mirror of
https://git.yoctoproject.org/poky-contrib
synced 2025-05-09 08:02:36 +08:00
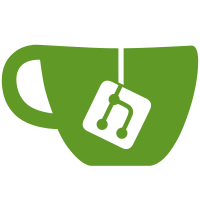
Uses TEST_SERIALCONTROL_CMD to open a serial connection to the target and execute commands. This is a drop in replacement for the ssh target, fully supporting the same API. Supported with testexport. To use, set the following in local.conf: - TEST_TARGET to "serial" - TEST_SERIALCONTROL_CMD to a shell command or script which connects to the serial console of the target and forwards that connection to standard input/output. - TEST_SERIALCONTROL_EXTRA_ARGS (optional) any parameters that must be passed to the serial control command. - TEST_SERIALCONTROL_PS1 (optional) A regex string representing an empty prompt on the target terminal. Example: "root@target:.*# ". This is used to find an empty shell after each command is run. This field is optional and will default to "root@{MACHINE}:.*# " if no other value is given. - TEST_SERIALCONTROL_CONNECT_TIMEOUT (optional) Specifies the timeout in seconds for the initial connection to the target. Defaults to 10 if no other value is given. The serial target does have some additional limitations over the ssh target. 1. Only supports one "run" command at a time. If two threads attempt to call "run", one will block until it finishes. This is a limitation of the serial link, since two connections cannot be opened at once. 2. For file transfer, the target needs a shell and the base32 program. The file transfer implementation was chosen to be as generic as possible, so it could support as many targets as possible. 3. Transferring files is significantly slower. On a 115200 baud serial connection, the fastest observed speed was 30kbps. This is due to overhead in the implementation due to decisions documented in #2 above. (From OE-Core rev: d817b27d73d29ba2beffa2e0a4e31a14dbe0f1bf) Signed-off-by: Andrew Oppelt <andrew.j.oppelt@boeing.com> Signed-off-by: Matthew Weber <matthew.l.weber3@boeing.com> Signed-off-by: Chuck Wolber <chuck.wolber@boeing.com> -- Tested with core-image-sato on real hardware. TEST_SERIALCONTROL_CMD was set to a bash script which connected with telnet to the target. Additionally tested with QEMU by setting TEST_SERIALCONTROL_CMD to "ssh -o StrictHostKeyChecking=no root@192.168.7.2". This imitates a serial connection to the QEMU instance. Steps: 1) Set the following in local.conf: - IMAGE_CLASSES += "testexport" - TEST_TARGET = "serial" - TEST_SERIALCONTROL_CMD="ssh -o StrictHostKeyChecking=no root@192.168.7.2" 2) Build an image - bitbake core-image-sato 3) Run the test export - bitbake -c testexport core-image-sato 4) Run the image in qemu - runqemu nographic core-image-sato 5) Navigate to the test export directory 6) Run the exported tests with target-type set to serial - ./oe-test runtime --test-data-file ./data/testdata.json --packages-manifest ./data/manifest --debug --target-type serial Signed-off-by: Alexandre Belloni <alexandre.belloni@bootlin.com> Signed-off-by: Richard Purdie <richard.purdie@linuxfoundation.org>
228 lines
9.5 KiB
Python
228 lines
9.5 KiB
Python
#
|
|
# Copyright (C) 2016 Intel Corporation
|
|
#
|
|
# SPDX-License-Identifier: MIT
|
|
#
|
|
|
|
import os
|
|
import sys
|
|
|
|
from oeqa.core.context import OETestContext, OETestContextExecutor
|
|
from oeqa.core.target.serial import OESerialTarget
|
|
from oeqa.core.target.ssh import OESSHTarget
|
|
from oeqa.core.target.qemu import OEQemuTarget
|
|
|
|
from oeqa.runtime.loader import OERuntimeTestLoader
|
|
|
|
class OERuntimeTestContext(OETestContext):
|
|
loaderClass = OERuntimeTestLoader
|
|
runtime_files_dir = os.path.join(
|
|
os.path.dirname(os.path.abspath(__file__)), "files")
|
|
|
|
def __init__(self, td, logger, target,
|
|
image_packages, extract_dir):
|
|
super(OERuntimeTestContext, self).__init__(td, logger)
|
|
|
|
self.target = target
|
|
self.image_packages = image_packages
|
|
self.extract_dir = extract_dir
