(
|
|
"ai_domain",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/domain",
|
|
compact="ai_domain",
|
|
)
|
|
# Indicates the amount of energy consumption incurred by an AI model.
|
|
cls._add_property(
|
|
"ai_energyConsumption",
|
|
ObjectProp(ai_EnergyConsumption, False),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/energyConsumption",
|
|
compact="ai_energyConsumption",
|
|
)
|
|
# Records a hyperparameter used to build the AI model contained in the AI
|
|
# package.
|
|
cls._add_property(
|
|
"ai_hyperparameter",
|
|
ListProp(ObjectProp(DictionaryEntry, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/hyperparameter",
|
|
compact="ai_hyperparameter",
|
|
)
|
|
# Provides relevant information about the AI software, not including the model
|
|
# description.
|
|
cls._add_property(
|
|
"ai_informationAboutApplication",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/informationAboutApplication",
|
|
compact="ai_informationAboutApplication",
|
|
)
|
|
# Describes relevant information about different steps of the training process.
|
|
cls._add_property(
|
|
"ai_informationAboutTraining",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/informationAboutTraining",
|
|
compact="ai_informationAboutTraining",
|
|
)
|
|
# Captures a limitation of the AI software.
|
|
cls._add_property(
|
|
"ai_limitation",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/limitation",
|
|
compact="ai_limitation",
|
|
)
|
|
# Records the measurement of prediction quality of the AI model.
|
|
cls._add_property(
|
|
"ai_metric",
|
|
ListProp(ObjectProp(DictionaryEntry, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/metric",
|
|
compact="ai_metric",
|
|
)
|
|
# Captures the threshold that was used for computation of a metric described in
|
|
# the metric field.
|
|
cls._add_property(
|
|
"ai_metricDecisionThreshold",
|
|
ListProp(ObjectProp(DictionaryEntry, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/metricDecisionThreshold",
|
|
compact="ai_metricDecisionThreshold",
|
|
)
|
|
# Describes all the preprocessing steps applied to the training data before the
|
|
# model training.
|
|
cls._add_property(
|
|
"ai_modelDataPreprocessing",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/modelDataPreprocessing",
|
|
compact="ai_modelDataPreprocessing",
|
|
)
|
|
# Describes methods that can be used to explain the results from the AI model.
|
|
cls._add_property(
|
|
"ai_modelExplainability",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/modelExplainability",
|
|
compact="ai_modelExplainability",
|
|
)
|
|
# Records the results of general safety risk assessment of the AI system.
|
|
cls._add_property(
|
|
"ai_safetyRiskAssessment",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType/high", "high"),
|
|
("https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType/low", "low"),
|
|
("https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType/medium", "medium"),
|
|
("https://spdx.org/rdf/3.0.1/terms/AI/SafetyRiskAssessmentType/serious", "serious"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/safetyRiskAssessment",
|
|
compact="ai_safetyRiskAssessment",
|
|
)
|
|
# Captures a standard that is being complied with.
|
|
cls._add_property(
|
|
"ai_standardCompliance",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/standardCompliance",
|
|
compact="ai_standardCompliance",
|
|
)
|
|
# Records the type of the model used in the AI software.
|
|
cls._add_property(
|
|
"ai_typeOfModel",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/typeOfModel",
|
|
compact="ai_typeOfModel",
|
|
)
|
|
# Records if sensitive personal information is used during model training or
|
|
# could be used during the inference.
|
|
cls._add_property(
|
|
"ai_useSensitivePersonalInformation",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/no", "no"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/noAssertion", "noAssertion"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/yes", "yes"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/AI/useSensitivePersonalInformation",
|
|
compact="ai_useSensitivePersonalInformation",
|
|
)
|
|
|
|
|
|
# Specifies a data package and its associated information.
|
|
@register("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetPackage", compact_type="dataset_DatasetPackage", abstract=False)
|
|
class dataset_DatasetPackage(software_Package):
|
|
NODE_KIND = NodeKind.IRI
|
|
ID_ALIAS = "spdxId"
|
|
NAMED_INDIVIDUALS = {
|
|
}
|
|
|
|
@classmethod
|
|
def _register_props(cls):
|
|
super()._register_props()
|
|
# Describes the anonymization methods used.
|
|
cls._add_property(
|
|
"dataset_anonymizationMethodUsed",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/anonymizationMethodUsed",
|
|
compact="dataset_anonymizationMethodUsed",
|
|
)
|
|
# Describes the confidentiality level of the data points contained in the dataset.
|
|
cls._add_property(
|
|
"dataset_confidentialityLevel",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType/amber", "amber"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType/clear", "clear"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType/green", "green"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/ConfidentialityLevelType/red", "red"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/confidentialityLevel",
|
|
compact="dataset_confidentialityLevel",
|
|
)
