mirror of
https://git.yoctoproject.org/poky-contrib
synced 2025-05-08 23:52:25 +08:00
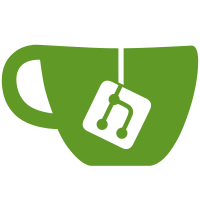
This adds SPDX license headers in place of the wide assortment of things currently in our script headers. We default to GPL-2.0-only except for the oeqa code where it was clearly submitted and marked as MIT on the most part or some scripts which had the "or later" GPL versioning. The patch also drops other obsolete bits of file headers where they were encoountered such as editor modelines, obsolete maintainer information or the phrase "All rights reserved" which is now obsolete and not required in copyright headers (in this case its actually confusing for licensing as all rights were not reserved). More work is needed for OE-Core but this takes care of the bulk of the scripts and meta/lib directories. The top level LICENSE files are tweaked to match the new structure and the SPDX naming. (From OE-Core rev: f8c9c511b5f1b7dbd45b77f345cb6c048ae6763e) Signed-off-by: Richard Purdie <richard.purdie@linuxfoundation.org>
172 lines
4.1 KiB
Bash
Executable File
172 lines
4.1 KiB
Bash
Executable File
#!/bin/bash
|
|
#
|
|
# Copyright (c) 2010-2011, Intel Corporation.
|
|
#
|
|
# SPDX-License-Identifier: GPL-2.0-or-later
|
|
#
|
|
|
|
#
|
|
# This script is intended to be used to send a patch series prepared by the
|
|
# create-pull-request script to Open Embedded and The Yocto Project, as well
|
|
# as to related projects and layers.
|
|
#
|
|
|
|
AUTO=0
|
|
AUTO_CL=0
|
|
GITSOBCC="--suppress-cc=all"
|
|
|
|
# Prevent environment leakage to these vars.
|
|
unset TO
|
|
unset CC
|
|
unset AUTO_CC
|
|
unset EXTRA_CC
|
|
|
|
usage()
|
|
{
|
|
cat <<EOM
|
|
Usage: $(basename $0) [-h] [-a] [-c] [[-t email]...] -p pull-dir
|
|
-a Send the cover letter to every recipient listed in Cc and
|
|
Signed-off-by lines found in the cover letter and the patches.
|
|
This option implies -c.
|
|
-c Expand the Cc list for the individual patches using the Cc and
|
|
Signed-off-by lines from the same patch.
|
|
-C Add extra CC to each email sent.
|
|
-p pull-dir Directory containing summary and patch files
|
|
-t email Explicitly add email to the recipients
|
|
EOM
|
|
}
|
|
|
|
# Collect addresses from a patch into AUTO_CC
|
|
# $1: a patch file
|
|
harvest_recipients()
|
|
{
|
|
PATCH=$1
|
|
export IFS=$',\n'
|
|
for REGX in "^[Cc][Cc]: *" "^[Ss]igned-[Oo]ff-[Bb]y: *"; do
|
|
for EMAIL in $(sed '/^---$/q' $PATCH | grep -e "$REGX" | sed "s/$REGX//"); do
|
|
if [ "${AUTO_CC/$EMAIL/}" == "$AUTO_CC" ] && [ -n "$EMAIL" ]; then
|
|
if [ -z "$AUTO_CC" ]; then
|
|
AUTO_CC=$EMAIL;
|
|
else
|
|
AUTO_CC="$AUTO_CC,$EMAIL";
|
|
fi
|
|
fi
|
|
done
|
|
done
|
|
unset IFS
|
|
}
|
|
|
|
# Parse and verify arguments
|
|
while getopts "acC:hp:t:" OPT; do
|
|
case $OPT in
|
|
a)
|
|
AUTO=1
|
|
GITSOBCC="--signed-off-by-cc"
|
|
AUTO_CL=1
|
|
;;
|
|
c)
|
|
AUTO=1
|
|
GITSOBCC="--signed-off-by-cc"
|
|
;;
|
|
C)
|
|
EXTRA_CC="$OPTARG"
|
|
;;
|
|
h)
|
|
usage
|
|
exit 0
|
|
;;
|
|
p)
|
|
PDIR=${OPTARG%/}
|
|
if [ ! -d $PDIR ]; then
|
|
echo "ERROR: pull-dir \"$PDIR\" does not exist."