|
|
self._set_target_cmds()
|
|
|
|
def _set_target_cmds(self):
|
|
self.target_cmds = {}
|
|
|
|
self.target_cmds['ps'] = 'ps'
|
|
if 'procps' in self.image_packages:
|
|
self.target_cmds['ps'] = self.target_cmds['ps'] + ' -ef'
|
|
|
|
class OERuntimeTestContextExecutor(OETestContextExecutor):
|
|
_context_class = OERuntimeTestContext
|
|
|
|
name = 'runtime'
|
|
help = 'runtime test component'
|
|
description = 'executes runtime tests over targets'
|
|
|
|
default_cases = os.path.join(os.path.abspath(os.path.dirname(__file__)),
|
|
'cases')
|
|
default_data = None
|
|
default_test_data = 'data/testdata.json'
|
|
default_tests = ''
|
|
default_json_result_dir = '%s-results' % name
|
|
|
|
default_target_type = 'simpleremote'
|
|
default_manifest = 'data/manifest'
|
|
default_server_ip = '192.168.7.1'
|
|
default_target_ip = '192.168.7.2'
|
|
default_extract_dir = 'packages/extracted'
|
|
|
|
def register_commands(self, logger, subparsers):
|
|
super(OERuntimeTestContextExecutor, self).register_commands(logger, subparsers)
|
|
|
|
runtime_group = self.parser.add_argument_group('runtime options')
|
|
|
|
runtime_group.add_argument('--target-type', action='store',
|
|
default=self.default_target_type, choices=['simpleremote', 'qemu', 'serial'],
|
|
help="Target type of device under test, default: %s" \
|
|
% self.default_target_type)
|
|
runtime_group.add_argument('--target-ip', action='store',
|
|
default=self.default_target_ip,
|
|
help="IP address and optionally ssh port (default 22) of device under test, for example '192.168.0.7:22'. Default: %s" \
|
|
% self.default_target_ip)
|
|
runtime_group.add_argument('--server-ip', action='store',
|
|
default=self.default_target_ip,
|
|
help="IP address of the test host from test target machine, default: %s" \
|
|
% self.default_server_ip)
|
|
|
|
runtime_group.add_argument('--host-dumper-dir', action='store',
|
|
help="Directory where host status is dumped, if tests fails")
|
|
|
|
runtime_group.add_argument('--packages-manifest', action='store',
|
|
default=self.default_manifest,
|
|
help="Package manifest of the image under test, default: %s" \
|
|
% self.default_manifest)
|
|
|
|
runtime_group.add_argument('--extract-dir', action='store',
|
|
default=self.default_extract_dir,
|
|
help='Directory where extracted packages reside, default: %s' \
|
|
% self.default_extract_dir)
|
|
|
|
runtime_group.add_argument('--qemu-boot', action='store',
|
|
help="Qemu boot configuration, only needed when target_type is QEMU.")
|
|
|
|
@staticmethod
|
|
def getTarget(target_type, logger, target_ip, server_ip, **kwargs):
|
|
target = None
|
|
|
|
if target_ip:
|
|
target_ip_port = target_ip.split(':')
|
|
if len(target_ip_port) == 2:
|
|
target_ip = target_ip_port[0]
|
|
kwargs['port'] = target_ip_port[1]
|
|
|
|
if server_ip:
|
|
server_ip_port = server_ip.split(':')
|
|
if len(server_ip_port) == 2:
|
|
server_ip = server_ip_port[0]
|
|
kwargs['server_port'] = int(server_ip_port[1])
|
|
|
|
if target_type == 'simpleremote':
|
|
target = OESSHTarget(logger, target_ip, server_ip, **kwargs)
|
|
elif target_type == 'qemu':
|
|
target = OEQemuTarget(logger, server_ip, **kwargs)
|
|
elif target_type == 'serial':
|
|
target = OESerialTarget(logger, target_ip, server_ip, **kwargs)
|
|
else:
|
|
# XXX: This code uses the old naming convention for controllers and
|
|
# targets, the idea it is to leave just targets as the controller
|
|
# most of the time was just a wrapper.
|
|
# XXX: This code tries to import modules from lib/oeqa/controllers
|
|
# directory and treat them as controllers, it will less error prone
|
|
# to use introspection to load such modules.
|
|
# XXX: Don't base your targets on this code it will be refactored
|
|
# in the near future.
|
|
# Custom target module loading
|
|
controller = OERuntimeTestContextExecutor.getControllerModule(target_type)
|
|
target = controller(logger, target_ip, server_ip, **kwargs)