|
|
# Describes how the dataset was collected.
|
|
cls._add_property(
|
|
"dataset_dataCollectionProcess",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/dataCollectionProcess",
|
|
compact="dataset_dataCollectionProcess",
|
|
)
|
|
# Describes the preprocessing steps that were applied to the raw data to create the given dataset.
|
|
cls._add_property(
|
|
"dataset_dataPreprocessing",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/dataPreprocessing",
|
|
compact="dataset_dataPreprocessing",
|
|
)
|
|
# The field describes the availability of a dataset.
|
|
cls._add_property(
|
|
"dataset_datasetAvailability",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/clickthrough", "clickthrough"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/directDownload", "directDownload"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/query", "query"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/registration", "registration"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetAvailabilityType/scrapingScript", "scrapingScript"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/datasetAvailability",
|
|
compact="dataset_datasetAvailability",
|
|
)
|
|
# Describes potentially noisy elements of the dataset.
|
|
cls._add_property(
|
|
"dataset_datasetNoise",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/datasetNoise",
|
|
compact="dataset_datasetNoise",
|
|
)
|
|
# Captures the size of the dataset.
|
|
cls._add_property(
|
|
"dataset_datasetSize",
|
|
NonNegativeIntegerProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/datasetSize",
|
|
compact="dataset_datasetSize",
|
|
)
|
|
# Describes the type of the given dataset.
|
|
cls._add_property(
|
|
"dataset_datasetType",
|
|
ListProp(EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/audio", "audio"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/categorical", "categorical"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/graph", "graph"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/image", "image"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/noAssertion", "noAssertion"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/numeric", "numeric"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/other", "other"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/sensor", "sensor"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/structured", "structured"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/syntactic", "syntactic"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/text", "text"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/timeseries", "timeseries"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/timestamp", "timestamp"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Dataset/DatasetType/video", "video"),
|
|
])),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/datasetType",
|
|
min_count=1,
|
|
compact="dataset_datasetType",
|
|
)
|
|
# Describes a mechanism to update the dataset.
|
|
cls._add_property(
|
|
"dataset_datasetUpdateMechanism",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/datasetUpdateMechanism",
|
|
compact="dataset_datasetUpdateMechanism",
|
|
)
|
|
# Describes if any sensitive personal information is present in the dataset.
|
|
cls._add_property(
|
|
"dataset_hasSensitivePersonalInformation",
|
|
EnumProp([
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/no", "no"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/noAssertion", "noAssertion"),
|
|
("https://spdx.org/rdf/3.0.1/terms/Core/PresenceType/yes", "yes"),
|
|
]),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/hasSensitivePersonalInformation",
|
|
compact="dataset_hasSensitivePersonalInformation",
|
|
)
|
|
# Describes what the given dataset should be used for.
|
|
cls._add_property(
|
|
"dataset_intendedUse",
|
|
StringProp(),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/intendedUse",
|
|
compact="dataset_intendedUse",
|
|
)
|
|
# Records the biases that the dataset is known to encompass.
|
|
cls._add_property(
|
|
"dataset_knownBias",
|
|
ListProp(StringProp()),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/knownBias",
|
|
compact="dataset_knownBias",
|
|
)
|
|
# Describes a sensor used for collecting the data.
|
|
cls._add_property(
|
|
"dataset_sensor",
|
|
ListProp(ObjectProp(DictionaryEntry, False)),
|
|
iri="https://spdx.org/rdf/3.0.1/terms/Dataset/sensor",
|
|
compact="dataset_sensor",
|
|
)
|
|
|
|
|
|
"""Format Guard"""
|
|
# fmt: on
|
|
|
|
|
|
def main():
|
|
import argparse
|
|
from pathlib import Path
|
|
|
|
parser = argparse.ArgumentParser(description="Python SHACL model test")
|
|
parser.add_argument("infile", type=Path, help="Input file")
|
|
parser.add_argument("--print", action="store_true", help="Print object tree")
|
|
parser.add_argument("--outfile", type=Path, help="Output file")
|
|
|
|
args = parser.parse_args()
|
|
|
|
objectset = SHACLObjectSet()
|
|
with args.infile.open("r") as f:
|
|
d = JSONLDDeserializer()
|
|
d.read(f, objectset)
|
|
|
|
if args.print:
|
|
print_tree(objectset.objects)
|
|
|
|
if args.outfile:
|
|
with args.outfile.open("wb") as f:
|
|
s = JSONLDSerializer()
|
|
s.write(objectset, f)
|
|
|
|
return 0
|
|
|
|
|
|
if __name__ == "__main__":
|
|
sys.exit(main())
|