|
|
usage
|
|
exit 1
|
|
fi
|
|
;;
|
|
t)
|
|
if [ -n "$TO" ]; then
|
|
TO="$TO,$OPTARG"
|
|
else
|
|
TO="$OPTARG"
|
|
fi
|
|
;;
|
|
esac
|
|
done
|
|
|
|
if [ -z "$PDIR" ]; then
|
|
echo "ERROR: you must specify a pull-dir."
|
|
usage
|
|
exit 1
|
|
fi
|
|
|
|
|
|
# Verify the cover letter is complete and free of tokens
|
|
if [ -e $PDIR/0000-cover-letter.patch ]; then
|
|
CL="$PDIR/0000-cover-letter.patch"
|
|
for TOKEN in SUBJECT BLURB; do
|
|
grep -q "*** $TOKEN HERE ***" "$CL"
|
|
if [ $? -eq 0 ]; then
|
|
echo "ERROR: Please edit $CL and try again (Look for '*** $TOKEN HERE ***')."
|
|
exit 1
|
|
fi
|
|
done
|
|
else
|
|
echo "WARNING: No cover letter will be sent."
|
|
fi
|
|
|
|
# Harvest emails from the generated patches and populate AUTO_CC.
|
|
if [ $AUTO_CL -eq 1 ]; then
|
|
for PATCH in $PDIR/*.patch; do
|
|
harvest_recipients $PATCH
|
|
done
|
|
fi
|
|
|
|
AUTO_TO="$(git config sendemail.to)"
|
|
if [ -n "$AUTO_TO" ]; then
|
|
if [ -n "$TO" ]; then
|
|
TO="$TO,$AUTO_TO"
|
|
else
|
|
TO="$AUTO_TO"
|
|
fi
|
|
fi
|
|
|
|
if [ -z "$TO" ] && [ -z "$AUTO_CC" ]; then
|
|
echo "ERROR: you have not specified any recipients."
|
|
usage
|
|
exit 1
|
|
fi
|
|
|
|
|
|
# Convert the collected addresses into git-send-email argument strings
|
|
export IFS=$','
|
|
GIT_TO=$(for R in $TO; do echo -n "--to='$R' "; done)
|
|
GIT_CC=$(for R in $AUTO_CC; do echo -n "--cc='$R' "; done)
|
|
GIT_EXTRA_CC=$(for R in $EXTRA_CC; do echo -n "--cc='$R' "; done)
|
|
unset IFS
|
|
|
|
# Handoff to git-send-email. It will perform the send confirmation.
|
|
# Mail threading was already handled by git-format-patch in
|
|
# create-pull-request, so we must not allow git-send-email to
|
|
# add In-Reply-To and References headers again.
|
|
PATCHES=$(echo $PDIR/*.patch)
|
|
if [ $AUTO_CL -eq 1 ]; then
|
|
# Send the cover letter to every recipient, both specified as well as
|
|
# harvested. Then remove it from the patches list.
|
|
# --no-thread is redundant here (only sending a single message) and
|
|
# merely added for the sake of consistency.
|
|
eval "git send-email $GIT_TO $GIT_CC $GIT_EXTRA_CC --confirm=always --no-thread --suppress-cc=all $CL"
|
|
if [ $? -eq 1 ]; then
|
|
echo "ERROR: failed to send cover-letter with automatic recipients."
|
|
exit 1
|
|
fi
|
|
PATCHES=${PATCHES/"$CL"/}
|
|
fi
|
|
|
|
# Send the patch to the specified recipients and, if -c was specified, those git
|
|
# finds in this specific patch.
|
|
eval "git send-email $GIT_TO $GIT_EXTRA_CC --confirm=always --no-thread $GITSOBCC $PATCHES"
|
|
if [ $? -eq 1 ]; then
|
|
echo "ERROR: failed to send patches."
|
|
exit 1
|
|
fi
|