|
|
|
|
return target
|
|
|
|
# Search oeqa.controllers module directory for and return a controller
|
|
# corresponding to the given target name.
|
|
# AttributeError raised if not found.
|
|
# ImportError raised if a provided module can not be imported.
|
|
@staticmethod
|
|
def getControllerModule(target):
|
|
controllerslist = OERuntimeTestContextExecutor._getControllerModulenames()
|
|
controller = OERuntimeTestContextExecutor._loadControllerFromName(target, controllerslist)
|
|
return controller
|
|
|
|
# Return a list of all python modules in lib/oeqa/controllers for each
|
|
# layer in bbpath
|
|
@staticmethod
|
|
def _getControllerModulenames():
|
|
|
|
controllerslist = []
|
|
|
|
def add_controller_list(path):
|
|
if not os.path.exists(os.path.join(path, '__init__.py')):
|
|
raise OSError('Controllers directory %s exists but is missing __init__.py' % path)
|
|
files = sorted([f for f in os.listdir(path) if f.endswith('.py') and not f.startswith('_') and not f.startswith('.#')])
|
|
for f in files:
|
|
module = 'oeqa.controllers.' + f[:-3]
|
|
if module not in controllerslist:
|
|
controllerslist.append(module)
|
|
else:
|
|
raise RuntimeError("Duplicate controller module found for %s. Layers should create unique controller module names" % module)
|
|
|
|
# sys.path can contain duplicate paths, but because of the login in
|
|
# add_controller_list this doesn't work and causes testimage to abort.
|
|
# Remove duplicates using an intermediate dictionary to ensure this
|
|
# doesn't happen.
|
|
for p in list(dict.fromkeys(sys.path)):
|
|
controllerpath = os.path.join(p, 'oeqa', 'controllers')
|
|
if os.path.exists(controllerpath):
|
|
add_controller_list(controllerpath)
|
|
return controllerslist
|
|
|
|
# Search for and return a controller from given target name and
|
|
# set of module names.
|
|
# Raise AttributeError if not found.
|
|
# Raise ImportError if a provided module can not be imported
|
|
@staticmethod
|
|
def _loadControllerFromName(target, modulenames):
|
|
for name in modulenames:
|
|
obj = OERuntimeTestContextExecutor._loadControllerFromModule(target, name)
|
|
if obj:
|
|
return obj
|
|
raise AttributeError("Unable to load {0} from available modules: {1}".format(target, str(modulenames)))
|
|
|
|
# Search for and return a controller or None from given module name
|
|
@staticmethod
|
|
def _loadControllerFromModule(target, modulename):
|
|
try:
|
|
import importlib
|
|
module = importlib.import_module(modulename)
|
|
return getattr(module, target)
|
|
except AttributeError:
|
|
return None
|
|
|
|
@staticmethod
|
|
def readPackagesManifest(manifest):
|
|
if not manifest or not os.path.exists(manifest):
|
|
raise OSError("Manifest file not exists: %s" % manifest)
|
|
|
|
image_packages = set()
|
|
with open(manifest, 'r') as f:
|
|
for line in f.readlines():
|
|
line = line.strip()
|
|
if line and not line.startswith("#"):
|
|
image_packages.add(line.split()[0])
|
|
|
|
return image_packages
|
|
|
|
def _process_args(self, logger, args):
|
|
if not args.packages_manifest:
|
|
raise TypeError('Manifest file not provided')
|
|
|
|
super(OERuntimeTestContextExecutor, self)._process_args(logger, args)
|
|
|
|
td = self.tc_kwargs['init']['td']
|
|
|
|
target_kwargs = {}
|
|
target_kwargs['machine'] = td.get("MACHINE") or None
|
|
target_kwargs['qemuboot'] = args.qemu_boot
|
|
target_kwargs['serialcontrol_cmd'] = td.get("TEST_SERIALCONTROL_CMD") or None
|
|
target_kwargs['serialcontrol_extra_args'] = td.get("TEST_SERIALCONTROL_EXTRA_ARGS") or ""
|
|
target_kwargs['serialcontrol_ps1'] = td.get("TEST_SERIALCONTROL_PS1") or None
|
|
target_kwargs['serialcontrol_connect_timeout'] = td.get("TEST_SERIALCONTROL_CONNECT_TIMEOUT") or None
|
|
|
|
self.tc_kwargs['init']['target'] = \
|
|
OERuntimeTestContextExecutor.getTarget(args.target_type,
|
|
None, args.target_ip, args.server_ip, **target_kwargs)
|
|
self.tc_kwargs['init']['image_packages'] = \
|
|
OERuntimeTestContextExecutor.readPackagesManifest(
|
|
args.packages_manifest)
|
|
self.tc_kwargs['init']['extract_dir'] = args.extract_dir
|
|
|
|
_executor_class = OERuntimeTestContextExecutor
|